这篇文章主要为大家详细介绍了用Java实现聊天程序,文中示例代码介绍的非常详细,具有一定的参考价值,感兴趣的小伙伴们可以参考一下
利用Java编写聊天程序,供大家参考,具体内容如下
首先计算机网络有两种传输层协议:TCP(面向连接),UDP(面向无连接)。今天就介绍基于这两种协议的聊天程序。
先查明自己电脑的主机名
右键我的电脑-->属性
一、基于UDP的聊天程序
1.基于UDP的发送端
package cn.com;
/**
* 基于UDP
* 聊天发送端
*/
import java.io.IOException;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
import java.net.InetAddress;
import java.util.Scanner;
public class Send {
public static void main(String[] args) throws IOException {
@SuppressWarnings("resource")
DatagramSocket ds = new DatagramSocket();
Scanner sc = new Scanner(System.in);
String line = null;
while ((line = sc.nextLine()) != null) {
byte[] buf = line.getBytes();
int length = buf.length;
InetAddress address = InetAddress.getByName("1-PC21");//主机名
DatagramPacket dp = new DatagramPacket(buf, length, address, 10086); //10086为自己设置的端口号
ds.send(dp);
}
sc.close();
}
}
2.基于UDP的接收端
package cn.com;
/**
* 基于UDP
* 聊天接收端
*/
import java.io.IOException;
import java.net.DatagramPacket;
import java.net.DatagramSocket;
public class Receive {
public static void main(String[] args) throws IOException {
@SuppressWarnings("resource")
DatagramSocket ds = new DatagramSocket(10086);//端口号需一致
while (true) {
byte[] b = new byte[1024 * 1];
int length = b.length;
DatagramPacket dp = new DatagramPacket(b, length);
ds.receive(dp);
byte[] data = dp.getData();
int length2 = dp.getLength();
String hostAddress = dp.getAddress().getHostAddress();
String s = new String(data, 0, length2);
System.out.println(s + "来自" + hostAddress);
}
}
}
3.先运行接收端,在运行发送端
发送端发送“Hello World”,“My name is Tom”。
接收端收到信息
二、基于TCP的聊天程序
1.客户端
package cn.com;
/**
* 基于TCP
* 聊天系统客户端
*/
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.InetAddress;
import java.net.Socket;
import java.util.Scanner;
public class Client {
public static void main(String[] args) throws IOException {
InetAddress address = InetAddress.getByName("1-PC21");//主机名
int port = 10089;
Scanner sc = new Scanner(System.in);
String line = null;
while ((line = sc.nextLine()) != null) {
@SuppressWarnings("resource")
Socket socket = new Socket(address, port); //socket要在循环体中定义
OutputStream os = socket.getOutputStream();
os.write(line.getBytes());
// 客户端接收服务端返回的消息(输入流)
InputStream is = socket.getInputStream();
byte[] b = new byte[1024 * 1];
int len = is.read(b);
String s = new String(b, 0, len);
System.out.println(s);
}
sc.close();
}
}
2.服务端
package cn.com;
/**
* 基于TCP
* 聊天系统服务端
*/
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.ServerSocket;
import java.net.Socket;
public class Server {
public static void main(String[] args) throws IOException {
@SuppressWarnings("resource")
ServerSocket ss = new ServerSocket(10089);
while (true) {
Socket accept = ss.accept(); //循环中用到accept,所以要在循环中新建定义
InputStream is = accept.getInputStream();
byte[] b = new byte[1024 * 1];
int len = is.read(b);
String s = new String(b, 0, len);
System.out.println("已接收客户端内容-->" + s);
// 给客户端返回数据
OutputStream os = accept.getOutputStream();
String content = "客户端接收成功";
os.write(content.getBytes());
os.close();
is.close();
accept.close();
}
}
}
3.还是先打开服务端,再打开客户端,发送信息
客户端发送:“今天星期四”,“天气很好” 两条信息。
服务端收到信息:
以上就是本文的全部内容,希望对大家的学习有所帮助,也希望大家多多支持编程学习网。
沃梦达教程
本文标题为:用Java实现聊天程序
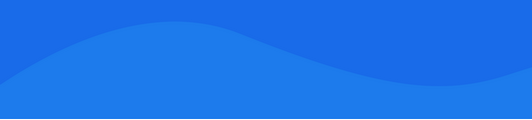
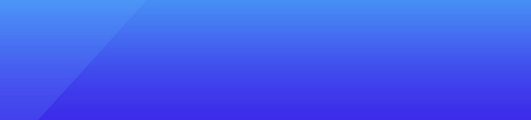
基础教程推荐
猜你喜欢
- Java文件管理操作的知识点整理 2023-05-19
- ConditionalOnProperty配置swagger不生效问题及解决 2023-01-02
- Java数据结构之对象比较详解 2023-03-07
- java基础知识之FileInputStream流的使用 2023-08-11
- Java实现查找文件和替换文件内容 2023-04-06
- Java实现线程插队的示例代码 2022-09-03
- Java并发编程进阶之线程控制篇 2023-03-07
- JDK数组阻塞队列源码深入分析总结 2023-04-18
- java实现多人聊天系统 2023-05-19
- springboot自定义starter方法及注解实例 2023-03-31