我来给您讲解一下“JSP文件上传与下载实例代码”的完整攻略。
我来给您讲解一下“JSP文件上传与下载实例代码”的完整攻略。
步骤一:添加文件上传功能
首先,在JSP页面上添加文件上传功能,可以使用HTML中的<form>
表单和<input>
标签实现。上传文件时,需要使用enctype
属性来指定提交的方式为multipart/form-data
。以下是一个简单的文件上传表单的例子:
<form action="upload.jsp" method="post" enctype="multipart/form-data">
<input type="file" name="file">
<input type="submit" value="上传">
</form>
在上传文件的JSP页面upload.jsp
中,需要使用Java文件上传类库,例如Apache Commons FileUpload,来处理文件上传逻辑。以下是一个使用Apache Commons FileUpload的例子:
<%@ page import="org.apache.commons.fileupload.*"%>
<%@ page import="org.apache.commons.fileupload.util.*"%>
<%@ page import="org.apache.commons.fileupload.disk.*"%>
<%
// Create a factory for disk-based file items
DiskFileItemFactory factory = new DiskFileItemFactory();
// Create a new file upload handler
ServletFileUpload upload = new ServletFileUpload(factory);
// Parse the request
List<FileItem> items = upload.parseRequest(request);
// Process the uploaded files
for (FileItem item : items) {
if (!item.isFormField()) {
String fileName = item.getName();
File uploadedFile = new File("path/to/uploads", fileName);
item.write(uploadedFile);
}
}
%>
将以上代码保存为upload.jsp
,在浏览器中访问upload.jsp
页面,即可上传文件并保存到指定的文件夹中。
步骤二:添加文件下载功能
接下来,我们需要添加文件下载功能。首先,我们需要在JSP页面上显示可供下载的文件列表,并使用<a>
标签为每个文件指定下载链接。以下是一个简单的文件下载列表的例子:
<ul>
<li><a href="download.jsp?file=example.zip">example.zip</a></li>
<li><a href="download.jsp?file=example.pdf">example.pdf</a></li>
</ul>
在文件下载的JSP页面download.jsp
中,我们需要通过获取URL参数file
来确定要下载的文件,并将该文件作为输出流写入到HTTP响应中,供用户下载。以下是一个简单的文件下载的例子:
<%@ page import="java.io.*"%>
<%@ page import="javax.servlet.ServletOutputStream"%>
<%
String fileName = request.getParameter("file");
String filePath = "path/to/uploads/" + fileName;
File file = new File(filePath);
if (file.exists()) {
response.setContentType("application/octet-stream");
response.setHeader("Content-Disposition", "attachment;filename=\"" + fileName + "\"");
FileInputStream in = new FileInputStream(file);
ServletOutputStream out = response.getOutputStream();
byte[] buffer = new byte[4096];
int length = 0;
while ((length = in.read(buffer)) > 0) {
out.write(buffer, 0, length);
}
in.close();
out.close();
} else {
out.print("File not found!");
}
%>
将以上代码保存为download.jsp
,在浏览器中访问download.jsp
页面,即可下载指定的文件。
示例一:上传图片并在网站上显示
现在,让我们来看一个实际的应用场景。假设我们需要允许用户上传图片并在网站上显示。首先,在JSP页面上添加文件上传功能,如上所述,然后在成功上传文件后,将文件名保存在一个List中。然后,在页面上展示已经上传的图片,并为每张图片添加下载链接。以下是一个简单的文件上传和图片展示的例子:
<%@ page import="org.apache.commons.fileupload.*"%>
<%@ page import="org.apache.commons.fileupload.util.*"%>
<%@ page import="org.apache.commons.fileupload.disk.*"%>
<%@ page import="java.io.*"%>
<%@ page import="java.util.List"%>
<%@ page contentType="text/html" pageEncoding="UTF-8"%>
<%
// Create a factory for disk-based file items
DiskFileItemFactory factory = new DiskFileItemFactory();
// Create a new file upload handler
ServletFileUpload upload = new ServletFileUpload(factory);
// Parse the request
List<FileItem> items = upload.parseRequest(request);
// Process the uploaded files
List<String> uploadedFiles = new ArrayList<String>();
for (FileItem item : items) {
if (!item.isFormField()) {
String fileName = item.getName();
File uploadedFile = new File("path/to/uploads", fileName);
item.write(uploadedFile);
uploadedFiles.add(fileName);
}
}
%>
<!DOCTYPE html>
<html>
<head>
<title>文件上传示例</title>
</head>
<body>
<h1>上传图片</h1>
<form action="upload.jsp" method="post" enctype="multipart/form-data">
<input type="file" name="file">
<input type="submit" value="上传">
</form>
<h1>已上传图片</h1>
<ul>
<%
for (String file : uploadedFiles) {
out.println("<li><img src='path/to/uploads/" + file + "' width='100'><br><a href='download.jsp?file=" + file + "'>" + file + "</a></li>");
}
%>
</ul>
</body>
</html>
在以上代码中,我们利用了<img>
标签来显示图片,它会自动从指定的URL路径加载图片。
示例二:下载PDF格式的表格
现在,让我们看另一个实例,假设我们需要为用户提供一个可以下载PDF格式表格的页面。首先,我们需要创建一个表格,并使用JavaScript将其转换为PDF格式。然后,在JSP页面上添加下载链接,并为其添加参数file
以便指定下载的文件。以下是一个简单的例子:
<!DOCTYPE html>
<html>
<head>
<title>文件下载示例</title>
<script src="//cdnjs.cloudflare.com/ajax/libs/jspdf/1.3.2/jspdf.min.js"></script>
<script>
function downloadPDF() {
var doc = new jsPDF();
doc.text(20, 20, 'This is a PDF table!');
doc.autoTable({html: '#my-table'});
doc.save('example.pdf');
}
</script>
</head>
<body>
<h1>下载PDF格式表格</h1>
<button onclick="downloadPDF()">下载表格</button>
<table id="my-table">
<thead>
<tr>
<th>Name</th>
<th>Gender</th>
<th>Age</th>
</tr>
</thead>
<tbody>
<tr>
<td>John Doe</td>
<td>Male</td>
<td>30</td>
</tr>
<tr>
<td>Jane Doe</td>
<td>Female</td>
<td>25</td>
</tr>
</tbody>
</table>
<br>
<a href="download.jsp?file=example.pdf">下载表格</a>
</body>
</html>
在以上代码中,我们使用了jsPDF库来生成PDF格式的表格,并将其保存为example.pdf
文件。然后,我们在页面上添加下载链接,并指定文件名为example.pdf
。
接下来,在download.jsp
中,我们需要使用以下代码将指定的PDF文件作为数据流写入到HTTP响应中,并提供给用户下载:
<%@ page import="java.io.*"%>
<%@ page import="javax.servlet.ServletOutputStream"%>
<%
String fileName = request.getParameter("file");
String filePath = "path/to/uploads/" + fileName;
File file = new File(filePath);
if (file.exists()) {
response.setContentType("application/octet-stream");
response.setHeader("Content-Disposition", "attachment;filename=\"" + fileName + "\"");
FileInputStream in = new FileInputStream(file);
ServletOutputStream out = response.getOutputStream();
byte[] buffer = new byte[4096];
int length = 0;
while ((length = in.read(buffer)) > 0) {
out.write(buffer, 0, length);
}
in.close();
out.close();
} else {
out.print("File not found!");
}
%>
以上就是“JSP文件上传与下载实例代码”的完整攻略,希望可以帮到您!
本文标题为:jsp文件上传与下载实例代码
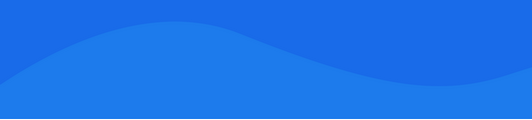
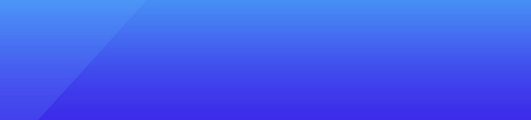
基础教程推荐
- 详解RSA加密算法的原理与Java实现 2023-06-17
- Java使用arthas修改日志级别详解 2023-01-13
- SpringMVC使用注解配置方式 2022-11-20
- Java时间戳类Instant的使用详解 2023-06-02
- Java SpringBoot自动配置原理详情 2023-03-21
- Java Service Wrapper 发布Java程序为Windows服务 2023-09-01
- SpringBoot接口如何统一异常处理 2023-02-28
- SpringBoot复杂参数应用详细讲解 2023-06-05
- java如何从地址串中解析提取省市区(完美匹配中国所有地址) 2023-02-19
- 如何使用Collections.reverse对list集合进行降序排序 2023-08-10