下面是关于“springboot 返回json格式数据时间格式配置方式”的完整攻略。
下面是关于“springboot 返回json格式数据时间格式配置方式”的完整攻略。
一、需求分析
在Spring Boot应用中,我们常常需要返回JSON格式数据。而JSON格式中的时间字段经常需要进行格式化,以便更加直观和易读。因此,我们需要对返回的时间字段进行格式化处理。
二、解决方法
Spring Boot提供了多种方式来解决这个问题,下面将介绍两种不同的方法。
1. 使用注解@JsonFormat
使用注解@JsonFormat,可以对Java对象(包含时间类型字段)进行自定义格式化输出。
具体实现方式:
-
添加注解 @JsonFormat 在属性字段上
```java
import com.fasterxml.jackson.annotation.JsonFormat;import java.util.Date;
public class User {
private Integer id; private String name; @JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss", timezone = "GMT+8") private Date createTime; // getter 和 setter 方法省略
}
``` -
在controller中返回包含User的json对象
```java
@RestController
@RequestMapping("user")
public class UserController {@RequestMapping(value = "/{id}", method = RequestMethod.GET) public User getUser(@PathVariable Integer id) { User user = new User(); user.setId(id); user.setName("Jack"); user.setCreateTime(new Date(System.currentTimeMillis())); return user; }
}
``` -
返回结果
{
"id": 1,
"name": "Jack",
"createTime": "2021-06-08 17:04:45"
}
2. 配置SpringBoot默认的Jackson ObjectMapper
通过配置SpringBoot默认的Jackson ObjectMapper,可以实现对所有时间类型字段的格式化输出。
具体实现方式:
-
在Spring配置文件(application.yml或application.properties)中添加以下配置
yml
spring:
jackson:
date-format: yyyy-MM-dd HH:mm:ss
time-zone: GMT+8 -
在controller中返回包含User的json对象
```java
@RestController
@RequestMapping("user")
public class UserController {@RequestMapping(value = "/{id}", method = RequestMethod.GET) public User getUser(@PathVariable Integer id) { User user = new User(); user.setId(id); user.setName("Jack"); user.setCreateTime(new Date(System.currentTimeMillis())); return user; }
}
``` -
返回结果
{
"id": 1,
"name": "Jack",
"createTime": "2021-06-08 17:04:45"
}
三、总结
以上两种方法都可以实现对Spring Boot应用返回JSON格式数据中的时间类型字段进行格式化处理。具体使用哪种方式取决于实际场景和需求。
四、参考链接
- Spring Boot官方文档
- 关于Spring Boot修改默认时间格式的三种方法
本文标题为:springboot 返回json格式数据时间格式配置方式
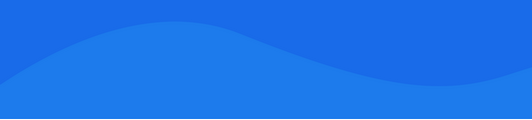
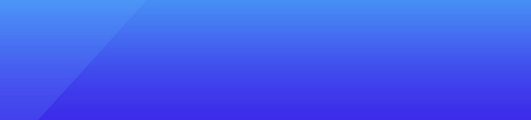
基础教程推荐
- Java通过word模板实现创建word文档报告 2023-05-19
- SpringSecurity自定义登录界面 2023-05-14
- springboot集成redis存对象乱码的问题及解决 2023-01-13
- 使用java基于pushlet和bootstrap实现的简单聊天室 2023-12-17
- JSP 开发之THE SERVLET NAME ALREADY EXISTS.解决方法 2023-07-30
- SpringCloud集成Sleuth和Zipkin的思路讲解 2023-07-01
- JavaMail实现带附件的邮件发送 2023-04-12
- SpringBoot浅析安全管理之OAuth2框架 2023-04-12
- Spring Boot整合ELK实现日志采集与监控 2023-01-24
- SpringBoot接口如何对参数进行校验 2023-02-28