PHP中的预定义接口是指在类的内部定义特定的方法,以实现与其他对象或系统交互的标准化方式。这些接口已经在PHP中定义好了,并且拥有了相应的语法和特性。实现这些预定义接口可以使你的类可以更好的兼容PHP中的其他类和系统。
PHP之预定义接口详解
什么是预定义接口
PHP中的预定义接口是指在类的内部定义特定的方法,以实现与其他对象或系统交互的标准化方式。这些接口已经在PHP中定义好了,并且拥有了相应的语法和特性。实现这些预定义接口可以使你的类可以更好的兼容PHP中的其他类和系统。
下面我们详细介绍几个常用的PHP预定义接口及其实现方法。
Iterator
Iterator接口是PHP中一个非常重要的预定义接口,用于表现一种可以迭代的对象。
接口方法
Iterator接口中包含了以下五个方法:
- current(): 返回当前元素。
- next(): 移动到下一个元素。
- rewind(): 将迭代器重置为起始位置。
- valid(): 返回当前位置是否存在元素。
- key(): 返回当前位置的键。
示例实现
下面是一个示例实现Iterator接口的类:
class MyIterator implements Iterator {
private $items = [];
private $position = 0;
public function __construct($array) {
$this->items = $array;
$this->position = 0;
}
public function rewind() {
$this->position = 0;
}
public function current() {
return $this->items[$this->position];
}
public function key() {
return $this->position;
}
public function next() {
++$this->position;
}
public function valid() {
return isset($this->items[$this->position]);
}
}
ArrayAccess
ArrayAccess接口是PHP中用来表现数组式访问的对象的预定义接口,它可以让一个对象像数组一样被访问。
接口方法
ArrayAccess接口中包含了以下三个方法:
- offsetExists($offset): 返回指定的偏移量是否存在。
- offsetGet($offset): 获取指定偏移量的值。
- offsetSet($offset, $value): 设置指定偏移量的值。
- offsetUnset($offset): 删除指定偏移量的值。
示例实现
下面是一个使用ArrayAccess接口实现的简单类:
class MyArray implements ArrayAccess {
private $container = [];
public function __construct() {
$this->container = [];
}
public function offsetSet($offset, $value) {
if (is_null($offset)) {
$this->container[] = $value;
} else {
$this->container[$offset] = $value;
}
}
public function offsetExists($offset) {
return isset($this->container[$offset]);
}
public function offsetUnset($offset) {
unset($this->container[$offset]);
}
public function offsetGet($offset) {
return isset($this->container[$offset]) ? $this->container[$offset] : null;
}
}
总结
在PHP中,预定义接口是非常有用的工具,可以帮助我们更好地管理和控制类的行为。上面提到的两个接口都是非常常见和使用的接口。在实际开发中,我们可以根据需要实现和应用不同的预定义接口,以达到更好的效果。
本文标题为:PHP之预定义接口详解
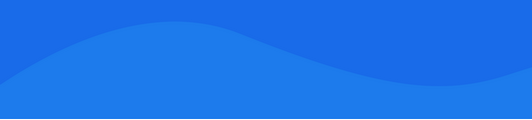
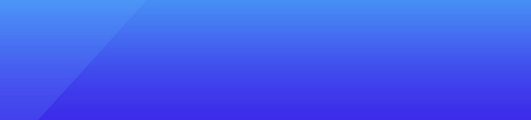
基础教程推荐
- 解决laravel中日志权限莫名变成了root的问题 2023-03-03
- ThinkPHP5.1表单令牌Token失效问题的解决 2023-01-04
- PHP如何通过date() 函数格式化显示时间 2023-05-03
- php5.6.x到php7.0.x特性小结 2023-02-05
- php进程通信之信号量浅析介绍 2023-07-03
- PHP实现的Redis操作通用类示例 2022-11-04
- 微信公众平台开发教程⑥ 微信开发集成类的使用图文详解 2023-01-05
- PHP下利用header()函数设置浏览器缓存的代码 2023-08-03
- CI框架安全过滤函数示例 2022-11-04
- 基于PHP+Mysql简单实现了图书购物车系统的实例详解 2023-04-25