下面是关于SpringBoot读取资源目录中JSON文件的方法实例的完整攻略:
下面是关于"SpringBoot读取资源目录中JSON文件的方法实例"的完整攻略:
1.准备工作
首先需要在Spring Boot项目中创建一个资源目录,在其中添加一个JSON文件。
例如,在src/main/resources目录下创建json目录,然后在json目录下创建example.json文件,如下图所示:
src/main/resources/json/example.json
2. 读取JSON文件
读取资源目录中的JSON文件
在Spring Boot项目中,可以使用ClassLoader来读取资源目录中的JSON文件。ClassLoader负责加载Java类、资源等文件,主要有以下两种方式:
1.使用自带的ClassLoader#getResourceAsStream方法,如下所示:
InputStream inputStream = getClass().getClassLoader().getResourceAsStream("json/example.json");
2.使用Spring Boot的ResourceLoader,如下所示:
@Service
public class JsonReadService {
@Autowired
private ResourceLoader resourceLoader;
public String readJsonFile(String fileName) throws IOException {
Resource resource = resourceLoader.getResource("classpath:json/" + fileName);
InputStream inputStream = resource.getInputStream();
byte[] b = new byte[inputStream.available()];
inputStream.read(b);
return new String(b, StandardCharsets.UTF_8);
}
}
使用ResourceLoader可以更方便地读取资源目录中的文件。
将JSON文件转换为Java对象
将JSON文件转换为Java对象需要使用第三方库,例如Jackson或Gson。下面以Jackson为例,演示将JSON文件转换为Java对象:
- 添加依赖:
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.8.11.1</version>
</dependency>
- 创建Java对象,例如:
public class Example {
private String name;
private int age;
// getter/setter
}
- 读取并转换JSON文件:
ObjectMapper mapper = new ObjectMapper();
Example example = mapper.readValue(inputStream, Example.class);
其中,输入流inputStream来自于上面提到的读取JSON文件的方法。
示例
下面是两条示例说明:
示例1:读取并输出JSON文件的内容
读取并输出example.json文件的内容,如下所示:
@Service
public class JsonReadService {
@Autowired
private ResourceLoader resourceLoader;
public String readJsonFile(String fileName) throws IOException {
Resource resource = resourceLoader.getResource("classpath:json/" + fileName);
InputStream inputStream = resource.getInputStream();
byte[] b = new byte[inputStream.available()];
inputStream.read(b);
return new String(b, StandardCharsets.UTF_8);
}
}
调用readJsonFile方法来读取example.json文件的内容:
@Autowired
private JsonReadService jsonReadService;
@GetMapping("/json")
public String getJsonContent() throws IOException {
String content = jsonReadService.readJsonFile("example.json");
return content;
}
示例2:将JSON文件转换为Java对象
读取example.json文件,并将其转换为Example对象,如下所示:
@Service
public class JsonReadService {
@Autowired
private ResourceLoader resourceLoader;
public Example readJsonFile(String fileName) throws IOException {
ObjectMapper mapper = new ObjectMapper();
Resource resource = resourceLoader.getResource("classpath:json/" + fileName);
InputStream inputStream = resource.getInputStream();
Example example = mapper.readValue(inputStream, Example.class);
return example;
}
}
调用readJsonFile方法来读取example.json文件,并将其转换为Example对象:
@Autowired
private JsonReadService jsonReadService;
@GetMapping("/json")
public Example getJsonContent() throws IOException {
Example example = jsonReadService.readJsonFile("example.json");
return example;
}
以上就是SpringBoot读取资源目录中JSON文件的方法实例的完整攻略了。
本文标题为:SpringBoot读取资源目录中JSON文件的方法实例
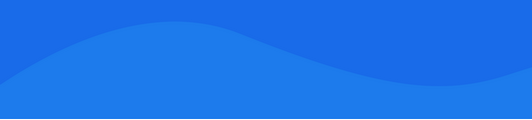
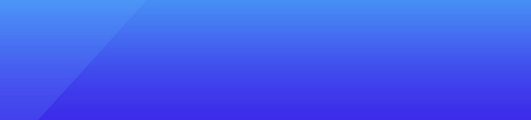
基础教程推荐
- Spring的Model 和 Map的原理源码解析 2023-01-13
- Java中Socket用法详解 2023-08-08
- 基于Springboot一个注解搞定数据字典的实践方案 2022-12-27
- 使用JDBC工具类实现简单的登录管理系统 2022-10-30
- MyBatis加解密插件的示例详解 2023-04-23
- Classloader隔离技术在业务监控中的应用详解 2023-04-23
- 关于Mysql的四种存储引擎 2023-07-15
- mybatis-plus 插入修改配置默认值的实现方式 2023-02-19
- 详解Java深拷贝,浅拷贝和Cloneable接口 2023-04-23
- 深入浅出探索Java分布式锁原理 2022-10-24