讲解SpringMvc直接接收json数据自动转化为Map的实例的完整攻略如下:
讲解SpringMvc直接接收json数据自动转化为Map的实例的完整攻略如下:
1. 添加相关依赖
首先,我们需要添加SpringMvc相关的依赖。在pom.xml文件中添加以下依赖:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>5.3.9</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.12.3</version>
</dependency>
其中,spring-web依赖包含SpringMvc的相关支持;jackson-databind包含了将JSON数据转换为Java对象(例如将JSON对象转换为Map对象)的支持。
2. 配置SpringMvc接收JSON请求
其次,我们需要在Spring配置文件中配置SpringMvc接收JSON请求。在SpringMvc的配置文件中添加以下配置:
<mvc:annotation-driven>
<mvc:message-converters>
<bean class="org.springframework.http.converter.json.MappingJackson2HttpMessageConverter"/>
</mvc:message-converters>
</mvc:annotation-driven>
其中,MappingJackson2HttpMessageConverter是将JSON数据转换为Java对象(例如将JSON对象转换为Map对象)的核心组件之一。
3. 创建Controller
我们可以通过以下方式创建Controller来处理JSON请求并将其转换为Map对象。
@RestController
@RequestMapping("/api")
public class ApiController {
@PostMapping("/test")
public Map<String, Object> test(@RequestBody Map<String, Object> param) {
// 处理Map对象
return param;
}
}
在上面的代码中,我们使用了@RestController注解来标示我们的Controller,这表明这个Controller将会返回JSON格式的数据。@PostMapping("/test")注解用于处理POST请求,并将请求的路径设置为/api/test。@RequestBody注解用于告诉SpringMvc将请求体中的JSON数据转换为Map对象。
4. 发送JSON请求
我们可以通过以下方式发送JSON请求并将其转换为Map对象:
POST /api/test HTTP/1.1
Host: localhost:8080
Content-Type: application/json;charset=UTF-8
Content-Length: 21
{"name":"tom","age":18}
在上面的请求中,我们向/api/test路径发送POST请求,并将请求体设置为JSON格式的数据。请求体中包含一个键值对(name:tom,age:18),它将会被转换为一个Map对象。
示例
我们通过两个示例来演示SpringMvc直接接收JSON数据自动转换为Map的实例。
示例1:处理JSON请求并返回结果
我们可以在SpringMvc中创建一个Controller,该Controller将接收POST请求,该请求将包含JSON数据。在Controller中,我们将转换JSON数据为Map对象并进行处理,并将结果以JSON格式返回。
@RestController
@RequestMapping("/api")
public class ApiController {
@PostMapping("/test")
public Map<String, Object> test(@RequestBody Map<String, Object> param) {
// 处理Map对象
Map<String, Object> result = new HashMap<>();
result.put("code", "200");
result.put("message", "success");
result.put("data", param);
return result;
}
}
现在,我们可以使用Postman或者其他HTTP客户端来发送一个POST请求,请求的JSON数据为:
{
"name": "Tom",
"age": 18
}
请求的URL为:http://localhost:8080/api/test
发送请求后,我们将会在返回结果中看到JSON数据,其中包含请求中的JSON数据以及我们处理后的结果:
{
"code": "200",
"message": "success",
"data": {
"name": "Tom",
"age": 18
}
}
示例2:处理JSON请求并写入数据库
我们可以在SpringMvc中创建一个Controller,该Controller将接收POST请求,该请求将包含JSON数据。在Controller中,我们将转换JSON数据为Map对象并进行处理,并将数据写入数据库中。
@RestController
@RequestMapping("/api")
public class ApiController {
@Autowired
private UserService userService;
@PostMapping("/user")
public Map<String, Object> createUser(@RequestBody Map<String, Object> param) {
// 将Map对象转换为User对象并写入数据库
User user = new User();
user.setName((String)param.get("name"));
user.setAge((Integer)param.get("age"));
userService.save(user);
Map<String, Object> result = new HashMap<>();
result.put("code", "200");
result.put("message", "success");
result.put("data", user);
return result;
}
}
现在,我们可以使用Postman或者其他HTTP客户端来发送一个POST请求,请求的JSON数据为:
{
"name": "Tom",
"age": 18
}
请求的URL为:http://localhost:8080/api/user
发送请求后,我们将会在返回结果中看到JSON数据,其中包含我们写入数据库的记录。此外,我们也可以通过查询数据库来验证数据是否成功写入。
{
"code": "200",
"message": "success",
"data": {
"id": 1,
"name": "Tom",
"age": 18,
"create_time": "2021-08-03T17:21:00.000+00:00"
}
}
本文标题为:SpringMvc直接接收json数据自动转化为Map的实例
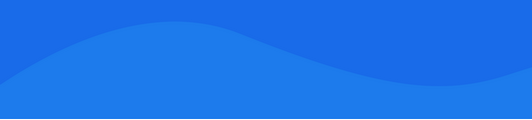
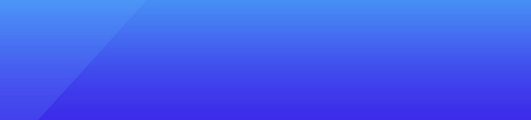
基础教程推荐
- java – 使用Hibernate 5的方言SQLite 3的问题 2023-11-10
- js动态创建标签示例代码 2024-02-25
- Java钩子方法概念原理详解 2024-03-03
- Spring5新功能日志框架Log4j2整合示例 2022-11-25
- Java函数式编程用法介绍 2023-10-08
- Java8 Lamdba函数式推导 2022-09-03
- Java查看和修改线程优先级操作详解 2022-09-03
- 解决@JsonInclude(JsonInclude.Include.NON_NULL)不起作用问题 2023-01-09
- angular实现input输入监听的示例 2023-12-16
- java实现在原有日期时间上加几个月或几天 2023-06-23