关于JSP遍历文件夹下的文件,可以通过以下步骤实现:
关于JSP遍历文件夹下的文件,可以通过以下步骤实现:
1.获取文件夹路径
首先需要获取要遍历的文件夹路径,可以通过JSP页面中的request对象获取,例如:
String folderPath = request.getParameter("folderPath"); //获取前端传来的文件夹路径
File folder = new File(folderPath); //将路径转换成文件对象
其中,我们通过request.getParameter()
获取前端传来的文件夹路径,然后将其转换成File
对象,方便后续操作。
2.遍历文件夹
接下来就是遍历文件夹下的文件。可以通过递归的方式实现:
public static void getFileList(File folder) {
File[] files = folder.listFiles(); //获取文件夹下的所有文件
for (File file : files) {
if (file.isDirectory()) {
getFileList(file); //如果是文件夹,则递归调用
} else {
//如果是文件,则进行相应的操作
String fileName = file.getName();
String filePath = file.getAbsolutePath();
//TODO:进行具体的操作,例如将文件名和路径输出到页面等
}
}
}
上述代码中,我们首先通过listFiles()
方法获取文件夹下的所有文件,然后使用for循环进行遍历。如果某个文件是文件夹,那么就递归调用getFileList()
方法继续遍历;否则,就进行相应的操作,例如将文件名和路径输出到页面等。
3.将文件信息输出到页面
最后,我们需要将遍历的文件信息输出到JSP页面上。可以通过JSP的JSTL标签库中的<c:forEach>
标签实现:
<c:forEach items="${fileList}" var="file">
<tr>
<td>${file.name}</td>
<td>${file.path}</td>
</tr>
</c:forEach>
上述代码中,我们通过JSTL的<c:forEach>
标签循环遍历fileList
中的文件信息,然后将文件名和文件路径分别输出到表格中。
下面给出一个完整的示例,以演示如何实现JSP遍历文件夹下的文件:
<%@ page contentType="text/html; charset=UTF-8" language="java" %>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<html>
<head>
<title>遍历文件夹下的文件</title>
</head>
<body>
<form action="" method="post">
<label for="folderPath">文件夹路径:</label>
<input type="text" name="folderPath" id="folderPath">
<input type="submit" value="开始遍历">
</form>
<hr>
<c:if test="${not empty fileList}">
<table>
<thead>
<tr>
<th>文件名</th>
<th>文件路径</th>
</tr>
</thead>
<tbody>
<c:forEach items="${fileList}" var="file">
<tr>
<td>${file.name}</td>
<td>${file.path}</td>
</tr>
</c:forEach>
</tbody>
</table>
</c:if>
<%
if ("POST".equalsIgnoreCase(request.getMethod())) {
String folderPath = request.getParameter("folderPath");
File folder = new File(folderPath);
List<File> fileList = new ArrayList<>();
getFileList(folder, fileList);
request.setAttribute("fileList", fileList);
}
public static void getFileList(File folder, List<File> fileList) {
File[] files = folder.listFiles();
for (File file : files) {
if (file.isDirectory()) {
getFileList(file, fileList);
} else {
fileList.add(file);
}
}
}
%>
</body>
</html>
在上述示例代码中,我们在form表单中添加了一个输入框,用户可以在其中输入要遍历的文件夹路径。然后,通过JSTL的<c:if>
标签判断fileList
是否为空,如果不为空,则输出文件信息到表格中。最后是通过getFileList()
方法递归遍历文件夹,并将遍历到的文件信息放入到fileList
中。
本文标题为:jsp遍历文件夹下的文件的代码
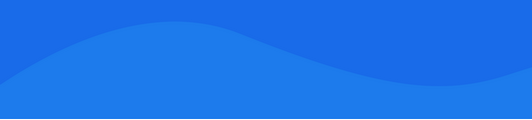
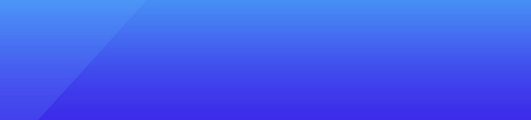
基础教程推荐
- JSP+Servlet+JavaBean实现登录网页实例详解 2023-12-16
- IDEA中实体类(POJO)与JSON快速互转问题 2023-04-17
- java IP归属地功能实现详解 2023-02-28
- Java MyBatis框架环境搭建详解 2023-04-12
- 关于Spring的AnnotationAwareAspectJAutoProxyCreator类解析 2023-07-15
- SpringBoot+Querydsl 框架实现复杂查询解析 2022-11-29
- SpringBoot注入自定义的配置文件的方法详解 2023-05-25
- java锁机制ReentrantLock源码实例分析 2023-06-09
- SpringMVC RESTFul实战案例访问首页 2022-11-20
- Linux系统下安装和卸载JDK8的方式 2023-05-14