为了实现Java程序发送POST请求,需要使用Java API中的HttpURLConnection类。具体的步骤如下:
为了实现Java程序发送POST请求,需要使用Java API中的HttpURLConnection类。具体的步骤如下:
1.获取HttpURLConnection对象
HttpURLConnection是Java中实现HTTP协议的常用类。利用URL.openConnection()方法可以获取HttpURLConnection对象。
URL url = new URL("http://www.example.com/resource");
HttpURLConnection connection = (HttpURLConnection)url.openConnection();
2.设置请求方法和头部参数
利用HttpURLConnection.setRequestMethod()可以设置HTTP请求的方法,如POST、GET、PUT等。同时可以设置头部参数,如Content-Type、Authorization等。
connection.setRequestMethod("POST");
connection.setRequestProperty("Content-Type", "application/json");
3.设置请求体内容
针对POST请求方法,需要设置请求体内容。
String requestBody = "{\"name\":\"John\", \"age\":30}";
OutputStream os = connection.getOutputStream();
os.write(requestBody.getBytes());
os.flush();
os.close();
4.获取响应
利用HttpURLConnection.getResponseCode()方法可以获取HTTP响应的状态码。如果状态码等于HttpURLConnection.HTTP_OK,则可以用InputStream获取服务器返回的响应内容。
if (connection.getResponseCode() == HttpURLConnection.HTTP_OK) {
InputStream is = connection.getInputStream();
BufferedReader reader = new BufferedReader(new InputStreamReader(is));
String line;
StringBuilder response = new StringBuilder();
while ((line = reader.readLine()) != null) {
response.append(line);
}
reader.close();
is.close();
System.out.println(response.toString());
}
下面是两个不同的使用HTTP POST请求发送JSON数据的示例:
示例1:使用java.net.HttpURLConnection类
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
public class Example1 {
public static void main(String[] args) throws IOException {
URL url = new URL("http://www.example.com/resource");
HttpURLConnection connection = (HttpURLConnection)url.openConnection();
connection.setRequestMethod("POST");
connection.setRequestProperty("Content-Type", "application/json");
String requestBody = "{\"name\":\"John\", \"age\":30}";
OutputStream os = connection.getOutputStream();
os.write(requestBody.getBytes());
os.flush();
os.close();
if (connection.getResponseCode() == HttpURLConnection.HTTP_OK) {
InputStream is = connection.getInputStream();
BufferedReader reader = new BufferedReader(new InputStreamReader(is));
String line;
StringBuilder response = new StringBuilder();
while ((line = reader.readLine()) != null) {
response.append(line);
}
reader.close();
is.close();
System.out.println(response.toString());
}
}
}
示例2:使用Apache HttpClient库
import java.io.IOException;
import org.apache.http.HttpEntity;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
public class Example2 {
public static void main(String[] args) throws ClientProtocolException, IOException {
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost("http://www.example.com/resource");
httpPost.setHeader("Content-Type", "application/json");
String requestBody = "{\"name\":\"John\", \"age\":30}";
HttpEntity httpEntity = new UrlEncodedFormEntity(requestBody);
httpPost.setEntity(httpEntity);
try (CloseableHttpResponse response = httpClient.execute(httpPost)) {
HttpEntity responseEntity = response.getEntity();
String responseString = EntityUtils.toString(responseEntity);
System.out.println(responseString);
}
}
}
以上就是Java发送POST请求的详细攻略,希望对您有所帮助。
本文标题为:Java发送post方法详解
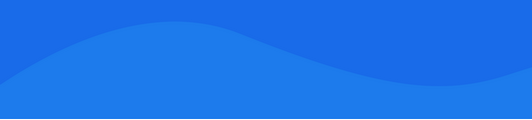
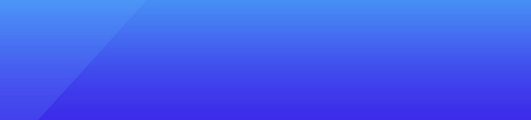
基础教程推荐
- 详解Spring Security如何在权限中使用通配符 2023-02-05
- @RequestBody注解Ajax post json List集合数据请求400/415的处理 2023-06-30
- 在js文件中如何获取basePath处理js路径问题 2024-01-10
- SpringSecurity+Redis认证过程小结 2023-08-07
- Java TCP网络通信协议详细讲解 2023-05-24
- Kotlin 标准函数和静态方法示例详解 2024-03-02
- JSP页面中如何用select标签实现级联 2024-01-12
- java – 如何从API端点按需按数据库逐个获取yield yield结果?是否需要网络套接字? 2023-11-04
- 浅谈一下Java线程组ThreadGroup 2023-07-14
- Spring Boot整合持久层之JdbcTemplate多数据源 2023-04-12