这篇文章主要介绍了c#如何使用 XML 文档功能,帮助大家更好的理解和使用c#,感兴趣的朋友可以了解下
下面的示例提供对某个已存档类型的基本概述。
示例
// If compiling from the command line, compile with: -doc:YourFileName.xml
/// <summary>
/// Class level summary documentation goes here.
/// </summary>
/// <remarks>
/// Longer comments can be associated with a type or member through
/// the remarks tag.
/// </remarks>
public class TestClass : TestInterface
{
/// <summary>
/// Store for the Name property.
/// </summary>
private string _name = null;
/// <summary>
/// The class constructor.
/// </summary>
public TestClass()
{
// TODO: Add Constructor Logic here.
}
/// <summary>
/// Name property.
/// </summary>
/// <value>
/// A value tag is used to describe the property value.
/// </value>
public string Name
{
get
{
if (_name == null)
{
throw new System.Exception("Name is null");
}
return _name;
}
}
/// <summary>
/// Description for SomeMethod.
/// </summary>
/// <param name="s"> Parameter description for s goes here.</param>
/// <seealso cref="System.String">
/// You can use the cref attribute on any tag to reference a type or member
/// and the compiler will check that the reference exists.
/// </seealso>
public void SomeMethod(string s)
{
}
/// <summary>
/// Some other method.
/// </summary>
/// <returns>
/// Return values are described through the returns tag.
/// </returns>
/// <seealso cref="SomeMethod(string)">
/// Notice the use of the cref attribute to reference a specific method.
/// </seealso>
public int SomeOtherMethod()
{
return 0;
}
public int InterfaceMethod(int n)
{
return n * n;
}
/// <summary>
/// The entry point for the application.
/// </summary>
/// <param name="args"> A list of command line arguments.</param>
static int Main(System.String[] args)
{
// TODO: Add code to start application here.
return 0;
}
}
/// <summary>
/// Documentation that describes the interface goes here.
/// </summary>
/// <remarks>
/// Details about the interface go here.
/// </remarks>
interface TestInterface
{
/// <summary>
/// Documentation that describes the method goes here.
/// </summary>
/// <param name="n">
/// Parameter n requires an integer argument.
/// </param>
/// <returns>
/// The method returns an integer.
/// </returns>
int InterfaceMethod(int n);
}
该示例生成一个包含以下内容的 .xml 文件。
<?xml version="1.0"?>
<doc>
<assembly>
<name>xmlsample</name>
</assembly>
<members>
<member name="T:TestClass">
<summary>
Class level summary documentation goes here.
</summary>
<remarks>
Longer comments can be associated with a type or member through
the remarks tag.
</remarks>
</member>
<member name="F:TestClass._name">
<summary>
Store for the Name property.
</summary>
</member>
<member name="M:TestClass.#ctor">
<summary>
The class constructor.
</summary>
</member>
<member name="P:TestClass.Name">
<summary>
Name property.
</summary>
<value>
A value tag is used to describe the property value.
</value>
</member>
<member name="M:TestClass.SomeMethod(System.String)">
<summary>
Description for SomeMethod.
</summary>
<param name="s"> Parameter description for s goes here.</param>
<seealso cref="T:System.String">
You can use the cref attribute on any tag to reference a type or member
and the compiler will check that the reference exists.
</seealso>
</member>
<member name="M:TestClass.SomeOtherMethod">
<summary>
Some other method.
</summary>
<returns>
Return values are described through the returns tag.
</returns>
<seealso cref="M:TestClass.SomeMethod(System.String)">
Notice the use of the cref attribute to reference a specific method.
</seealso>
</member>
<member name="M:TestClass.Main(System.String[])">
<summary>
The entry point for the application.
</summary>
<param name="args"> A list of command line arguments.</param>
</member>
<member name="T:TestInterface">
<summary>
Documentation that describes the interface goes here.
</summary>
<remarks>
Details about the interface go here.
</remarks>
</member>
<member name="M:TestInterface.InterfaceMethod(System.Int32)">
<summary>
Documentation that describes the method goes here.
</summary>
<param name="n">
Parameter n requires an integer argument.
</param>
<returns>
The method returns an integer.
</returns>
</member>
</members>
</doc>
编译代码
若要编译该示例,请输入以下命令:
csc XMLsample.cs /doc:XMLsample.xml
此命令创建 XML 文件 XMLsample.xml,可在浏览器中或使用 TYPE 命令查看该文件。
可靠编程
XML 文档以 /// 开头。 创建新项目时,向导会放置一些以 /// 开头的行。 处理这些注释时存在一些限制:
1.文档必须是格式正确的 XML。 如果 XML 格式不正确,则会生成警告,并且文档文件将包含一条注释,指出遇到错误。
2.开发人员可以随意创建自己的标记集。 有一组推荐的标记。 部分建议标记具有特殊含义:
- <param> 标记用于描述参数。 如果已使用,编译器会验证该参数是否存在,以及文档是否描述了所有参数。 如果验证失败,编译器会发出警告。
- cref 属性可以附加到任何标记,以引用代码元素。 编译器验证此代码元素是否存在。 如果验证失败,编译器会发出警告。 编译器在查找 cref 属性中描述的类型时会考虑所有 using 语句。
- <summary> 标记由 Visual Studio 中的 IntelliSense 用于显示有关某个类型或成员的附加信息。
备注
XML 文件不提供有关该类型和成员的完整信息(例如,它不包含任何类型信息)。 若要获取有关类型或成员的完整信息,请将文档文件与对实际类型或成员的反射一起使用。
以上就是c#如何使用 XML 文档功能的详细内容,更多关于c# 使用 XML 文档功能的资料请关注得得之家其它相关文章!
本文标题为:c#如何使用 XML 文档功能
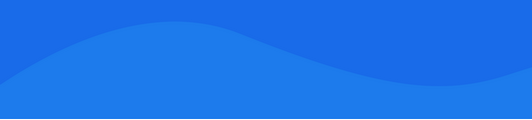
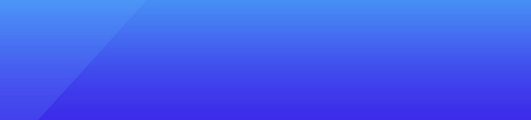
基础教程推荐
- ZooKeeper的安装及部署教程 2023-01-22
- linux – 如何在Debian Jessie中安装dotnet core sdk 2023-09-26
- 一个读写csv文件的C#类 2022-11-06
- unity实现动态排行榜 2023-04-27
- C# 调用WebService的方法 2023-03-09
- C#类和结构详解 2023-05-30
- C# List实现行转列的通用方案 2022-11-02
- winform把Office转成PDF文件 2023-06-14
- C# windows语音识别与朗读实例 2023-04-27
- C#控制台实现飞行棋小游戏 2023-04-22