Pattern.compile是Java正则表达式中的一个方法,可以用来编译正则表达式并生成一个Pattern对象。该对象可以被用于创建Matcher对象,以执行匹配操作。
Java之Pattern.compile函数用法详解
什么是Pattern.compile函数
Pattern.compile
是Java正则表达式中的一个方法,可以用来编译正则表达式并生成一个Pattern
对象。该对象可以被用于创建Matcher
对象,以执行匹配操作。
Pattern.compile函数的语法
下面是Pattern.compile函数的语法:
public static Pattern compile(String regex);
public static Pattern compile(String regex, int flags);
其中:
regex
是一个Java字符串,表示要编译的正则表达式。-
flags
是一个整数,表示编译时的标志位,可以是以下值之一: -
Pattern.CANON_EQ
:启用规范等价。 Pattern.CASE_INSENSITIVE
:启用不区分大小写的匹配。Pattern.COMMENTS
:启用注释模式,可以在正则表达式中使用#
来注释。Pattern.DOTALL
:启用点字符(.
)匹配所有字符,包括行终止符。Pattern.UNICODE_CASE
:启用Unicode感知的大小写匹配。Pattern.UNIX_LINES
:启用Unix行模式。
Pattern.compile函数的使用步骤
Pattern.compile函数的使用可以分为以下步骤:
- 编写正则表达式。
- 调用Pattern.compile函数,将正则表达式编译成Pattern对象。
- 调用Pattern对象的matcher函数,创建一个Matcher对象。
- 调用Matcher对象的find函数,进行匹配操作。
示例1:用正则表达式匹配字符串
import java.util.regex.*;
public class Main {
public static void main(String[] args) {
String text = "The quick brown fox jumps over the lazy dog.";
String regex = "fox";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(text);
if (matcher.find()) {
System.out.println("Match found.");
} else {
System.out.println("Match not found.");
}
}
}
以上示例输出结果为:Match found.
示例2:使用Pattern.compile函数的flags参数
import java.util.regex.*;
public class Main {
public static void main(String[] args) {
String text = "The quick brown fox jumps over the lazy dog.";
String regex = "the";
Pattern pattern = Pattern.compile(regex, Pattern.CASE_INSENSITIVE);
Matcher matcher = pattern.matcher(text);
int count = 0;
while (matcher.find()) {
count++;
}
System.out.println("Found " + count + " matches.");
}
}
以上示例输出结果为:Found 2 matches.
总结
以上是Pattern.compile函数的使用详解。在实际开发中,我们经常需要使用正则表达式来进行字符串匹配操作,Pattern.compile函数可以帮助我们编译正则表达式并生成一个可复用的Pattern对象,提高代码的可读性和重用性。
本文标题为:Java之Pattern.compile函数用法详解
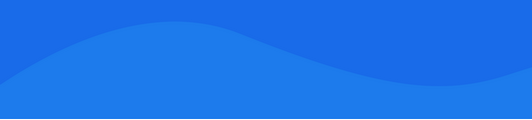
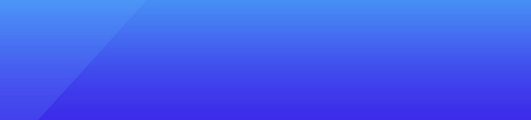
基础教程推荐
- java – mysql中UTC的日期 2023-11-05
- 使用Java桥接模式打破继承束缚优雅实现多维度变化 2023-07-15
- SpringCloud Config统一配置中心问题分析解决与客户端动态刷新实现 2023-06-23
- 超级全面的PHP面试题整理集合第1/2页 2023-12-15
- Java实现学生管理系统(控制台版本) 2022-12-27
- 在IDEA中运行Java程序 2023-10-08
- jsp要实现屏蔽退格键问题探讨 2024-02-25
- Java中Boolean和boolean的区别详析 2023-02-28
- 解析SpringBoot中使用LoadTimeWeaving技术实现AOP功能 2023-05-24
- 使用Java发送邮件 2023-10-08