介绍SpringMVC中的handlerMappings对象用法的完整攻略如下:
介绍SpringMVC中的handlerMappings对象用法的完整攻略如下:
什么是handlerMappings对象
handlerMappings对象是Spring MVC中的一个重要组件,负责将请求映射到对应的控制器处理器(handler)上。它是一个接口,定义了获取HandlerExecutionChain对象的方法。
HandlerExecutionChain对象是什么
HandlerExecutionChain对象是包装HandlerMethod对象的容器对象。它包含了处理器的所有拦截器对象,并提供了执行拦截器和执行处理器的方法。
如何使用handlerMappings对象
在SpringMVC中使用handlerMappings对象的过程如下:
第一步:在配置文件中定义handlerMappings对象
在SpringMVC的配置文件中定义handlerMappings对象。可以使用DefaultAnnotationHandlerMapping作为handlerMappings对象的实现,该实现会扫描所有带有@Controller注解的Bean,找到其中带有@RequestMapping注解的方法,并将其注册为处理请求的handler。
例如,在SpringMVC配置文件中进行如下配置:
<bean class="org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerMapping"/>
第二步:将handlerMappings对象注入到DispatcherServlet中
在DispatcherServlet中定义一个handlerMappings属性,并添加@Autowired注解注入handlerMappings对象。
例如,在DispatcherServlet配置文件中进行如下配置:
<bean class="org.springframework.web.servlet.DispatcherServlet">
<property name="handlerMappings">
<bean class="org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerMapping"/>
</property>
</bean>
第三步:使用handlerMappings对象
通过handlerMappings对象的getHandler方法获取对应的HandlerExecutionChain对象。然后,可以使用HandlerExecutionChain对象的getInterceptors方法获取所有的拦截器对象,使用execute方法执行拦截器和处理器对象。
例如,在处理请求时可以进行如下代码编写:
HandlerExecutionChain chain = handlerMappings.getHandler(request);
Object handler = chain.getHandler();
if (handler instanceof HandlerMethod) {
HandlerMethod handlerMethod = (HandlerMethod) handler;
// 获取拦截器列表
List<Object> interceptors = chain.getInterceptors();
// 执行拦截器
for (Object interceptor : interceptors) {
((HandlerInterceptor) interceptor).preHandle(request, response, handlerMethod);
}
// 执行处理器
ModelAndView mav = handlerAdapter.handle(request, response, handlerMethod);
// 执行拦截器
for (Object interceptor : interceptors) {
((HandlerInterceptor) interceptor).postHandle(request, response, handlerMethod, mav);
}
}
示例
下面提供两个示例说明handlerMappings对象的使用:
示例一:全局拦截器
使用handlerMappings对象定义一个全局拦截器,拦截所有请求,并在拦截前后输出日志。
- 在SpringMVC配置文件中定义handlerMappings对象
<bean class="org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerMapping"/>
- 将handlerMappings对象注入到DispatcherServlet中
<bean class="org.springframework.web.servlet.DispatcherServlet">
<property name="handlerMappings">
<bean class="org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerMapping"/>
</property>
<property name="interceptors">
<list>
<bean class="com.example.interceptor.LogInterceptor"/>
</list>
</property>
</bean>
- 定义一个全局拦截器
public class LogInterceptor implements HandlerInterceptor {
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception {
System.out.println("before handling request");
return true;
}
public void postHandle(HttpServletRequest request, HttpServletResponse response, Object handler,
ModelAndView modelAndView) throws Exception {
System.out.println("after handling request");
}
}
示例二:动态添加handlerMappings对象
动态添加一些handlerMappings对象,将不同的请求映射到不同的处理器上。
- 创建handlerMappings对象
public class TestHandlerMapping extends AbstractUrlHandlerMapping {
private Map<String, Object> handlerMap;
public TestHandlerMapping() {
handlerMap = new HashMap<>();
handlerMap.put("/test1", new Test1Controller());
handlerMap.put("/test2", new Test2Controller());
}
protected Object getHandlerInternal(HttpServletRequest request) throws Exception {
String requestUri = getUrlPathHelper().getLookupPathForRequest(request);
return handlerMap.get(requestUri);
}
}
- 在SpringMVC配置文件中动态添加handlerMappings对象
<bean class="org.springframework.beans.factory.config.MethodInvokingFactoryBean">
<property name="targetObject">
<bean class="org.springframework.web.servlet.DispatcherServlet"
init-method="initServletBean">
<property name="handlerMappings">
<list>
<bean class="com.example.handler.TestHandlerMapping"/>
</list>
</property>
</bean>
</property>
<property name="targetMethod" value="onRefresh"/>
</bean>
这个示例中,我们动态创建了一个TestHandlerMapping对象,并将其添加到DispatcherServlet的handlerMappings列表中。该TestHandlerMapping对象会根据请求的URI动态选择一个处理器进行处理。
本文标题为:SpringMVC中的handlerMappings对象用法
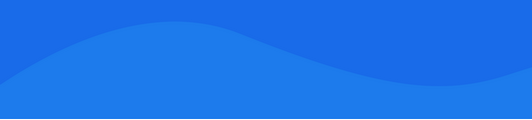
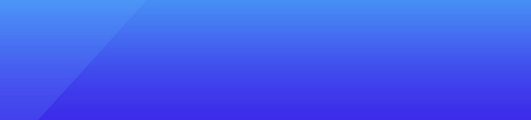
基础教程推荐
- EJB3.0开发之多对多和一对一 2024-01-10
- SpringBoot+SpringSecurity+JWT实现系统认证与授权示例 2023-04-06
- jsp的九大内置对象深入讲解 2023-07-30
- java – 服务方法的事务管理器,它对来自多个模式的表执行数据库操作 2023-11-10
- dbcp 连接池不合理的锁导致连接耗尽解决方案 2023-08-01
- Spring Boot实现文件上传下载 2023-04-12
- Java文件流的解读 2023-10-08
- java – 从另一个线程取消MySQL查询执行 2023-11-06
- Go Java算法之外观数列实现方法示例详解 2023-04-07
- Java 在 Array 和 Set 之间进行转换的示例 2023-07-14