在Java Web开发中,图形验证码是常用的用户验证工具。通过在表单中添加验证码,可以有效防止自动化机器人等非人类恶意行为的攻击。本文将详细介绍Java Web开发中,如何生成和使用图形验证码。
Java Web开发之图形验证码的生成与使用方法
在Java Web开发中,图形验证码是常用的用户验证工具。通过在表单中添加验证码,可以有效防止自动化机器人等非人类恶意行为的攻击。本文将详细介绍Java Web开发中,如何生成和使用图形验证码。
生成图形验证码
生成图形验证码需要使用Java提供的Graphics2D类。其中,需要注意以下几个关键点:
- 随机生成指定位数的验证码字符串
- 定义图形验证码图片的宽度和高度
- 绘制并设置图形的字体和颜色
- 绘制验证码字符串,并添加干扰线
- 输出图片格式并输出到客户端
下面是一个生成图形验证码图片的示例代码:
import java.awt.Color;
import java.awt.Font;
import java.awt.Graphics2D;
import java.awt.image.BufferedImage;
import java.io.IOException;
import java.util.Random;
import javax.imageio.ImageIO;
public class CaptchaGenerator {
private static final int WIDTH = 100;
private static final int HEIGHT = 40;
private static final int LENGTH = 4;
private static String generateCaptchaString() {
String source = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789";
StringBuilder sb = new StringBuilder();
Random random = new Random();
for (int i = 0; i < LENGTH; ++i) {
int index = random.nextInt(source.length());
sb.append(source.charAt(index));
}
return sb.toString();
}
public static BufferedImage generateCaptchaImage() throws IOException {
BufferedImage captchaImage = new BufferedImage(WIDTH, HEIGHT, BufferedImage.TYPE_INT_RGB);
Graphics2D graphics = captchaImage.createGraphics();
graphics.setColor(Color.LIGHT_GRAY);
graphics.fillRect(0, 0, WIDTH, HEIGHT);
Font font = new Font("Arial", Font.BOLD, 20);
graphics.setFont(font);
String captchaString = generateCaptchaString();
for (int i = 0; i < LENGTH; ++i) {
int x = 10 + i * 20;
int y = 25;
int theta = (int) (Math.random() * 45);
graphics.rotate(theta * Math.PI / 180, x, y);
graphics.setColor(getRandomColor());
graphics.drawString(String.valueOf(captchaString.charAt(i)), x, y);
graphics.rotate(-theta * Math.PI / 180, x, y);
}
Random random = new Random();
for (int i = 0; i < 4; ++i) {
int x1 = random.nextInt(WIDTH);
int y1 = random.nextInt(HEIGHT);
int x2 = random.nextInt(WIDTH);
int y2 = random.nextInt(HEIGHT);
graphics.setColor(getRandomColor());
graphics.drawLine(x1, y1, x2, y2);
}
ImageIO.write(captchaImage, "JPEG", response.getOutputStream());
graphics.dispose();
return captchaImage;
}
private static Color getRandomColor() {
Random random = new Random();
int r = random.nextInt(256);
int g = random.nextInt(256);
int b = random.nextInt(256);
return new Color(r, g, b);
}
}
在网页中使用图形验证码
在网页中使用图形验证码,需要在表单中添加一个验证码输入框,同时定义一个表示图形验证码的图片。点击验证码图片之后,可以通过AJAX请求向后台获取最新的验证码图片,从而避免使用缓存的旧图片。
以下是一个使用Java Servlet实现图形验证码功能的示例代码:
@WebServlet("/captcha")
public class CaptchaServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("image/jpeg");
response.setHeader("Cache-Control", "no-store");
response.setHeader("Pragma", "no-cache");
response.setDateHeader("Expires", 0);
BufferedImage captchaImage = CaptchaGenerator.generateCaptchaImage(response);
HttpSession session = request.getSession(true);
session.setAttribute("captcha", captchaString);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String userCaptcha = request.getParameter("captcha");
HttpSession session = request.getSession();
String captcha = (String) session.getAttribute("captcha");
if (captcha != null && captcha.equalsIgnoreCase(userCaptcha)) {
// 验证码正确
} else {
// 验证码错误
}
}
}
在表单中添加验证码输入框和图形验证码图片:
<form action="login" method="post">
<div>
<label for="username">用户名:</label>
<input type="text" id="username" name="username">
</div>
<div>
<label for="password">密码:</label>
<input type="password" id="password" name="password">
</div>
<div>
<label for="captcha">验证码:</label>
<input type="text" id="captcha" name="captcha">
<img id="captchaImage" src="captcha" alt="验证码">
<a href="#" onclick="javascript:document.getElementById('captchaImage').src='captcha?' + Math.random();">看不清?换一个</a>
</div>
<div>
<input type="submit" value="登录">
</div>
</form>
在上述表单中,当用户点击验证码图片时,会通过JavaScript生成一个新的URL,从而向服务器请求最新的验证码图片。
以上即为Java Web开发中图形验证码的生成和使用方法的完整攻略,并附带两个示例说明。
本文标题为:Java Web开发之图形验证码的生成与使用方法
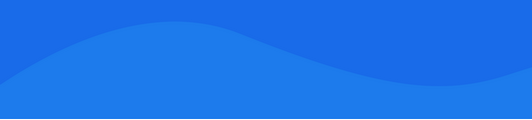
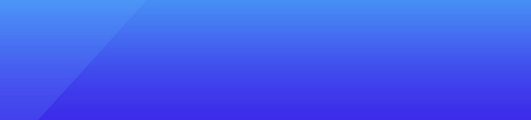
基础教程推荐
- 详解JDBC使用 2023-12-15
- java – 从另一个线程取消MySQL查询执行 2023-11-06
- Java InheritableThreadLocal使用示例详解 2023-06-01
- SpringBoot项目多数据源及mybatis 驼峰失效的问题解决方法 2023-02-19
- java实现简易聊天功能 2023-05-19
- jsp实现Servlet文件下载的方法 2023-08-02
- 关于报错IDEA Terminated with exit code 1的解决方法 2023-04-17
- springboot vue测试前端项目管理列表分页功能实现 2022-11-16
- Spring Cloud oauth2 认证服务搭建过程示例 2023-02-05
- 在Java函数中执行SQL语句 2023-11-09