感谢你对“详解Electron中如何使用SQLite存储笔记”的攻略感兴趣。我将分享以下步骤:
感谢你对“详解Electron中如何使用SQLite存储笔记”的攻略感兴趣。我将分享以下步骤:
1. 安装SQLite
在Electron中使用SQLite存储笔记,首先需要安装SQLite。可以通过以下命令行进行安装:
npm install sqlite3 --save
2. 创建数据库连接
在Electron中打开SQLite数据库,需要引入SQLite模块并创建数据库连接。可以使用以下代码:
const sqlite3 = require('sqlite3').verbose();
const db = new sqlite3.Database('./path/to/database.db', (err) => {
if (err) {
console.error(err.message);
}
console.log('Connected to the database.');
});
该代码会创建一个名为database.db
的SQLite数据库,并连接到该数据库。
3. 创建表
在SQLite数据库中创建表,以存储笔记。可以使用以下代码:
db.run(`CREATE TABLE notes (
id INTEGER PRIMARY KEY AUTOINCREMENT,
title TEXT,
content TEXT
);`, (err) => {
if (err) {
console.error(err.message);
}
console.log('Table created.');
});
该代码会创建一个名为notes
的表,其中包含id、title和content三个字段。
4. 插入数据
在SQLite数据库中插入数据,以存储笔记信息。可以使用以下代码:
db.run(`INSERT INTO notes (title, content) VALUES (?, ?)`, ['Note 1', 'This is the content of note 1.'], (err) => {
if (err) {
console.error(err.message);
}
console.log('Data inserted.');
});
该代码会将一条笔记信息插入到notes
表中,其中包含一个标题为Note 1
,内容为This is the content of note 1.
的笔记。
5. 查询数据
在SQLite数据库中查询数据,以获取笔记信息。可以使用以下代码:
db.all(`SELECT * FROM notes`, [], (err, rows) => {
if (err) {
console.error(err.message);
}
rows.forEach(row => {
console.log(row.title, row.content);
});
});
该代码会查询notes
表中的所有数据,并将每条笔记信息输出到控制台。
示例1
以下代码演示了在Electron中创建SQLite数据库,创建notes
表并插入一条笔记信息。
const sqlite3 = require('sqlite3').verbose();
const db = new sqlite3.Database('./path/to/database.db', (err) => {
if (err) {
console.error(err.message);
}
console.log('Connected to the database.');
});
db.run(`CREATE TABLE notes (
id INTEGER PRIMARY KEY AUTOINCREMENT,
title TEXT,
content TEXT
);`, (err) => {
if (err) {
console.error(err.message);
}
console.log('Table created.');
});
db.run(`INSERT INTO notes (title, content) VALUES (?, ?)`, ['Note 1', 'This is the content of note 1.'], (err) => {
if (err) {
console.error(err.message);
}
console.log('Data inserted.');
});
db.close();
示例2
以下代码演示了在Electron中查询SQLite数据库中的笔记信息,并将结果输出到控制台。
const sqlite3 = require('sqlite3').verbose();
const db = new sqlite3.Database('./path/to/database.db', (err) => {
if (err) {
console.error(err.message);
}
console.log('Connected to the database.');
});
db.all(`SELECT * FROM notes`, [], (err, rows) => {
if (err) {
console.error(err.message);
}
rows.forEach(row => {
console.log(row.title, row.content);
});
});
db.close();
以上就是使用Electron的方式,使用SQLite存储笔记的完整攻略,希望能帮到你。
本文标题为:详解Electron中如何使用SQLite存储笔记
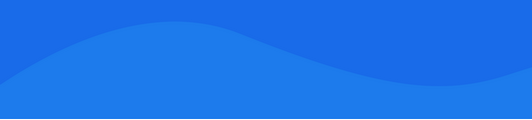
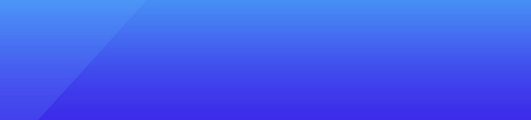
基础教程推荐
- Oracle 19c创建数据库的完整步骤(详细明了) 2023-07-24
- MySQL介绍 2023-10-08
- 记一次mariadb数据库无法连接 2023-07-24
- MySQL常见优化方案汇总 2023-12-06
- 详解MySQL存储过程的创建和调用 2023-08-12
- 在执行gem install redis时 : ERROR: Error installing redis: redis requires Ruby version >= 2.2.2 2023-09-11
- mysql数据库中替换所有表中的所有字段值(存储过程) 2022-07-18
- 详解如何清理Redis内存碎片 2023-07-13
- oracle中关于case when then的使用 2023-07-23
- MongoDB排序时内存大小限制与创建索引的注意事项详解 2023-07-16