下面我将为你详细讲解Java实现图片上传至FastDFS入门教程的完整攻略。
下面我将为你详细讲解Java实现图片上传至FastDFS入门教程的完整攻略。
什么是FastDFS?
FastDFS是用于分布式文件存储的开源软件,支持文件上传、下载以及文件元数据的管理等操作。它采用了分布式的架构设计,可以实现高可用、高性能的文件存储。
准备工作
-
创建一个Maven项目。
-
在项目的pom.xml文件中添加FastDFS客户端的依赖。
<dependency>
<groupId>com.github.tobato</groupId>
<artifactId>fastdfs-client</artifactId>
<version>1.29.4</version>
</dependency>
- 在项目的配置文件中添加FastDFS的配置信息。
# FastDFS配置
fdfs.client.tracker-list=192.168.1.2:22122
实现图片上传
以下是使用FastDFS实现图片上传的示例代码:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.web.multipart.MultipartFile;
import java.io.IOException;
@Service
public class FileServiceImpl implements FileService {
@Autowired
private FastFileStorageClient fastFileStorageClient;
@Override
public String uploadFile(MultipartFile file) throws IOException {
StorePath storePath = fastFileStorageClient.uploadFile(file.getInputStream(), file.getSize(), FilenameUtils.getExtension(file.getOriginalFilename()), null);
return storePath.getFullPath();
}
}
在以上代码中,我们通过FastDFS客户端的uploadFile方法将文件上传至FastDFS,并获取到上传后的文件路径。
实现图片下载
以下是使用FastDFS实现图片下载的示例代码:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
@Service
public class FileServiceImpl implements FileService {
@Autowired
private FastFileStorageClient fastFileStorageClient;
@Override
public byte[] downloadFile(String filePath) throws IOException {
byte[] bytes = null;
InputStream inputStream = null;
ByteArrayOutputStream outputStream = null;
try {
inputStream = fastFileStorageClient.downloadFile(filePath.substring(0, filePath.indexOf("/")), filePath.substring(filePath.indexOf("/") + 1));
outputStream = new ByteArrayOutputStream();
byte[] buffer = new byte[4096];
int n = 0;
while (-1 != (n = inputStream.read(buffer))) {
outputStream.write(buffer, 0, n);
}
bytes = outputStream.toByteArray();
} finally {
if (null != outputStream) {
outputStream.close();
}
if (null != inputStream) {
inputStream.close();
}
}
return bytes;
}
}
在以上代码中,我们通过FastDFS客户端的downloadFile方法将文件下载至本地,最终获取到文件的二进制数据。
总结
通过以上的实现,我们可以看到,FastDFS提供了一个简单易用的方式来进行文件的存储和访问。同时,它还具备一定的扩展性,可以支持集群部署,从而提高文件存储的可用性和性能。
沃梦达教程
本文标题为:Java实现图片上传至FastDFS入门教程
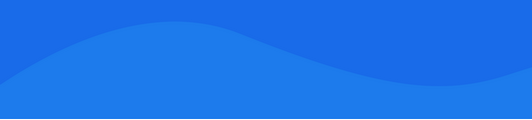
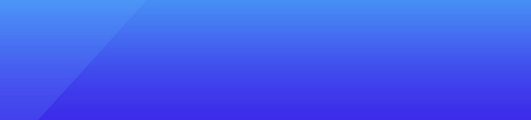
基础教程推荐
猜你喜欢
- Spring创建Bean的生命周期详析 2023-06-02
- java – 如何在MySQL中的INT列中区分0和null 2023-11-08
- IntelliJ IDEA 2022.1.1创建java项目的详细方法步骤 2023-03-07
- MyBatis if test 判断字符串相等不生效问题 2023-06-10
- Java手写线程池之向JDK线程池进发 2023-06-23
- SSM框架JSP使用Layui实现layer弹出层效果 2023-07-30
- Java+swing实现抖音上的表白程序详解 2023-01-29
- java – 商业智能和NoSQL 2023-11-07
- SpringMVC请求数据详解讲解 2023-03-21
- Java中实现两个线程交替运行的方法 2023-08-10