下面是详细的攻略:
下面是详细的攻略:
PHP检测数据类型的几种方法(总结)
在PHP中,检测数据类型是开发中常见的操作。下面是几种PHP检测数据类型的方法:
方法一:使用gettype()函数
gettype()函数可以返回一个给定变量的类型。例如:
<?php
$str = 'hello';
echo gettype($str); // 输出:string
?>
方法二:使用is_numeric()函数
is_numeric()函数可以检查一个变量是否为数字或数字字符串。例如:
<?php
$num = 123;
if(is_numeric($num)){
echo "num is numeric";
} else {
echo "num is not numeric";
}
?>
方法三:使用is_array()函数
is_array()函数可以检查一个变量是否为数组类型。例如:
<?php
$arr = array('a', 'b', 'c');
if(is_array($arr)){
echo "arr is an array";
} else {
echo "arr is not an array";
}
?>
方法四:使用is_object()函数
is_object()函数可以检查一个变量是否为对象类型。例如:
<?php
class Test {
function sayHello() {
echo "Hello";
}
}
$obj = new Test();
if(is_object($obj)){
echo "obj is an object";
} else {
echo "obj is not an object";
}
?>
示例一:判断变量类型并输出相应的信息
下面的示例演示了如何使用gettype()函数来判断变量类型并输出相应的信息:
<?php
$a = 123;
$b = "hello";
$c = true;
$d = array();
echo "变量a的类型是:" . gettype($a) . "<br/>";
echo "变量b的类型是:" . gettype($b) . "<br/>";
echo "变量c的类型是:" . gettype($c) . "<br/>";
echo "变量d的类型是:" . gettype($d) . "<br/>";
?>
输出结果为:
变量a的类型是:integer
变量b的类型是:string
变量c的类型是:boolean
变量d的类型是:array
示例二:检查变量是否为整数
下面的示例演示了如何使用is_numeric()函数来检查变量是否为整数:
<?php
$num1 = 123;
$num2 = '456';
$str = 'hello';
if(is_numeric($num1)){
echo "num1是数字<br/>";
} else {
echo "num1不是数字<br/>";
}
if(is_numeric($num2)){
echo "num2是数字<br/>";
} else {
echo "num2不是数字<br/>";
}
if(is_numeric($str)){
echo "str是数字<br/>";
} else {
echo "str不是数字<br/>";
}
?>
输出结果为:
num1是数字
num2是数字
str不是数字
希望这个攻略能够帮助你理解PHP检测数据类型的几种方法。
沃梦达教程
本文标题为:PHP检测数据类型的几种方法(总结)
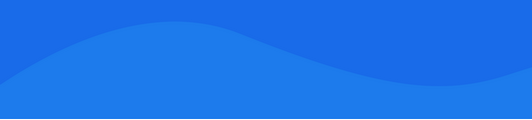
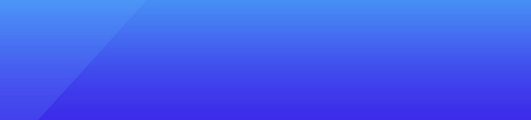
基础教程推荐
猜你喜欢
- PHP5.5新特性之yield理解与用法实例分析 2022-12-05
- Mac系统下安装PHP Xdebug 2022-10-12
- PHP之CI框架学习讲解 2023-06-19
- php使用QueryList轻松采集js动态渲染页面方法 2022-11-14
- php计算十二星座的函数代码 2024-01-16
- Laravel使用swoole实现websocket主动消息推送的方法介绍 2023-03-03
- PHP进阶学习之依赖注入与Ioc容器详解 2023-01-20
- PHP的数组中提高元素查找与元素去重的效率的技巧解析 2024-01-15
- PHP const定义常量及global定义全局常量实例解析 2023-04-20
- PHP正则匹配到2个字符串之间的内容方法 2022-12-01