PHP可以通过SimpleXMLElement类来实现将数组转换为XML的操作,步骤如下:
PHP可以通过SimpleXMLElement类来实现将数组转换为XML的操作,步骤如下:
- 创建一个SimpleXMLElement对象。
$xml = new SimpleXMLElement('<?xml version="1.0" encoding="UTF-8"?><root></root>');
- 将数组转换为XML节点并添加到SimpleXMLElement对象中。
function arrayToXml($arr, &$xml) {
foreach ($arr as $key => $value) {
if (is_array($value)) {
$child = $xml->addChild($key);
arrayToXml($value, $child);
} else {
$xml->addChild($key, $value);
}
}
}
上述代码中的arrayToXml函数将传入的数组转换为XML节点并递归地添加到SimpleXMLElement对象中。
- 输出生成的XML字符串。
header("Content-type: text/xml");
echo $xml->asXML();
完整示例:
$arr = array(
"name" => "John Doe",
"age" => 30,
"address" => array(
"street" => "123 Main St",
"city" => "Anytown",
"state" => "CA",
"zip" => "12345"
)
);
$xml = new SimpleXMLElement('<?xml version="1.0" encoding="UTF-8"?><root></root>');
arrayToXml($arr, $xml);
header("Content-type: text/xml");
echo $xml->asXML();
输出结果:
<?xml version="1.0" encoding="UTF-8"?>
<root>
<name>John Doe</name>
<age>30</age>
<address>
<street>123 Main St</street>
<city>Anytown</city>
<state>CA</state>
<zip>12345</zip>
</address>
</root>
另一个示例:
$arr = array(
"book" => array(
array(
"title" => "Harry Potter and the Philosopher's Stone",
"author" => "J.K. Rowling",
"year" => 1997
),
array(
"title" => "The Hitchhiker's Guide to the Galaxy",
"author" => "Douglas Adams",
"year" => 1979
)
)
);
$xml = new SimpleXMLElement('<?xml version="1.0" encoding="UTF-8"?><root></root>');
arrayToXml($arr, $xml);
header("Content-type: text/xml");
echo $xml->asXML();
输出结果:
<?xml version="1.0" encoding="UTF-8"?>
<root>
<book>
<item>
<title>Harry Potter and the Philosopher's Stone</title>
<author>J.K. Rowling</author>
<year>1997</year>
</item>
<item>
<title>The Hitchhiker's Guide to the Galaxy</title>
<author>Douglas Adams</author>
<year>1979</year>
</item>
</book>
</root>
沃梦达教程
本文标题为:PHP实现数组array转换成xml的方法
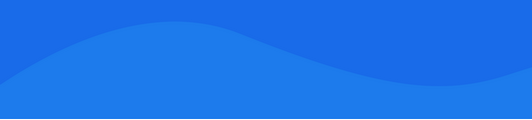
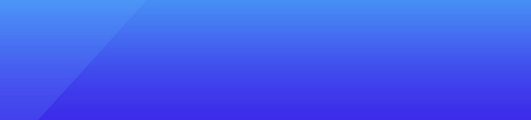
基础教程推荐
猜你喜欢
- thinkphp 3.2框架视图模型 实例视图查询结果的二维数组合并操作示例 2023-04-02
- PhpWord的一些简单用法 2022-09-21
- 详解PHP队列的实现 2022-12-30
- TP5框架实现签到功能的方法分析 2023-04-07
- php网页上传文件到Ubuntu服务器(input type=fire) 2023-09-02
- bindParam和bindValue的区别以及在Yii2中的使用详解 2022-10-08
- 在 Laravel 中动态隐藏 API 字段的方法 2023-03-12
- one.php 多项目、函数库、类库 统一为一个版本的方法 2023-04-25
- PHP PDOStatement::setFetchMode讲解 2022-12-12
- Laravel统一封装接口返回状态实例讲解 2023-05-20