下面是关于 Java 使用 HttpClient 发送 POST 请求的完整攻略。
下面是关于 Java 使用 HttpClient 发送 POST 请求的完整攻略。
组件
在 Java 中发送 HTTP 请求,我们可以使用 Apache 的 HttpClient 组件,它提供了一系列的 API 帮助我们创建和发送请求。
在使用 HttpClient 组件之前,需要下载 HttpClient 组件的 jar 包,并将其添加到项目依赖中。
POST 请求
设置请求参数
发送 POST 请求需要设置请求参数,我们可以通过创建 Map 对象或者使用其他方式来设置请求参数,下面是以创建 Map 对象来设置请求参数的示例代码:
Map<String, String> params = new HashMap<>();
params.put("name", "张三");
params.put("age", "18");
params.put("sex", "男");
创建HttpPost对象
创建 HttpPost 对象,用于设置请求地址和请求参数:
HttpPost post = new HttpPost("http://localhost:8080/api/user");
post.setEntity(new UrlEncodedFormEntity(params, Charset.forName("UTF-8")));
发送请求并获取响应
使用 HttpClient 组件发送 HTTP 请求,然后获取响应结果:
CloseableHttpClient client = HttpClients.createDefault();
CloseableHttpResponse response = client.execute(post);
int statusCode = response.getStatusLine().getStatusCode();
if (statusCode == HttpStatus.SC_OK) {
HttpEntity entity = response.getEntity();
String result = EntityUtils.toString(entity, Charset.forName("UTF-8"));
System.out.println(result);
}
示例
下面我们来看一下两个发送 POST 请求的示例。
示例1
发送 JSON 格式的 POST 请求:
CloseableHttpClient client = HttpClients.createDefault();
HttpPost post = new HttpPost("http://localhost:8080/api/user");
Map<String, Object> jsonMap = new HashMap<>();
jsonMap.put("name", "张三");
jsonMap.put("age", "18");
jsonMap.put("sex", "男");
String jsonString = JSON.toJSONString(jsonMap);
StringEntity entity = new StringEntity(jsonString, ContentType.APPLICATION_JSON);
post.setEntity(entity);
CloseableHttpResponse response = client.execute(post);
int statusCode = response.getStatusLine().getStatusCode();
if (statusCode == HttpStatus.SC_OK) {
HttpEntity httpEntity = response.getEntity();
String result = EntityUtils.toString(httpEntity, Charset.forName("UTF-8"));
System.out.println(result);
}
示例2
发送 Form 格式的 POST 请求:
CloseableHttpClient client = HttpClients.createDefault();
HttpPost post = new HttpPost("http://localhost:8080/api/user");
Map<String, String> params = new HashMap<>();
params.put("name", "张三");
params.put("age", "18");
params.put("sex", "男");
post.setEntity(new UrlEncodedFormEntity(params, Charset.forName("UTF-8")));
CloseableHttpResponse response = client.execute(post);
int statusCode = response.getStatusLine().getStatusCode();
if (statusCode == HttpStatus.SC_OK) {
HttpEntity httpEntity = response.getEntity();
String result = EntityUtils.toString(httpEntity, Charset.forName("UTF-8"));
System.out.println(result);
}
以上就是 Java 使用 HttpClient 发送 POST 请求的完整攻略和两个示例。
沃梦达教程
本文标题为:java使用httpclient发送post请求示例
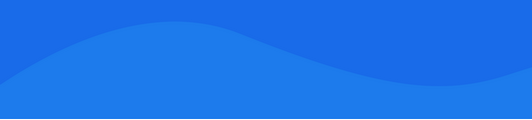
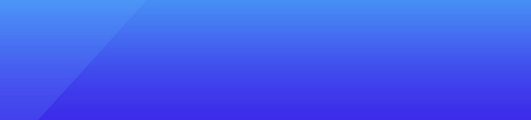
基础教程推荐
猜你喜欢
- 从JAVA应用程序使用SSL连接到MongoDb 2023-11-06
- Tree组件实现支持50W数据方法剖析 2023-04-07
- 一文搞懂Java项目中枚举的定义与使用 2023-01-12
- Java实现折半插入排序算法的示例代码 2023-04-12
- mybatis-plus 如何配置逻辑删除 2023-02-19
- JQuery ztree 异步加载实例讲解 2024-02-27
- JSP 多条SQL语句同时执行的方法 2024-01-11
- Java设计模式中的门面模式详解 2023-05-25
- Java装饰者模式的示例详解 2022-11-05
- Synchronized 和 ReentrantLock 的实现原理及区别 2023-05-19