下面是关于「SpringBoot 定制化返回数据的实现示例」的完整攻略,包含以下内容:
下面是关于「SpringBoot 定制化返回数据的实现示例」的完整攻略,包含以下内容:
- 背景介绍
- 基本思路
- 实现步骤
- 示例说明
背景介绍
在实际开发中,我们经常需要对接口返回数据进行定制化处理。例如,有时候我们需要统一的返回格式、或者在某些接口中需要添加额外的字段信息。这时候,我们就需要对 SpringBoot 中默认的返回数据进行定制化处理。
基本思路
SpringBoot 默认使用 Jackson 库将对象序列化为 JSON 字符串,然后返回给前端。基于这个特点,我们可以通过定制化 Jackson 的序列化方式,来实现接口返回数据的自定义格式。
实现步骤
- 创建返回数据的基本模型类,例如:
public class ResponseData<T> {
private int code; //请求状态码
private String message; //请求响应信息
private T data; //请求返回数据
// 省略构造方法,Getter 和 Setter 方法
}
- 创建一个 Jackson 的序列化器,将 ResponseData 序列化成定制化的 JSON 数据。
@JsonComponent
public class CustomResponseBodyAdvice implements ResponseBodyAdvice<Object> {
@Override
public boolean supports(MethodParameter returnType, Class<? extends HttpMessageConverter<?>> converterType) {
// 判断返回类型是否为 ResponseData 类型
return returnType.getGenericParameterType().equals(ResponseData.class);
}
@Override
public Object beforeBodyWrite(Object body,
MethodParameter returnType,
MediaType selectedContentType,
Class<? extends HttpMessageConverter<?>> selectedConverterType,
ServerHttpRequest request,
ServerHttpResponse response) {
ResponseData<Object> responseData = new ResponseData<>();
if (body instanceof String) {
// 处理返回类型为 String 的情况
responseData.setMessage(body.toString());
} else {
// 处理普通 Object 类型的数据
responseData.setData(body);
}
// 自定义返回状态码和信息
responseData.setCode(response.getStatusCode().value());
responseData.setMessage(response.getStatus().getReasonPhrase());
return responseData;
}
}
- 在 SpringBoot 的配置文件中,增加 Jackson 序列化器的自动配置。
spring:
jackson:
default-property-inclusion: non_null # 设置 Jackson 默认忽略 null 值的属性
serialization:
indent-output: true # 设置序列化输出格式化
mapper:
registered-modules:
- com.example.demo.config.CustomResponseBodyAdvice
示例说明
下面给出两个示例说明:
示例1:定制化返回的状态码和信息。
对于这个示例,我们需要将默认的返回状态码和信息进行定制化。例如,将 404 错误返回的状态码和信息分别改成 101 和 Not Found。具体实现方法如下:
在 CustomResponseBodyAdvice 类的 beforeBodyWrite 方法中,增加如下代码块。
if (response.getStatusCode() == HttpStatus.NOT_FOUND) {
responseData.setCode(101);
responseData.setMessage("Not Found");
}
示例 2: 定制化返回数据中增加额外的字段信息。
对于这个示例,我们需要在默认返回数据的基础上,增加额外的字段信息。例如,接口返回的数据中,增加一个 current_time 字段,用于标识返回数据的时间。具体实现方法如下:
在 CustomResponseBodyAdvice 类的 beforeBodyWrite 方法中,获取当前时间,并将其添加到返回数据的基础上,增加如下代码块。
Instant instant = Instant.now(); // 获取当前时间
ZonedDateTime zonedDateTime = instant.atZone(ZoneId.systemDefault()); // 获取默认时区的时间
responseData.setCurrentTime(zonedDateTime.toString()); // 将其添加到 ResponseData 对象中
这就是关于「SpringBoot 定制化返回数据的实现示例」的完整攻略和示例说明。
本文标题为:SpringBoot 定制化返回数据的实现示例
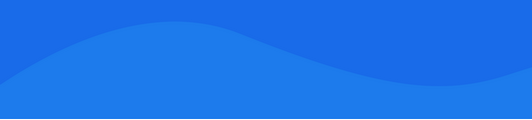
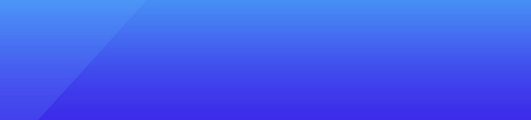
基础教程推荐
- jsp用过滤器解决中文乱码问题的方法 2023-08-01
- Java实现List集合转树形结构的示例详解 2023-04-17
- 在JSTL EL中处理java.util.Map,及嵌套List的情况 2024-01-11
- Android学习笔记45之gson解析json 2024-03-02
- MyBatis加载映射文件和动态代理的实现 2023-07-15
- idea web项目没有小蓝点的的两种解决方法 2023-02-27
- 图解Java ReentrantLock公平锁和非公平锁的实现 2023-06-10
- JDO 2.0查询语言的特点 2023-12-17
- Netty核心功能之数据容器ByteBuf详解 2023-06-17
- java高并发InterruptedException异常引发思考 2023-05-08