这篇文章主要介绍了使用C#实现Windows组和用户管理的示例代码,帮助大家更好的理解和使用c#,感兴趣的朋友可以了解下
1、WindowsAccountHelper类实现
using System;
using System.Collections.Generic;
using System.DirectoryServices.AccountManagement;
using System.Linq;
public class WindowsAccountHelper
{
public static string LastErrorMsg { get; private set; }
public static List<string> GetGroups()
{
var groups = new List<string>();
try
{
var context = new PrincipalContext(ContextType.Machine);
var queryGroup = new GroupPrincipal(context);
var searcher = new PrincipalSearcher(queryGroup);
searcher.FindAll().ToList().ForEach(t => groups.Add(t.Name));
}
catch (Exception)
{
groups.Clear();
}
return groups;
}
public static List<string> GetGroupUsers(string groupName)
{
var group = GetGroup(groupName);
return GetGroupUsers(group);
}
public static List<string> GetGroupUsers(GroupPrincipal group)
{
var users = new List<string>();
if (group == null)
{
return users;
}
group.GetMembers().ToList().ForEach(t => users.Add(t.Name));
return users;
}
public static GroupPrincipal GetGroup(string groupName)
{
GroupPrincipal group = null;
try
{
var context = new PrincipalContext(ContextType.Machine);
var queryGroup = new GroupPrincipal(context);
var searcher = new PrincipalSearcher(queryGroup);
foreach (var principal in searcher.FindAll())
{
var groupPrincipal = (GroupPrincipal)principal;
if (groupPrincipal != null && groupPrincipal.Name.Equals(groupName))
{
group = groupPrincipal;
break;
}
}
}
catch (Exception)
{
// ignored
}
return group;
}
public static GroupPrincipal CreateGroup(string groupName, string description, bool isSecurityGroup)
{
GroupPrincipal group;
try
{
group = GetGroup(groupName);
if (group == null)
{
var context = new PrincipalContext(ContextType.Machine);
group = new GroupPrincipal(context)
{
Name = groupName,
Description = description,
IsSecurityGroup = isSecurityGroup,
GroupScope = GroupScope.Local
};
group.Save();
}
}
catch (Exception e)
{
LastErrorMsg = e.Message;
group = null;
}
return group;
}
public static bool DeleteGroup(string groupName)
{
var group = GetGroup(groupName);
if (group == null)
{
return true;
}
var ret = true;
try
{
group.Delete();
}
catch (Exception)
{
ret = false;
}
return ret;
}
public static bool CreateWindowsAccount(string userName, string password,
string displayName, string description, bool cannotChangePassword,
bool passwordNeverExpires, string groupName)
{
bool ret;
try
{
var context = new PrincipalContext(ContextType.Machine);
var group = GroupPrincipal.FindByIdentity(context, groupName);
if (group == null)
{
return false;
}
ret = CreateWindowsAccount(userName, password, displayName,
description, cannotChangePassword, passwordNeverExpires, group);
}
catch (Exception)
{
ret = false;
}
return ret;
}
public static bool CreateWindowsAccount(string userName, string password,
string displayName, string description, bool cannotChangePassword,
bool passwordNeverExpires, GroupPrincipal group)
{
bool ret;
try
{
if (group == null)
{
return false;
}
var context = new PrincipalContext(ContextType.Machine);
var user = UserPrincipal.FindByIdentity(context, userName)
?? new UserPrincipal(context);
user.SetPassword(password);
user.DisplayName = displayName;
user.Name = userName;
user.Description = description;
user.UserCannotChangePassword = cannotChangePassword;
user.PasswordNeverExpires = passwordNeverExpires;
user.Save();
group.Members.Add(user);
group.Save();
ret = true;
}
catch (Exception)
{
ret = false;
}
return ret;
}
public static bool DeleteWindowsAccount(List<string> userNameList)
{
var ret = true;
try
{
foreach (var userName in userNameList)
{
var context = new PrincipalContext(ContextType.Machine);
var user = UserPrincipal.FindByIdentity(context, userName);
user?.Delete();
}
}
catch (Exception)
{
ret = false;
}
return ret;
}
public static bool ChangeUserGroup(string userName, string groupName)
{
bool ret;
try
{
var context = new PrincipalContext(ContextType.Machine);
var group = GroupPrincipal.FindByIdentity(context, groupName);
if (group == null)
{
return false;
}
ret = ChangeUserGroup(userName, group);
}
catch (Exception)
{
ret = false;
}
return ret;
}
public static bool ChangeUserGroup(string userName, GroupPrincipal group)
{
bool ret;
try
{
if (group == null)
{
return false;
}
var context = new PrincipalContext(ContextType.Machine);
var user = UserPrincipal.FindByIdentity(context, userName);
if (user == null)
{
return false;
}
if (!group.Members.Contains(user))
{
group.Members.Add(user);
group.Save();
}
ret = true;
}
catch (Exception)
{
ret = false;
}
return ret;
}
public static int UpdateGroupUsers(string groupName, List<string> userNames, string password = "")
{
var group = CreateGroup(groupName, string.Empty, false);
if (group == null)
{
return 0;
}
var userNameList = new List<string>();
userNameList.AddRange(userNames);
var addedUsers = new List<string>();
int groupUserCount;
try
{
foreach (var principal in group.GetMembers())
{
var user = (UserPrincipal)principal;
if (user == null)
{
continue;
}
if (userNameList.Contains(user.Name))
{
//已有用户
addedUsers.Add(user.Name);
}
else
{
user.Delete();
}
}
//已有用户数
groupUserCount = addedUsers.Count;
//剩余的即为需要添加的用户集合
foreach (var userName in addedUsers)
{
userNameList.Remove(userName);
}
//创建用户
foreach (var userName in userNameList)
{
if (CreateWindowsAccount(userName, password,
userName, string.Empty,
false, false, group))
{
groupUserCount++;
}
}
}
catch (UnauthorizedAccessException)
{
groupUserCount = 0;
}
return groupUserCount;
}
}
2、使用示例
private bool CreateGroupUsers(string groupName, List<string> windowsUserList,
string password, int userCount)
{
var group = WindowsAccountHelper.CreateGroup(groupName, string.Empty, true);
if (group == null)
{
return false;
}
var userNames = WindowsAccountHelper.GetGroupUsers(group);
foreach (var userName in WindowsUserList)
{
if (!userNames.Contains(userName))
{
if (!WindowsAccountHelper.CreateWindowsAccount(userName, password,
userName, string.Empty,
false, false, group))
{
return false;
}
}
}
return true;
}
以上就是使用C#实现Windows组和用户管理的示例代码的详细内容,更多关于C#实现Windows组和用户管理的资料请关注得得之家其它相关文章!
沃梦达教程
本文标题为:使用C#实现Windows组和用户管理的示例代码
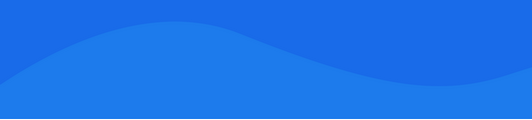
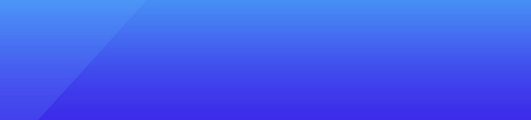
基础教程推荐
猜你喜欢
- C# 调用WebService的方法 2023-03-09
- C# windows语音识别与朗读实例 2023-04-27
- unity实现动态排行榜 2023-04-27
- C# List实现行转列的通用方案 2022-11-02
- C#类和结构详解 2023-05-30
- C#控制台实现飞行棋小游戏 2023-04-22
- winform把Office转成PDF文件 2023-06-14
- linux – 如何在Debian Jessie中安装dotnet core sdk 2023-09-26
- 一个读写csv文件的C#类 2022-11-06
- ZooKeeper的安装及部署教程 2023-01-22