Word中的替换功能以查找指定文本然后替换为新的文本,可单个替换或全部替换。本文将用Java语言实现Word中的文本、图片替换功能,需要的可以参考一下
前言
Word中的替换功能以查找指定文本然后替换为新的文本,可单个替换或全部替换。以下将要介绍的内容,除常见的以文本替换文本外,还将介绍使用不同对象进行替换的方法,具体可包括:
1. 指定字符串内容替换文本(通过方法replce(matchString, newValue, caseSensitive, wholeWord );直接指定替换的新字符串内容)
2. 获取文档内容替换文本(通过方法replace(String matchString, TextSelection textSelection, boolean caseSensitive, boolean wholeWord);替换指定文本)
3. 图片替换文本
4. 图片替换图片
使用工具及jar导入:
需要使用 Free Spire.Doc for Java 的jar包,可手动下载并解压导入Spire.Doc.jar文件到Java程序,也可以通过maven仓库下载导入。
1.指定字符串内容替换文本
import com.spire.doc.*;
public class ReplaceTextWithText {
public static void main(String[] args) {
//加载文档
Document doc = new Document();
doc.loadFromFile("test.docx");
//要替换第一个出现的指定文本,只需在替换前调用setReplaceFirst方法来指定只替换第一个出现的指定文本
//doc.setReplaceFirst(true);
//调用方法用新文本替换原文本内容
doc.replace("系统测试", "System Testing", false, true);
//保存文档
doc.saveToFile("ReplaceAllText.docx",FileFormat.Docx_2013);
doc.dispose();
}
}
2.获取文档内容替换文本
import com.spire.doc.*;
import com.spire.doc.documents.TextSelection;
public class ReplaceTextWithDocument {
public static void main(String[] args) {
//加载文档1
Document doc1 = new Document();
doc1.loadFromFile("test.docx");
//加载文档2
Document doc2 = new Document();
doc2.loadFromFile("TargetFile.docx");
//查找文档2中的指定内容
TextSelection textSelection = doc2.findString("Falling under the scope of black box testing, " +
"system testing is a phase in the software " +
"testing cycle where a total and integrated" +
" application /system is tested.",false,false);
//用文档2中查找到的内容替换文档1中的指定字符串
doc1.replace("System Test, ST",textSelection,false,true);
//保存文档1
doc1.saveToFile("ReplaceTextWithDocument.docx",FileFormat.Docx_2013);
doc1.dispose();
}
}
两个用于测试的文档如下,将文档2中的文本内容替换文档1中的指定文本内容:
替换结果:
3.图片替换文本
import com.spire.doc.*;
import com.spire.doc.documents.TextSelection;
import com.spire.doc.fields.DocPicture;
import com.spire.doc.fields.TextRange;
public class ReplaceTextWithImg {
public static void main(String[] args) {
//加载文档
Document doc = new Document("test.docx");
//查找需要替换的字符串
TextSelection[] textSelection = doc.findAllString("系统测试",true,false);
int index ;
//加载图片替换文本字符串
for (Object obj : textSelection) {
TextSelection Selection = (TextSelection)obj;
DocPicture pic = new DocPicture(doc);
pic.loadImage("tp.png");
TextRange range = Selection.getAsOneRange();
index = range.getOwnerParagraph().getChildObjects().indexOf(range);
range.getOwnerParagraph().getChildObjects().insert(index,pic);
range.getOwnerParagraph().getChildObjects().remove(range);
}
//保存文档
doc.saveToFile("ReplaceTextWithImage.docx", FileFormat.Docx_2013);
doc.dispose();
}
}
4.图片替换图片
import com.spire.doc.*;
import com.spire.doc.documents.Paragraph;
import com.spire.doc.fields.DocPicture;
public class ReplacePictureWithPicture {
public static void main(String[] args) {
//加载Word文档
Document doc = new Document();
doc.loadFromFile("sample.docx");
//获取文档中的指定段落
Section section = doc.getSections().get(0);
Paragraph para = section.getParagraphs().get(0);
//替换段落中的第一张图片
Object obj = para.getChildObjects().get(0);
if(obj instanceof DocPicture){
DocPicture pic = (DocPicture)obj;
pic.loadImage("tp.png");
}
/*//批量替换图片
for(int i =0;i < section.getParagraphs().getCount();i++){
Object obj = section.getParagraphs().get(i).getChildObjects();
if(obj instanceof DocPicture){
DocPicture pic = (DocPicture)obj;
pic.loadImage("tp.png");
}
}*/
//保存结果文档
doc.saveToFile("ReplaceWithImage.docx", FileFormat.Docx_2013);
doc.dispose();
}
}
到此这篇关于Java实现替换Word中文本和图片功能的文章就介绍到这了,更多相关Java替换Word文本 图片内容请搜索编程学习网以前的文章希望大家以后多多支持编程学习网!
本文标题为:Java实现替换Word中文本和图片功能
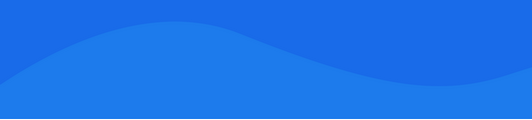
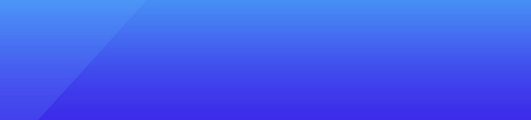
基础教程推荐
- JDK数组阻塞队列源码深入分析总结 2023-04-18
- Java实现查找文件和替换文件内容 2023-04-06
- ConditionalOnProperty配置swagger不生效问题及解决 2023-01-02
- Java文件管理操作的知识点整理 2023-05-19
- Java数据结构之对象比较详解 2023-03-07
- java基础知识之FileInputStream流的使用 2023-08-11
- java实现多人聊天系统 2023-05-19
- Java实现线程插队的示例代码 2022-09-03
- Java并发编程进阶之线程控制篇 2023-03-07
- springboot自定义starter方法及注解实例 2023-03-31