下面是一份JavaWeb分页的实现代码实例攻略。
下面是一份JavaWeb分页的实现代码实例攻略。
1. 需求分析
在网站中,当数据量较大时,我们需要把它分页显示,从而提高用户体验。而JavaWeb框架中可以使用JSP来实现分页的功能。具体来说,我们需要针对以下几个步骤实现分页功能。
2. 分页实现步骤
2.1 准备工作
首先,我们需要创建一个数据表来存储数据,其次我们需要创建一个JavaBean来封装数据,如下:
public class Student {
private int id;
private String name;
private int age;
// 必须要有getter和setter方法
// ...
}
2.2 实现数据查询
其次,我们需要对数据库进行查询操作,可以使用JDBC或MyBatis来实现。以JDBC为例,代码如下:
public List<Student> queryByPage(int pageSize, int pageNum) {
Connection conn = null;
PreparedStatement ps = null;
ResultSet rs = null;
try {
Class.forName("com.mysql.jdbc.Driver");
String url = "jdbc:mysql://localhost:3306/test";
String user = "root";
String password = "123456";
conn = DriverManager.getConnection(url, user, password);
String sql = "select * from student limit ?, ?";
ps = conn.prepareStatement(sql);
ps.setInt(1, (pageNum - 1) * pageSize); // 计算从哪行开始查询
ps.setInt(2, pageSize); // 每页显示的行数
rs = ps.executeQuery();
List<Student> list = new ArrayList<>();
while (rs.next()) {
Student student = new Student();
student.setId(rs.getInt("id"));
student.setName(rs.getString("name"));
student.setAge(rs.getInt("age"));
list.add(student);
}
return list;
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (rs != null) rs.close();
if (ps != null) ps.close();
if (conn != null) conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
return null;
}
2.3 显示数据
通过查询,我们获得了当前页的数据,接下来就是将其展示给用户。在JSP中,我们可以使用JSTL和EL表达式来操作JavaBean对象。比如:
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<!DOCTYPE html>
<html>
<head>
<title>分页</title>
</head>
<body>
<table border="1">
<tr>
<th>ID</th>
<th>姓名</th>
<th>年龄</th>
</tr>
<c:forEach items="${students}" var="student">
<tr>
<td>${student.id}</td>
<td>${student.name}</td>
<td>${student.age}</td>
</tr>
</c:forEach>
</table>
</body>
</html>
在这里,我们使用了
2.4 实现分页功能
最后一步是实现分页功能,这里我们可以使用Bootstrap的分页组件。具体实现如下:
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<!DOCTYPE html>
<html>
<head>
<title>分页</title>
<link rel="stylesheet" href="https://cdn.bootcss.com/bootstrap/3.3.7/css/bootstrap.min.css">
</head>
<body>
<table class="table table-striped">
<tr>
<th>ID</th>
<th>姓名</th>
<th>年龄</th>
</tr>
<c:forEach items="${students}" var="student">
<tr>
<td>${student.id}</td>
<td>${student.name}</td>
<td>${student.age}</td>
</tr>
</c:forEach>
</table>
<ul class="pagination">
<c:if test="${currentPage > 1}">
<li><a href="?pageNum=${currentPage-1}">«</a></li>
</c:if>
<c:forEach begin="1" end="${totalPage}" var="i">
<c:choose>
<c:when test="${i eq currentPage}">
<li class="active"><a href="?pageNum=${i}">${i}</a></li>
</c:when>
<c:otherwise>
<li><a href="?pageNum=${i}">${i}</a></li>
</c:otherwise>
</c:choose>
</c:forEach>
<c:if test="${currentPage < totalPage}">
<li><a href="?pageNum=${currentPage+1}">»</a></li>
</c:if>
</ul>
</body>
</html>
在这里,我们使用了
3. 示例说明
以上是对JavaWeb分页的实现代码实例的完整攻略,下面分别列举两个示例说明。
示例1:简单分页
比如我们要在页面上显示10条数据,每页只显示3条数据,那么访问第1页只显示从第0-2条数据,访问第2页只显示从第3-5条数据,访问第3页只显示从第6-8条数据,访问第4页只显示第9条数据。
示例2:正常分页
比如我们要在页面上显示100条数据,每页只显示10条数据,那么访问第1页只显示从第0-9条数据,访问第2页只显示从第10-19条数据,访问第3页只显示从第20-29条数据,访问第4页只显示第30-39条数据,以此类推。同时,如果当前数据不足10条时(比如最后一页只有7条数据),就只显示最后7条。
本文标题为:JavaWeb分页的实现代码实例
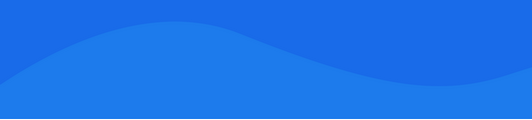
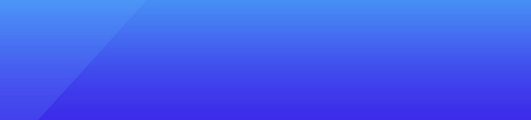
基础教程推荐
- java内部类的最详细详解 2023-01-09
- JSP + Servlet实现生成登录验证码示例 2023-08-01
- 使用spring动态获取接口的不同实现类 2022-11-04
- java Object转byte与byte转Object方式 2023-04-18
- Java虚拟机启动过程探索 2022-11-20
- java 如何查看jar包加载顺序 2023-08-10
- java eclipse 中文件的上传和下载示例解析 2023-12-15
- Mybatis返回Map数据方式示例 2022-12-04
- SpringBoot入门教程详解 2023-01-29
- Java详细分析LCN框架分布式事务 2023-03-22