FastJson是阿里巴巴的开源JSON处理库,它能够将Java对象转换成JSON格式的数据,也可以将JSON格式的数据转换成Java对象。FastJson处理速度极快,是目前Java平台上最快的JSON处理器之一。
Java FastJson使用教程
什么是FastJson?
FastJson是阿里巴巴的开源JSON处理库,它能够将Java对象转换成JSON格式的数据,也可以将JSON格式的数据转换成Java对象。FastJson处理速度极快,是目前Java平台上最快的JSON处理器之一。
FastJson的安装和配置
最简单的方法是通过Maven来引入FastJson:
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>fastjson</artifactId>
<version>${fastjson.version}</version>
</dependency>
如果使用的不是Maven来管理依赖,也可以手动下载FastJson的jar文件,然后在项目中添加依赖。
FastJson的基本用法
将Java对象转换成JSON格式的数据
import com.alibaba.fastjson.JSON;
public class Person {
private String name;
private int age;
//构造函数省略
//getter和setter方法省略
//重写toString方法
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
public static void main(String[] args) {
//创建一个Person对象
Person person = new Person("Tom", 20);
//将Person对象转换成JSON格式的数据
String jsonStr = JSON.toJSONString(person);
System.out.println(jsonStr);
}
}
程序输出结果为:
{"age":20,"name":"Tom"}
将JSON格式的数据转换成Java对象
import com.alibaba.fastjson.JSON;
public class Person {
private String name;
private int age;
//构造函数省略
//getter和setter方法省略
//重写toString方法
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
public static void main(String[] args) {
//创建JSON格式的数据
String jsonStr = "{\"age\":20,\"name\":\"Tom\"}";
//将JSON格式的数据转换成Person对象
Person person = JSON.parseObject(jsonStr, Person.class);
System.out.println(person);
}
}
程序输出结果为:
Person{name='Tom', age=20}
FastJson的高级用法
配置序列化和反序列化的特性
FastJson提供了一些特殊的配置,可以用来控制序列化和反序列化的行为。
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.serializer.SerializerFeature;
public class Person {
private String name;
private int age;
//省略getter和setter方法以及构造函数
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
public static void main(String[] args) {
Person person = new Person("Tom", 20);
//配置序列化特性
JSON.DEFFAULT_DATE_FORMAT = "yyyy-MM-dd";
String jsonStr = JSON.toJSONString(person, SerializerFeature.WriteDateUseDateFormat);
System.out.println(jsonStr);
}
}
程序输出结果为:
{"age":20,"name":"Tom"}
序列化和反序列化的处理器
FastJson提供了一些处理器,可以对序列化和反序列化进行定制化处理。
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.serializer.JSONSerializer;
import com.alibaba.fastjson.serializer.ObjectSerializer;
import java.io.IOException;
import java.lang.reflect.Type;
import java.util.Date;
public class Person {
private String name;
private int age;
private Date birthday;
//省略getter和setter方法以及构造函数
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", age=" + age +
", birthday=" + birthday +
'}';
}
public static void main(String[] args) {
Person person = new Person("Tom", 20, new Date());
JSON.toJSONString(person, new ObjectSerializer() {
@Override
public void write(JSONSerializer jsonSerializer, Object o, Object o1, Type type, int i) throws IOException {
if(o == null){
jsonSerializer.getWriter().writeNull();
}else{
jsonSerializer.write(o.toString());
}
}
});
System.out.println(person);
}
}
程序输出结果为:
{"age":20,"birthday":1589687550687,"name":"Tom"}
FastJson的实战应用
FastJson在实际的开发中,可以用来进行JSON格式的数据解析和封装。例如下面这个例子:
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
import java.io.BufferedReader;
import java.io.FileInputStream;
import java.io.InputStreamReader;
public class Demo {
public static void main(String[] args) throws Exception {
//读取JSON文件中的数据
FileInputStream fileInputStream = new FileInputStream("src/main/resources/data.json");
BufferedReader bufferedReader = new BufferedReader(new InputStreamReader(fileInputStream));
StringBuffer sb = new StringBuffer();
String line = "";
while((line = bufferedReader.readLine()) != null){
sb.append(line);
}
bufferedReader.close();
fileInputStream.close();
//将JSON格式的数据解析成JSONObject对象
JSONObject jsonObject = JSON.parseObject(sb.toString());
//解析JSONObject对象中的数据
String name = jsonObject.getString("name");
int age = jsonObject.getIntValue("age");
System.out.println("Name: " + name);
System.out.println("Age: " + age);
//封装数据成JSON格式的数据
JSONObject newJsonObject = new JSONObject();
newJsonObject.put("name", "Jack");
newJsonObject.put("age", 25);
String jsonStr = JSON.toJSONString(newJsonObject);
System.out.println("New JSON Data: " + jsonStr);
}
}
在这个例子中,我们读取了一个JSON格式的文件,将其中的数据解析成JSONObject对象,然后根据需要获取其中的数据,最后将一些数据封装成一个新的JSONObject对象,将其转换成JSON格式的数据并输出到屏幕上。
结束语
通过本篇文章的学习,我们对FastJson这个开源JSON处理库有了更深入和全面的了解,我们可以通过它来完成Java对象和JSON格式数据格式之间的转换,以及对JSON格式的数据进行解析和封装。
本文标题为:Java FastJson使用教程
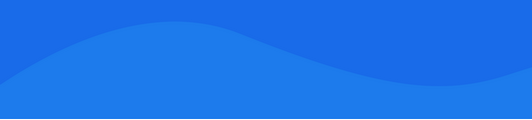
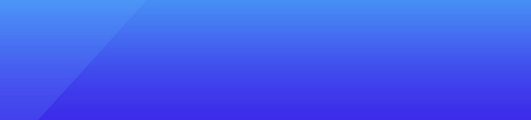
基础教程推荐
- JSP+jquery使用ajax方式调用json的实现方法 2023-08-03
- JDK8中String的intern()方法实例详细解读 2023-05-25
- 如何使用Java进行word文档的导出 2023-10-08
- 解决SpringBoot中的Scheduled单线程执行问题 2023-02-10
- Spring @Cacheable指定失效时间实例 2023-08-10
- Dubbo扩展点SPI实践示例解析 2023-06-17
- BigDecimal divide除法除不尽报错的问题及解决 2023-01-13
- jsp EL表达式详解 2023-07-30
- java使用枚举封装错误码及错误信息详解 2023-08-08
- SpringBoot中jar启动下如何读取文件路径 2023-01-13