下面是Spring Boot集成Thymeleaf模板引擎的完整步骤,包含两个示例说明。
下面是Spring Boot集成Thymeleaf模板引擎的完整步骤,包含两个示例说明。
1. 添加依赖
在pom.xml文件中添加如下依赖:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
这样就可以使用Thymeleaf模板引擎了。
2. 配置Thymeleaf
在application.properties文件中添加如下配置:
spring.thymeleaf.cache=false
spring.thymeleaf.check-template=true
spring.thymeleaf.check-template-location=true
spring.thymeleaf.enabled=true
spring.thymeleaf.mode=HTML
spring.thymeleaf.prefix=classpath:/templates/
spring.thymeleaf.suffix=.html
以上配置是常用的配置项,其中:
- spring.thymeleaf.cache=false:禁用缓存,这样在修改模板文件后不需要重启程序。
- spring.thymeleaf.prefix
:设置Thymeleaf模板文件所在的目录。
- spring.thymeleaf.suffix
:设置Thymeleaf模板文件的后缀名。
3. 编写控制器
@Controller
public class HomeController {
@GetMapping("/")
public String index(Model model) {
model.addAttribute("message", "Hello, Thymeleaf!");
return "index";
}
}
以上代码中,@Controller
注解用于声明HomeController为控制器,@GetMapping("/")
表示当访问根路径时,调用index方法。
Model
对象用于向模板中添加数据,这里向模板中添加了一个名为message的数据。
return "index"
表示返回名为index的模板文件。由于配置中设置了classpath:/templates/
为Thymeleaf模板文件所在的目录,所以这里返回的是classpath:/templates/index.html文件。
4. 编写模板文件
在classpath:/templates/
目录下创建index.html文件,并编写如下代码:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Spring Boot Thymeleaf Demo</title>
</head>
<body>
<h1 th:text="${message}"></h1>
</body>
</html>
以上代码中,th:text="${message}"
表示将模板中的message变量替换为HomeController中添加的数据。
示例1
我们现在启动程序,访问http://localhost:8080/,可以看到页面上显示了“Hello, Thymeleaf!”这个信息。
示例2
在HomeController中添加一个名为books的数据:
@Controller
public class HomeController {
@GetMapping("/")
public String index(Model model) {
model.addAttribute("message", "Hello, Thymeleaf!");
List<Book> books = new ArrayList<>();
books.add(new Book("Java编程思想", "Bruce Eckel"));
books.add(new Book("Spring实战", "Craig Walls"));
books.add(new Book("深入理解Java虚拟机", "周志明"));
model.addAttribute("books", books);
return "index";
}
public static class Book {
private String name;
private String author;
public Book(String name, String author) {
this.name = name;
this.author = author;
}
public String getName() {
return name;
}
public String getAuthor() {
return author;
}
}
}
在模板文件index.html中添加如下代码:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Spring Boot Thymeleaf Demo</title>
</head>
<body>
<h1 th:text="${message}"></h1>
<table>
<thead>
<tr>
<th>Name</th>
<th>Author</th>
</tr>
</thead>
<tbody>
<tr th:each="book : ${books}">
<td th:text="${book.name}"></td>
<td th:text="${book.author}"></td>
</tr>
</tbody>
</table>
</body>
</html>
在页面上我们可以看到一个表格,其中显示了三本书的信息。这是因为HomeController中添加了一个名为books的数据,并将其传递给了模板文件。在模板中循环遍历这个数据,并且使用th:text
将书的名字和作者显示在页面上。
本文标题为:Spring Boot集成Thymeleaf模板引擎的完整步骤
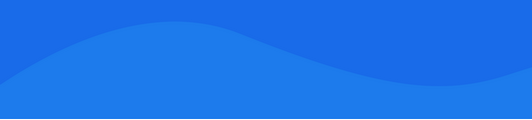
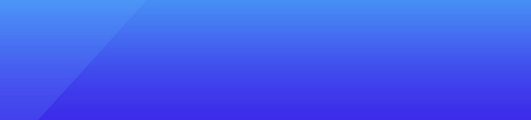
基础教程推荐
- 浅谈apache和nginx的rewrite的区别 2023-12-15
- Java实现注册登录跳转 2023-01-13
- Jsp+Servlet实现文件上传下载 文件列表展示(二) 2023-07-30
- Java知识梳理之泛型用法详解 2023-04-06
- JVM jstack实战之死锁问题详解 2023-06-10
- Spring Boot整合阿里开源中间件Canal实现数据增量同步 2023-01-29
- Java特性 Lambda 表达式和函数式接口 2023-01-18
- springboot max-http-header-size最大长度的那些事及JVM调优方式 2023-06-06
- Mybatis动态SQL之where标签用法说明 2023-02-04
- Java中 SLF4J和Logback和Log4j和Logging的区别与联系 2023-05-19