接下来我将为你讲解“详解SpringMVC重定向传参数的实现”的完整攻略。
接下来我将为你讲解“详解SpringMVC重定向传参数的实现”的完整攻略。
标题
介绍
在SpringMVC中,有时候需要在重定向跳转的时候把一些参数传递过去,以便在下一个请求中使用。本文将详细讲解如何在SpringMVC中实现重定向传参数。
实现步骤
第一步:使用RedirectAttributes
添加Flash属性
SpringMVC提供了RedirectAttributes
类用于在重定向请求中添加Flash属性。Flash属性可以在多个请求之间共享,直到被取出后被删除。这意味着我们可以在跳转到另一个页面之前设置Flash属性,然后在另一个页面中取出这些属性。下面是一个示例:
@GetMapping("/redirectWithFlashAttributes")
public String redirectWithFlashAttributes(RedirectAttributes ra) {
ra.addFlashAttribute("message", "Redirect with Flash attributes");
return "redirect:/showMessage";
}
在上面的示例中,我们添加一个名为message
的Flash属性,并在跳转到/showMessage
请求时自动包含在重定向请求中。
第二步:使用@RequestParam传递参数
如果我们需要传递一些非Flash属性,比如表单中的字段,我们可以使用@RequestParam注解在URL中传递它们。下面是一个示例:
@GetMapping("/redirectWithParams")
public String redirectWithParams(@RequestParam("username") String username,
@RequestParam("password") String password,
RedirectAttributes ra) {
ra.addAttribute("username", username);
ra.addAttribute("password", password);
return "redirect:/showMessage";
}
在上面的示例中,我们使用@RequestParam
注解来声明我们需要传递的参数。然后,我们把这些参数添加到RedirectAttributes
对象中,并在重定向请求中自动包含它们。
第三步:在重定向请求中取出参数
在下一个请求中,我们需要从重定向请求中获取参数。这可以通过@ModelAttribute
注解来实现。下面是一个示例:
@GetMapping("/showMessage")
public String showMessage(@ModelAttribute("message") String message,
@ModelAttribute("username") String username,
@ModelAttribute("password") String password,
Model model) {
System.out.println(message);
System.out.println(username);
System.out.println(password);
model.addAttribute("message", message);
return "showMessage";
}
在上面的示例中,我们通过@ModelAttribute
注解声明需要从请求中取出的参数,并将它们添加到Model对象中,以便在页面中使用。
示例说明
示例一
假设我们有一个登录页面,用户需要输入用户名和密码才能登录。当用户登录成功后,我们想要重定向到一个显示成功信息的页面,并在页面中显示用户输入的用户名。下面是一个示例:
@PostMapping("/login")
public String login(@RequestParam("username") String username,
@RequestParam("password") String password,
RedirectAttributes ra) {
// 假设验证成功
ra.addAttribute("username", username);
ra.addFlashAttribute("message", "登录成功");
return "redirect:/showMessage";
}
@GetMapping("/showMessage")
public String showMessage(@ModelAttribute("message") String message,
@ModelAttribute("username") String username,
Model model) {
model.addAttribute("message", message);
model.addAttribute("username", username);
return "showMessage";
}
在上面的示例中,我们使用@RequestParam
注解来获取用户名和密码,并在验证成功后将用户名添加到RedirectAttributes
中,然后使用addFlashAttribute
方法向Flash属性中添加消息。然后,我们将重定向到/showMessage
请求,该请求将从Flash属性中读取消息,并从重定向请求中读取用户名,并在页面中显示它们。
示例二
假设我们有一个用户信息编辑页面,用户可以输入姓名、邮件地址和电话号码。当用户提交表单时,我们想要重定向到另一个页面,并在页面上显示成功消息以及已输入的用户信息。下面是一个示例:
@PostMapping("/editUser")
public String editUser(User user, RedirectAttributes ra) {
// 更新用户信息到数据库
ra.addFlashAttribute("message", "用户信息已更新");
ra.addAttribute("name", user.getName());
ra.addAttribute("email", user.getEmail());
ra.addAttribute("phone", user.getPhone());
return "redirect:/showUser";
}
@GetMapping("/showUser")
public String showUser(@ModelAttribute("message") String message,
@ModelAttribute("name") String name,
@ModelAttribute("email") String email,
@ModelAttribute("phone") String phone,
Model model) {
model.addAttribute("message", message);
model.addAttribute("name", name);
model.addAttribute("email", email);
model.addAttribute("phone", phone);
return "showUser";
}
在上面的示例中,我们使用传递的User
对象更新用户信息,然后向RedirectAttributes
对象中添加Flash属性和用户名、邮箱和电话号码的值。然后,我们将重定向到/showUser
请求,该请求将从Flash属性中读取消息,并从重定向请求中读取用户信息,并在页面中显示它们。
总结
以上就是如何在SpringMVC中实现重定向传参数的完整攻略。我们可以使用RedirectAttributes
添加Flash属性,并使用@RequestParam
注解从表单中获取参数,然后通过@ModelAttribute
注解从重定向请求中获取参数。希望能对你有所帮助!
本文标题为:详解SpringMVC重定向传参数的实现
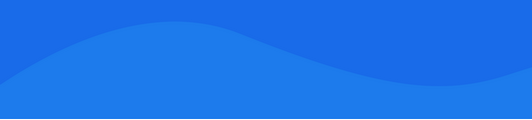
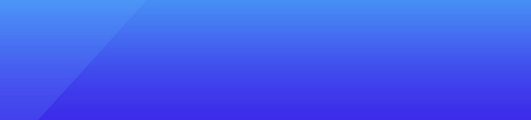
基础教程推荐
- Springboot详解整合SpringSecurity实现全过程 2023-03-21
- Java实现规则几何图形的绘制与周长面积计算详解 2023-02-28
- Spring基于注解整合Redis完整实例 2024-02-26
- Java实现空指针后的猜拳游戏 2023-05-19
- 解决SpringAop内部调用时不经过代理类的问题 2023-08-10
- mongodb java驱动程序聚合组 2023-11-10
- 使用SpringBoot+EasyExcel+Vue实现excel表格的导入和导出详解 2023-04-23
- Java中this和super关键字的使用详解 2023-06-10
- JSP使用ajaxFileUpload.js实现跨域问题 2023-08-02
- Spring Boot 的创建和运行示例代码详解 2023-03-15