在JavaScript中使用this是一个常见的问题,它可以在不同的情况下指向不同的变量。因此,在编写JavaScript代码时,正确地理解并使用this非常重要。
JavaScript中this的用法实践分析
在JavaScript中使用this
是一个常见的问题,它可以在不同的情况下指向不同的变量。因此,在编写JavaScript代码时,正确地理解并使用this
非常重要。
什么是this
this
是一个关键字,它表示当前执行代码的对象。但它不是常规的变量,而是在函数被调用时才被赋值。也就是说,this
关键字在程序运行时才有定义。
指向全局对象
当在全局作用域中使用this
时,它会返回全局对象。在浏览器中,可以使用window
来引用全局对象。
console.log(this === window); // true
指向当前对象
当在一个函数中使用this
时,它将会指向调用该函数的对象。
var obj = {
message: "Hello, world!",
greet: function() {
console.log(this.message);
}
}
obj.greet(); // "Hello, world!"
在该例中,this
指向了obj
对象。因此,this.message
就相当于是在调用obj.message
属性。
指向函数对象
如果一个函数不绑定到任何对象上,那么它的this
就会指向全局对象。
function test() {
console.log(this === window);
}
test(); // true
显示设置this的值
函数的call()
和apply()
方法可以用来显式地设置this
的值。call()
和apply()
方法都会立即调用函数,并将函数的上下文设置为this
的值。
var person1 = { name: "Alice" };
var person2 = { name: "Bob" };
function greet() {
console.log("Hello, " + this.name + "!");
}
greet.call(person1); // "Hello, Alice!"
greet.call(person2); // "Hello, Bob!"
在该例中,我们将greet()
函数绑定到了person1
和person2
对象上,这样在调用greet()
函数时,它的this
就会指向相应的对象。
结论
this
的指向在不同的情况下有不同的值,因此在编写JavaScript代码时,正确地理解并使用this
非常重要。在函数中使用this
,它将会指向调用该函数的对象;在全局作用域中使用this
,它将会指向全局对象;如果一个函数不绑定到任何对象上,那么它的this
就会指向全局对象。而通过函数的call()
和apply()
方法可以用来显式地设置this
的值,在JavaScript中实现更加灵活、高效的编程。
示例1:使用箭头函数与bind方法
function Person(name) {
this.name = name;
}
Person.prototype.sayName = function () {
console.log(this.name);
};
const person1 = new Person('Alice');
const person2 = new Person('Bob');
const sayName1 = person1.sayName;
sayName1(); // undefined
const sayName2 = person1.sayName.bind(person2);
sayName2(); // "Bob"
const sayName3 = () => {
console.log(this.name);
};
person1.sayName = sayName3.bind(person2);
person1.sayName(); // "Bob"
在该例中,我们定义了一个Person
函数和它的原型方法sayName
。当我们对sayName
方法进行赋值时,由于this
的指向未被改变,所以当我们调用该方法时,其输出值为undefined
。而如果我们使用bind
方法将其绑定到person2
对象后,再次调用输出结果就为"Bob"
。如果我们使用箭头函数定义sayName3
方法,并将其绑定到person2
对象之后,再次调用person1.sayName()
方法,将输出值为"Bob"
。
示例2:使用类
class Person {
constructor(name) {
this.name = name;
}
sayName() {
console.log(this.name);
}
}
const person1 = new Person('Alice');
const person2 = new Person('Bob');
person1.sayName(); // "Alice"
person2.sayName(); // "Bob"
const sayName1 = person1.sayName;
sayName1(); // "undefined"
const sayName2 = person1.sayName.bind(person2);
sayName2(); // "Bob"
const sayName3 = () => {
console.log(this.name);
};
person1.sayName = sayName3.bind(person2);
person1.sayName(); // "Bob"
在该例中,我们定义了一个Person
类,通过构造函数赋值初始化实例的名称,再定义了一个类方法sayName
。当我们创建两个Person
类实例后调用各自的sayName
方法,可以分别输出其设置值。而在定义过程中使用bind
方法或箭头函数绑定方法,这些会取改变this
的指向,进而改变其输出值。
本文标题为:javascript中this的用法实践分析
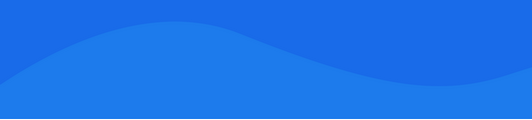
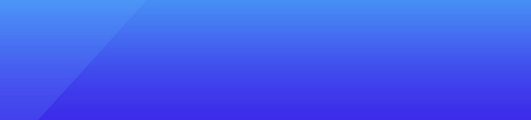
基础教程推荐
- swagger中如何给请求添加header 2023-01-02
- Clojure 与Java对比少数据结构多函数胜过多个单独类的优点 2022-12-16
- java – 如何在发生异常后使用PostgreSQL在Spring Boot中继续事务? 2023-11-10
- JSP监听器用法分析 2023-08-02
- servlet中session简介和使用例子 2024-02-25
- Spring Mvc下实现以文件流方式下载文件的方法示例 2024-02-27
- 快速学习JavaWeb中监听器(Listener)的使用方法 2024-02-25
- 教你如何在 javadoc 输出<> 符号 2023-07-14
- Java定时器Timer的源码分析 2023-07-01
- Java利用多线程复制文件 2023-05-19