让我们来详细讲解 “JSP 多个文件打包下载代码”的完整攻略。
让我们来详细讲解 “JSP 多个文件打包下载代码”的完整攻略。
1. 准备工作
在开始之前,我们需要在项目中引入如下三个依赖:
<!-- 需要用到的 Apache commons 库 -->
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.6</version>
</dependency>
<!-- 需要用到的 JSP 插件库 -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet.jsp</artifactId>
<version>2.2.1-b03</version>
</dependency>
<!-- 需要用到的 ZIP 压缩库 -->
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-compress</artifactId>
<version>1.18</version>
</dependency>
2. 实现思路
接下来,我们来讲解下实现思路,要实现多个文件打包下载,我们需要做以下几步:
- 首先,将要下载的文件保存在一个以当前时间戳为文件名的临时文件夹中。
- 通过 ZipArchiveOutputStream 类创建一个 ZIP 文件流。
- 逐个读取之前保存的文件并将文件通过 ZIP 文件流写入到 ZIP 包中。
- 将 ZIP 文件流写入到响应的输出流中,实现打包下载。
3. 代码实现
有了以上的思路,代码实现就会变得简单。
以下是一个简单的示例代码:
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// 假设要下载的文件路径存在一个数组中
String[] fileNames = {"path/to/file1", "path/to/file2", "path/to/file3"};
// 在服务器上创建一个临时文件夹,并以当前时间戳为文件名
File zipFile = new File(System.currentTimeMillis() + ".zip");
zipFile.createNewFile();
FileOutputStream fos = new FileOutputStream(zipFile);
ZipArchiveOutputStream zos = new ZipArchiveOutputStream(fos);
zos.setEncoding("GBK");
// 将要下载的文件读入到 ZIP 文件流中
for (int i = 0; i < fileNames.length; i++) {
File file = new File(fileNames[i]);
if (file.exists()) {
ZipArchiveEntry zipArchiveEntry = new ZipArchiveEntry(file, file.getName());
zos.putArchiveEntry(zipArchiveEntry);
FileInputStream fis = new FileInputStream(file);
byte[] buffer = new byte[1024 * 5];
int length;
while ((length = fis.read(buffer)) != -1) {
zos.write(buffer, 0, length);
}
fis.close();
zos.closeArchiveEntry();
}
}
zos.finish();
zos.close();
// 将 ZIP 文件流输出给客户端浏览器下载
response.setContentType("application/x-zip-compressed");
response.setHeader("Content-Disposition", "attachment;filename=" + zipFile.getName());
OutputStream out = response.getOutputStream();
FileInputStream in = new FileInputStream(zipFile);
byte[] buffer = new byte[1024 * 5];
int length;
while ((length = in.read(buffer)) != -1) {
out.write(buffer, 0, length);
}
in.close();
out.flush();
out.close();
// 删除临时文件
zipFile.delete();
}
以上代码基本涵盖了多个文件打包下载的全部内容,希望对你有所帮助!
另外,还有一个示例代码,用于演示如何下载指定文件夹下的所有文件:
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// 假设要下载的文件夹路径存在一个变量中
String folderPath = "path/to/folder";
// 在服务器上创建一个临时文件夹,并以当前时间戳为文件名
File zipFile = new File(System.currentTimeMillis() + ".zip");
zipFile.createNewFile();
FileOutputStream fos = new FileOutputStream(zipFile);
ZipArchiveOutputStream zos = new ZipArchiveOutputStream(fos);
zos.setEncoding("GBK");
// 遍历文件夹中的所有文件,读入到 ZIP 文件流中
File folder = new File(folderPath);
if (folder.exists() && folder.isDirectory()) {
File[] files = folder.listFiles();
for (int i = 0; i < files.length; i++) {
if (files[i].isFile()) {
ZipArchiveEntry zipArchiveEntry = new ZipArchiveEntry(files[i], files[i].getName());
zos.putArchiveEntry(zipArchiveEntry);
FileInputStream fis = new FileInputStream(files[i]);
byte[] buffer = new byte[1024 * 5];
int length;
while ((length = fis.read(buffer)) != -1) {
zos.write(buffer, 0, length);
}
fis.close();
zos.closeArchiveEntry();
}
}
}
zos.finish();
zos.close();
// 将 ZIP 文件流输出给客户端浏览器下载
response.setContentType("application/x-zip-compressed");
response.setHeader("Content-Disposition", "attachment;filename=" + zipFile.getName());
OutputStream out = response.getOutputStream();
FileInputStream in = new FileInputStream(zipFile);
byte[] buffer = new byte[1024 * 5];
int length;
while ((length = in.read(buffer)) != -1) {
out.write(buffer, 0, length);
}
in.close();
out.flush();
out.close();
// 删除临时文件
zipFile.delete();
}
沃梦达教程
本文标题为:JSP 多个文件打包下载代码
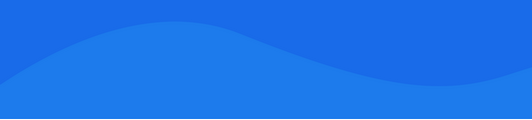
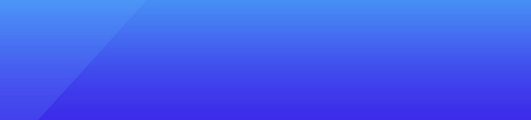
基础教程推荐
猜你喜欢
- Java设计模式之建造者模式 2023-06-01
- springboot实现指定mybatis中mapper文件扫描路径 2022-12-07
- java – JPA @SqlResultSetMapping无法处理要映射到空POJO的空sql结果 – 而是抛出异常 2023-11-08
- Java使用定时器编写一个简单的抢红包小游戏 2023-02-11
- Java正则校验密码至少包含字母数字特殊符号中的2种实例代码 2023-03-30
- js中let能否完全替代IIFE 2023-12-15
- HttpClient实现远程调用 2023-04-12
- Spring 配置文件XML头部文件模板实例详解 2023-07-31
- SpringCloud Feign远程调用实现详解 2023-07-01
- 基于Java开发实现ATM系统 2023-04-12