在Android应用的开发中,经常需要与远程服务器进行网络通信来获取数据,这就需要使用Android网络编程来实现。本文将介绍Android网络编程中几种常见的网络通信方式,并通过示例来说明。
Android 网络编程之网络通信几种方式实例分享
在Android应用的开发中,经常需要与远程服务器进行网络通信来获取数据,这就需要使用Android网络编程来实现。本文将介绍Android网络编程中几种常见的网络通信方式,并通过示例来说明。
1. HttpURLConnection
HttpURLConnection 是一个用于发送HTTP/HTTPS请求和接收HTTP响应的Java类。在Android应用中,我们可以通过HttpURLConnection来实现网络通信。下面是一个HTTP GET请求的示例:
new Thread(new Runnable() {
@Override
public void run() {
try {
URL url = new URL("https://www.example.com/api/data");
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
// 读取响应数据
BufferedReader reader = new BufferedReader(new InputStreamReader(conn.getInputStream()));
StringBuilder sb = new StringBuilder();
String line;
while ((line = reader.readLine()) != null) {
sb.append(line);
}
reader.close();
// 处理响应数据
String responseData = sb.toString();
Log.d("TAG", responseData);
conn.disconnect();
} catch (Exception e) {
e.printStackTrace();
}
}
}).start();
2. OkHttp
OkHttp 是一个基于 Java的HTTP客户端,支持访问HTTPS和HTTP。它比 HttpURLConnection 更加简单易用,并且能够对HTTP请求和响应进行自定义配置。下面是一个使用OkHttp发送HTTP POST请求并处理响应的示例:
new Thread(new Runnable() {
@Override
public void run() {
try {
OkHttpClient client = new OkHttpClient();
RequestBody formBody = new FormBody.Builder()
.add("username", "admin")
.add("password", "123456")
.build();
Request request = new Request.Builder()
.url("https://www.example.com/api/login")
.post(formBody)
.build();
// 发送请求
Response response = client.newCall(request).execute();
// 处理响应数据
String responseData = response.body().string();
Log.d("TAG", responseData);
} catch (IOException e) {
e.printStackTrace();
}
}
}).start();
3. Retrofit
Retrofit 是一个RESTful API的Java客户端,它是一个更加高级的HTTP客户端,在Android应用中广泛使用。使用Retrofit可以方便地创建并发送HTTP请求。下面是一个使用Retrofit发送HTTP GET请求并处理响应的示例:
public interface ApiService {
@GET("api/data")
Call<Result> getData();
}
Retrofit retrofit = new Retrofit.Builder()
.baseUrl("https://www.example.com/")
.addConverterFactory(GsonConverterFactory.create())
.build();
ApiService apiService = retrofit.create(ApiService.class);
Call<Result> call = apiService.getData();
call.enqueue(new Callback<Result>() {
@Override
public void onResponse(Call<Result> call, Response<Result> response) {
Result result = response.body();
Log.d("TAG", result.toString());
}
@Override
public void onFailure(Call<Result> call, Throwable t) {
t.printStackTrace();
}
});
结语
本文介绍了Android网络编程中的三种常见方式:HttpURLConnection、OkHttp和Retrofit,并通过示例对它们进行了说明。在实际开发中,根据需要选择不同的方式来实现网络通信。
本文标题为:android 网络编程之网络通信几种方式实例分享
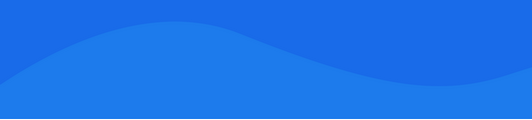
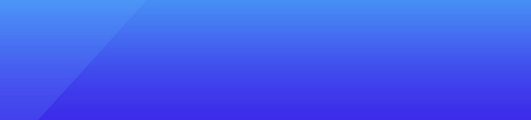
基础教程推荐
- SpringCloud hystrix断路器与全局解耦全面介绍 2023-06-23
- java – SQLSyntaxErrorException:ORA-00911:无效字符 2023-11-05
- 工作中禁止使用Executors快捷创建线程池原理详解 2023-07-01
- Java用邻接表存储图的示例代码 2023-01-18
- JSP实用教程之简易文件上传组件的实现方法(附源码) 2023-08-01
- MyBatis加解密插件的示例详解 2023-04-23
- Java利用遗传算法求解最短路径问题 2022-12-08
- SpringBoot浅析缓存机制之Redis单机缓存应用 2023-04-12
- java中random的用法小结 2022-12-12
- java – 在Oracle ucp中找不到oracle.ucp.jdbc.PoolDataSourceFactory 2023-11-07