HttpClient是Apache下的一个开源项目,用于模拟浏览器请求,支持协议如下:HTTP、HTTPS、FTP、LDAP、SMTP。
Httpclient模拟POST请求JSON封装表单数据的实现方法
什么是Httpclient?
HttpClient是Apache下的一个开源项目,用于模拟浏览器请求,支持协议如下:HTTP、HTTPS、FTP、LDAP、SMTP。
为什么使用Httpclient模拟POST请求JSON封装表单数据?
Httpclient模拟POST请求JSON封装表单数据,是一种请求方式,主要用于与服务端进行数据交互,使数据传输更安全、更高效。
Httpclient模拟POST请求JSON封装表单数据的实现步骤
- 创建HttpClient对象
在Java中创建HttpClient对象,可以使用HttpClients类的createDefault()方法。
CloseableHttpClient httpClient = HttpClients.createDefault();
- 创建HttpPost对象
创建HttpPost对象,同时指定要请求的URL地址。
HttpPost httpPost = new HttpPost("https://example.com/api");
- 设置请求头
设置请求头,可以添加需要的参数。
httpPost.setHeader("Content-Type", "application/json");
- 构造请求数据
构造要发送的请求数据,将需要发送的参数以JSON格式封装。
JSONObject jsonObject = new JSONObject();
jsonObject.put("username", "testuser");
jsonObject.put("password", "testpassword");
- 发送请求
将构造的请求数据添加到请求对象中,然后通过HttpClient进行请求。
StringEntity entity = new StringEntity(jsonObject.toJSONString(), "utf-8");
httpPost.setEntity(entity);
CloseableHttpResponse response = httpClient.execute(httpPost);
- 解析返回结果
当发送请求后,服务器返回响应结果,需要进行解析。可以通过response.getEntity()获取返回的实体,然后进行解析。
示例一
假设我们需要模拟API请求,发送用户名和密码。
private static void sendRequest() throws IOException {
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost("http://example.com/apis/login");
httpPost.setHeader("Content-Type", "application/json");
JSONObject jsonObject = new JSONObject();
jsonObject.put("username", "testuser");
jsonObject.put("password", "testpassword");
StringEntity entity = new StringEntity(jsonObject.toJSONString(), "utf-8");
httpPost.setEntity(entity);
CloseableHttpResponse response = httpClient.execute(httpPost);
HttpEntity responseEntity = response.getEntity();
if (responseEntity == null) {
return;
}
String result = EntityUtils.toString(responseEntity, "utf-8");
System.out.println(result);
}
示例二
另外一个场景是模拟form表单请求。
private static void sendForm() throws IOException {
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost("http://example.com/form");
List<NameValuePair> params = new ArrayList<NameValuePair>();
params.add(new BasicNameValuePair("username", "testuser"));
params.add(new BasicNameValuePair("password", "testpassword"));
UrlEncodedFormEntity entity = new UrlEncodedFormEntity(params, "utf-8");
httpPost.setEntity(entity);
CloseableHttpResponse response = httpClient.execute(httpPost);
HttpEntity responseEntity = response.getEntity();
if (responseEntity == null) {
return;
}
String result = EntityUtils.toString(responseEntity, "utf-8");
System.out.println(result);
}
以上便是使用Httpclient模拟POST请求JSON封装表单数据的实现方法,可以根据需要进行扩展。
本文标题为:httpclient模拟post请求json封装表单数据的实现方法
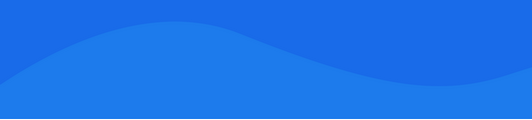
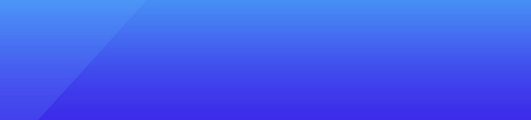
基础教程推荐
- Mybatis基础概念与高级应用小结 2023-01-02
- java使用Filter实现自动登录的方法 2024-02-25
- JSP页面传值乱码过滤方法 2023-08-03
- Java实现优先队列式广度优先搜索算法的示例代码 2022-09-03
- Spring Boot 多数据源处理事务的思路详解 2022-11-29
- java long 类型数据的赋值方式 2023-02-28
- Java 负载均衡算法作用详细解析 2023-03-07
- 利用 SpringBoot 在 ES 中实现类似连表查询功能 2023-02-28
- java – 超时更新DB2表 2023-11-10
- java – SLF4J是否可以在多线程应用程序中用于记录到数据库? 2023-11-07