下面是“JSP中使用JavaScript动态插入删除输入框实现代码”的完整攻略。
下面是“JSP中使用JavaScript动态插入删除输入框实现代码”的完整攻略。
简介
JSP是一种动态网页技术,而JavaScript是一种脚本语言,两者可以结合使用,达到更好的用户交互效果。此次攻略将详细讲解如何在JSP页面中使用JavaScript实现动态插入删除输入框的功能。
实现步骤
实现插入输入框功能
- 在JSP页面中添加一个按钮,用于触发插入输入框的功能。
<button onclick="addInput()">添加输入框</button>
- 在JavaScript中编写添加输入框的函数。
function addInput() {
var inputBox = document.createElement('div');
inputBox.innerHTML = '<input type="text" name="inputField" /><button onclick="removeInput(this)">删除</button>';
document.getElementById('inputArea').appendChild(inputBox);
}
上述函数的具体实现过程为:
- 创建一个div元素,用于包裹输入框和删除按钮。
- 在div中插入一个文本输入框和一个删除按钮。
-
将新创建的div添加到页面指定的输入框区域中。
-
在JSP页面中指定用于渲染新添加输入框的区域。
<div id="inputArea"></div>
完整示例代码:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>动态添加输入框</title>
</head>
<body>
<div id="inputArea"></div>
<button onclick="addInput()">添加输入框</button>
<script>
function addInput() {
var inputBox = document.createElement('div');
inputBox.innerHTML = '<input type="text" name="inputField" /><button onclick="removeInput(this)">删除</button>';
document.getElementById('inputArea').appendChild(inputBox);
}
function removeInput(inputNode) {
inputNode.parentNode.remove();
}
</script>
</body>
</html>
实现删除输入框功能
在添加输入框时我们已经实现了删除按钮的插入,下面我们需要编写一个函数实现删除按钮的点击操作。
- 在JSP页面中添加调用删除函数的按钮。
<button onclick="removeInput(this)">删除</button>
- 在JavaScript中编写删除输入框的函数。
function removeInput(inputNode) {
inputNode.parentNode.remove();
}
上述函数的具体实现过程为:
- 获取当前点击的删除按钮节点所在的输入框节点。
- 将该输入框节点从页面中删除。
完整示例代码:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>动态添加输入框</title>
</head>
<body>
<div id="inputArea"></div>
<button onclick="addInput()">添加输入框</button>
<script>
function addInput() {
var inputBox = document.createElement('div');
inputBox.innerHTML = '<input type="text" name="inputField" /><button onclick="removeInput(this)">删除</button>';
document.getElementById('inputArea').appendChild(inputBox);
}
function removeInput(inputNode) {
inputNode.parentNode.remove();
}
</script>
</body>
</html>
如上,我们已经实现了在JSP页面中使用JavaScript动态插入删除输入框的功能。
示例说明
示例1:动态添加多个输入框
在此示例中,我们演示如何使用动态添加输入框的方式,让用户可以动态添加多个输入框。
首先在JSP页面中添加一个按钮,并指定输入框的区域。
<div id="inputArea"></div>
<button onclick="addInput()">添加输入框</button>
然后在JavaScript中编写添加输入框的函数。
function addInput() {
var inputBox = document.createElement('div');
inputBox.innerHTML = '<input type="text" name="inputField" /><button onclick="removeInput(this)">删除</button>';
document.getElementById('inputArea').appendChild(inputBox);
}
最后在浏览器中打开页面,点击按钮就可以动态添加多个输入框了。
示例2:动态删除指定输入框
在此示例中,我们演示如何使用动态删除输入框的方式,让用户可以在多个输入框中删除指定的输入框。
在JSP页面中添加多个输入框,每个输入框绑定一个删除按钮。
<div id="inputArea">
<div><input type="text" name="inputField" /><button onclick="removeInput(this)">删除</button></div>
<div><input type="text" name="inputField" /><button onclick="removeInput(this)">删除</button></div>
<div><input type="text" name="inputField" /><button onclick="removeInput(this)">删除</button></div>
</div>
然后在JavaScript中编写删除输入框的函数。
function removeInput(inputNode) {
inputNode.parentNode.remove();
}
最后在浏览器中打开页面,点击每个输入框上的删除按钮,就可以删除指定的输入框。
本文标题为:JSP中使用JavaScript动态插入删除输入框实现代码
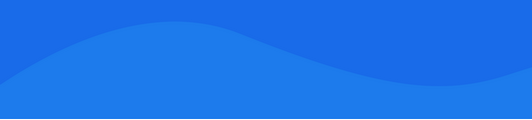
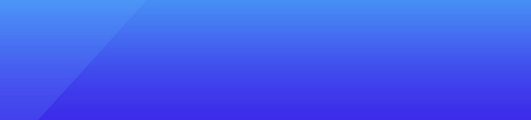
基础教程推荐
- Spring 中 Bean 的生命周期详解 2023-05-14
- Java实现字符串转为驼峰格式的方法详解 2023-02-19
- Java中的多个SQL语句(带?allowMultiQueries = true) 2023-11-06
- ConditionalOnProperty配置swagger不生效问题及解决 2023-01-02
- java实现多线程文件的断点续传 2023-01-29
- Spring component-scan XML配置与@ComponentScan注解配置 2023-05-08
- Spring获取ApplicationContext对象工具类的实现方法 2023-07-29
- Java使用MessageFormat应注意的问题 2023-01-18
- java – 当JSON对象作为字符串文字插入时,MySQL JSON列丢失小数精度 2023-11-04
- SpringBoot Webflux创建TCP/UDP server并使用handler解析数据 2022-10-30