我将为您讲解使用SpringMVC返回JSON字符串的实例攻略。
我将为您讲解使用SpringMVC返回JSON字符串的实例攻略。
1. 实现步骤
SpringMVC实现返回JSON字符串的步骤大致如下:
- 在pom.xml文件添加依赖:
<dependencies>
<!-- SpringMVC核心包 -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.2.0.RELEASE</version>
</dependency>
<!-- FastJson包 -->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.62</version>
</dependency>
</dependencies>
- 在SpringMVC配置文件中添加配置:
<mvc:annotation-driven/>
<bean id="jsonConverter" class="org.springframework.http.converter.json.MappingJackson2HttpMessageConverter" />
- 在Controller中处理请求,将返回结果转换为JSON字符串并返回:
@RequestMapping("/api/info")
@ResponseBody
public String getInfo() {
JSONObject json = new JSONObject();
json.put("name", "example");
json.put("age", 20);
return json.toJSONString();
}
其中@Controller注解表示此类是Controller,@RequestMapping注解表示此方法处理请求的URL地址,@ResponseBody注解表示此方法返回JSON字符串。
2. 实例示范
以下是两个简单的示例,说明如何使用SpringMVC返回JSON字符串。
示例一
1. pom.xml文件添加依赖
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.2.0.RELEASE</version>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.62</version>
</dependency>
</dependencies>
2. SpringMVC配置文件中添加配置
<mvc:annotation-driven/>
<bean id="jsonConverter" class="org.springframework.http.converter.json.MappingJackson2HttpMessageConverter" />
3. 编写Controller代码
package com.example.controller;
import com.alibaba.fastjson.JSONObject;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
@Controller
public class HelloController {
@RequestMapping("/hello")
@ResponseBody
public String hello() {
JSONObject json = new JSONObject();
json.put("message", "Hello World!");
return json.toJSONString();
}
}
4. 启动程序测试
访问http://localhost:8080/hello,将会得到如下JSON字符串:
{
"message": "Hello World!"
}
示例二
1. pom.xml文件添加依赖
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.2.0.RELEASE</version>
</dependency>
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>1.2.62</version>
</dependency>
</dependencies>
2. SpringMVC配置文件中添加配置
<mvc:annotation-driven/>
<bean id="jsonConverter" class="org.springframework.http.converter.json.MappingJackson2HttpMessageConverter" />
3. 编写Controller代码
package com.example.controller;
import com.alibaba.fastjson.JSONObject;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
@Controller
public class UserController {
@RequestMapping("/user")
@ResponseBody
public String getUser() {
User user = new User();
user.setName("example");
user.setEmail("example@example.com");
user.setAge(20);
return user.toJSONString();
}
private class User {
private String name;
private String email;
private int age;
//getters and setters
public String toJSONString() {
JSONObject json = new JSONObject();
json.put("name", this.getName());
json.put("email", this.getEmail());
json.put("age", this.getAge());
return json.toJSONString();
}
}
}
4. 启动程序测试
访问http://localhost:8080/user,将会得到如下JSON字符串:
{
"name": "example",
"email": "example@example.com",
"age": 20
}
结束语
以上就是使用SpringMVC返回JSON字符串的实例攻略,通过这两个示例,您应该能够掌握如何使用SpringMVC返回JSON字符串,希望对您有所帮助。
沃梦达教程
本文标题为:使用SpringMVC返回json字符串的实例讲解
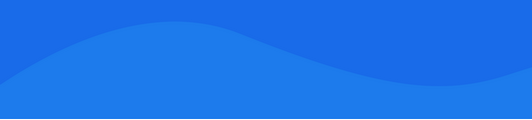
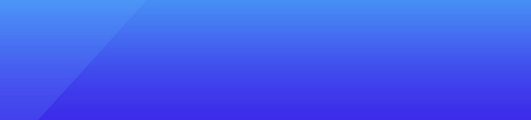
基础教程推荐
猜你喜欢
- Java开源项目用法介绍 2023-10-08
- Java实例讲解枚举enum的实现 2022-12-11
- 解决在微服务环境下远程调用feign和异步线程存在请求数据丢失问题 2022-11-11
- Sentinel源码解析入口类和SlotChain构建过程详解 2023-06-06
- springboot整合shardingsphere和seata实现分布式事务的实践 2023-03-21
- 关于idea2022.2 闪退的问题 2023-04-12
- 关于JSCH使用自定义连接池的说明 2023-06-02
- 利用AOP实现系统告警的方法详解 2023-05-19
- 如何解决Webservice第一次访问特别慢的问题 2023-02-10
- Java如何提供给第三方使用接口方法详解 2022-09-03