让我们来详细讲解一下“SpringMVC中controller返回json数据的方法”的完整攻略。
让我们来详细讲解一下“SpringMVC中controller返回json数据的方法”的完整攻略。
1.确保项目中已经引入SpringMVC相关的依赖
在使用SpringMVC返回json数据之前,需要确保项目中已经引入SpringMVC相关的依赖。通常情况下,这些依赖可以在pom.xml文件中找到。具体的依赖包括:spring-web、spring-webmvc、jackson-databind等。
2.在Controller中定义返回值类型为@ResponseBody的方法
在Controller中定义一个处理请求的方法,并且设置返回值类型为@ResponseBody。这个注解的作用是将方法的返回值转换为json格式,并将其写入Http响应体中。例如:
@GetMapping("/user/{id}")
@ResponseBody
public User getUserById(@PathVariable("id") Long id) {
return userService.getUserById(id);
}
上述代码中,我们定义了一个@GetMapping请求映射器,用于处理“/user/{id}”请求。同时,我们将该方法的返回值类型设置为User类型,并且添加了@ResponseBody注解,以确保返回的User对象可以被转换为json格式。
3.使用MappingJackson2HttpMessageConverter类进行序列化
SpringMVC在将Java对象序列化为json对象的过程中,会使用MessageConverter进行转换,其中MappingJackson2HttpMessageConverter是默认的转换类。因此,在确保引入了jackson-databind依赖包后,我们可以直接在Controller中使用该类来将Java对象序列化为json格式。例如:
@GetMapping("/users")
public ResponseEntity<List<User>> getAllUsers() {
List<User> userList = userService.getAllUsers();
HttpHeaders headers = new HttpHeaders();
headers.add("Content-Type", "application/json; charset=UTF-8");
return new ResponseEntity<>(userList, headers, HttpStatus.OK);
}
在上述代码中,我们定义了一个@GetMapping请求映射器,用于处理“/users”请求。然后,我们从userService获取了一个List
示例
下面提供两个示例:
1.如何在Controller中返回一个json对象:
@GetMapping("/user/{id}")
@ResponseBody
public User getUserById(@PathVariable("id") Long id) {
return userService.getUserById(id);
}
2.如何在Controller中返回一个json数组:
@GetMapping("/users")
public ResponseEntity<List<User>> getAllUsers() {
List<User> userList = userService.getAllUsers();
HttpHeaders headers = new HttpHeaders();
headers.add("Content-Type", "application/json; charset=UTF-8");
return new ResponseEntity<>(userList, headers, HttpStatus.OK);
}
以上就是关于“SpringMVC中controller返回json数据的方法”的完整攻略,希望对你有所帮助。
本文标题为:SpringMVC中controller返回json数据的方法
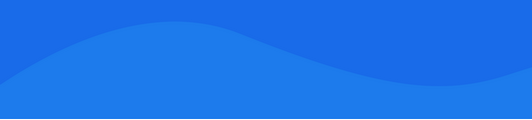
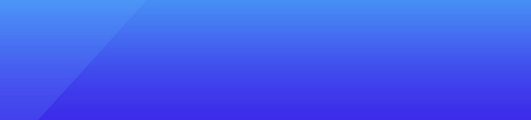
基础教程推荐
- Java ScheduledExecutorService的具体使用 2023-07-15
- SpringBoot自定义对象参数实现自动类型转换与格式化 2023-06-05
- Spring配置文件的拆分和整合过程分析 2023-06-06
- 理解Java Map的forEach方法 2023-10-08
- SpringBoot概述及在idea中创建方式 2023-05-19
- Java开发利器之Guava Cache的使用教程 2023-05-24
- springboot启动后和停止前执行方法示例详解 2023-05-08
- JavaWeb Hibernate使用全面介绍 2023-06-24
- jsp中自定义标签用法实例分析 2024-01-11
- 图解Java中归并排序算法的原理与实现 2023-04-23