这篇文章主要为大家详细介绍了C#实现简单的五子棋游戏,文中示例代码介绍的非常详细,具有一定的参考价值,感兴趣的小伙伴们可以参考一下
最近利用业余时间写了一个简单的五子棋游戏,没有利用深层次的面向对象技术,自学一年,代码和程序设计有不妥之处,还望大神指出,先看下实现的功能,三个button按钮,黑棋和白棋选择先出,和重置。
其他的不多说了,直接上全部代码(通过测试)。计算输赢的时候,左斜和右斜用了数学y=kx+b的线性函数计算。
private Image myImage;
/// <summary>
/// 初始化背景数组
/// int[x,y] x为行 y为列
/// </summary>
private int[,] bgGround = new int[11, 11]; /*{
{0,0,0,0,0,0,0,0,0,0,0},
{0,0,0,0,0,0,0,0,0,0,0},
{0,0,0,0,0,0,0,0,0,0,0},
{0,0,0,0,0,0,0,0,0,0,0},
{0,0,0,0,0,0,0,0,0,0,0},
{0,0,0,0,0,0,0,0,0,0,0},
{0,0,0,0,0,0,0,0,0,0,0},
{0,0,0,0,0,0,0,0,0,0,0},
{0,0,0,0,0,0,0,0,0,0,0},
{0,0,0,0,0,0,0,0,0,0,0},
{0,0,0,0,0,0,0,0,0,0,0}
};*/
private int CurrentX;//当前bgGround的x行
private int CurrentY;//当前bgGround的y列
private bool IsWhite = false;//判断白棋还是黑棋先
private bool IsOver = false;//记录游戏是否结束
private void Form1_Load(object sender, EventArgs e)
{
myImage = new Bitmap(panel1.Width, panel1.Height);
}
protected override void OnPaint(PaintEventArgs e)
{
Draw();
base.OnPaint(e);
}
/// <summary>
/// 画棋盘
/// </summary>
private void Draw()
{
Graphics g = Graphics.FromImage(myImage);
g.Clear(this.BackColor);
g.FillRectangle(Brushes.White,new Rectangle(new Point(10,10),new Size(400,400)));
//循环次数应比背景bgGround行、列少1
for (int i = 0; i < 10; i++)
{
for (int j = 0; j < 10; j++)
{
g.DrawRectangle(new Pen(Brushes.Black), i * 40 + 10, j * 40 + 10, 40, 40);
}
}
Graphics gg = panel1.CreateGraphics();
gg.DrawImage(myImage, 0, 0);
}
private void panel1_MouseClick(object sender, MouseEventArgs e)
{
if (IsOver)
{
return;
}
Graphics g = panel1.CreateGraphics();
//设置当鼠标点击坐标在某一落棋点坐标想x,y的+-10的范围内即可落子
int x = (e.X - 10) % 40;
int y = (e.Y - 10) % 40;
if (x > 30)
{
x = (e.X - 10) / 40 + 1;
}
else
{
x = (e.X - 10) / 40;
}
if (y > 30)
{
y = (e.Y - 10) / 40 + 1;
}
else
{
y = (e.Y - 10) / 40;
}
if (bgGround[x, y] == 0)
{
if (IsWhite)
{
DrawChess(g, x, y, new Pen(Brushes.White), Brushes.White, 1);
IsWhite = false;
JudgeResult(1);
}
else
{
DrawChess(g, x, y, new Pen(Brushes.Black), Brushes.Black, 2);
IsWhite = true;
JudgeResult(2);
}
}
}
/// <summary>
/// 判断输赢
/// </summary>
/// <param name="flag">1为白棋 2为黑棋</param>
private void JudgeResult(int flag)
{
int x = CurrentX;
int y = CurrentY;
int MinXNum = 0;
int MaxXNum = 0;
int count = 0;
if (x > 4)
{
MinXNum = x - 4;
if (x + 4 > 10)
{
MaxXNum = 10;
}
else
{
MaxXNum = x + 4;
}
}
else
{
MaxXNum = x + 4;
}
int MinYNum = 0;
int MaxYNum = 0;
if (y > 4)
{
MinYNum = y - 4;
if (y + 4 > 10)
{
MaxYNum = 10;
}
else
{
MaxYNum = y + 4;
}
}
else
{
MaxYNum = y + 4;
}
#region //横向
for (int i = MinXNum; i < MaxXNum+1; i++)
{
if (bgGround[i, y] == flag)
{
count++;
if (count > 4)
goto Label;
}
else
{
count = 0;
if (i > MaxXNum - 4)
break;
}
}
#endregion
#region //竖向
for (int i = MinYNum; i < MaxYNum+1; i++)
{
if (bgGround[x, i] == flag)
count++;
else
{
count = 0;
if (i > MaxYNum - 4)
break;
}
if (count > 4)
goto Label;
}
#endregion
//左斜
for (int i = MinXNum; i < MaxXNum+1; i++)
{
if (CurrentX + CurrentY - i < 0)
break;
if (CurrentX + CurrentY - i <= 10)
{
if (bgGround[i, CurrentX + CurrentY - i] == flag)
{
count++;
}
else
{
count = 0;
if (i > MaxYNum - 4)
break;
}
}
if (count > 4)
goto Label;
}
//右斜
for (int i = MinXNum; i < MaxXNum+1; i++)
{
if (i < CurrentX - CurrentY)
break;
if (i + CurrentY - CurrentX > 10)
break;
if (bgGround[i, i + CurrentY - CurrentX] == flag)
{
count++;
}
else
{
count = 0;
if (i > MaxYNum - 4)
break;
}
if (count > 4)
goto Label;
}
Label:
if (flag == 1 && count > 4)
{
IsOver = true;
MessageBox.Show("白棋赢,游戏结束");
return;
}
else if (flag == 2 && count > 4)
{
IsOver = true;
MessageBox.Show("黑棋赢,游戏结束");
return;
}
else
{
IsOver = false;
}
}
/// <summary>
/// 画棋子
/// </summary>
/// <param name="g"></param>
/// <param name="x">bgGround中x位置</param>
/// <param name="y">bgGround中y位置</param>
/// <param name="p">画笔</param>
/// <param name="brush">棋子颜色</param>
/// <param name="flag">1为白棋 2为黑棋</param>
private void DrawChess(Graphics g, int x, int y, Pen p, Brush brush, int flag)
{
CurrentX = x; CurrentY = y;
bgGround[x, y] = flag;
g.DrawEllipse(p, x * 40, y * 40, 20, 20);
g.FillEllipse(brush, x * 40, y * 40, 20, 20);
}
///btn_Chess_Click是黑棋先和白棋先按钮的共同事件,设置白棋先button的tag值为1,黑棋先button的tag值为2
/// <summary>
/// 判断哪个先下 设置button控件的tag值
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void btn_Chess_Click(object sender, EventArgs e)
{
Button btn = sender as Button;
string tag = btn.Tag.ToString();
if (tag.Equals("1"))//白棋先
{
IsWhite = true;
}
else//黑棋先 tag=2
{
IsWhite = false;
}
}
/// <summary>
/// 重置
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void btn_reset_Click(object sender, EventArgs e)
{
IsOver = false;
Draw();
bgGround = new int[11, 11];
}
以上就是本文的全部内容,希望对大家的学习有所帮助,也希望大家多多支持得得之家。
沃梦达教程
本文标题为:C#实现简单的五子棋游戏
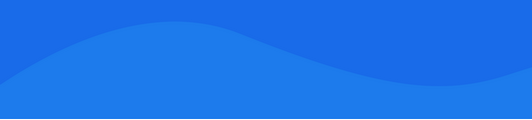
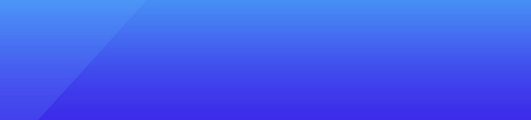
基础教程推荐
猜你喜欢
- 一个读写csv文件的C#类 2022-11-06
- linux – 如何在Debian Jessie中安装dotnet core sdk 2023-09-26
- C# windows语音识别与朗读实例 2023-04-27
- C#类和结构详解 2023-05-30
- unity实现动态排行榜 2023-04-27
- C# List实现行转列的通用方案 2022-11-02
- C#控制台实现飞行棋小游戏 2023-04-22
- winform把Office转成PDF文件 2023-06-14
- C# 调用WebService的方法 2023-03-09
- ZooKeeper的安装及部署教程 2023-01-22