要在Python中实现PHP中的var_dump函数的功能,需要运用Python的一些内置模块和数据类型。
要在Python中实现PHP中的var_dump函数的功能,需要运用Python的一些内置模块和数据类型。
具体步骤如下:
1. 获取变量的类型
使用Python的type()函数获取变量的类型,该函数返回变量的类型对象。
example1 = 10
example2 = 'Hello World'
example3 = [1,2,3]
example4 = {'name':'Mike', 'age':20}
print(type(example1)) # <class 'int'>
print(type(example2)) # <class 'str'>
print(type(example3)) # <class 'list'>
print(type(example4)) # <class 'dict'>
2. 根据类型输出变量信息
根据变量类型的不同,输出变量信息也不同。
对于整型和浮点型变量,直接输出变量值即可。
example1 = 10
example2 = 5.6
if isinstance(example1, (int, float)):
print(example1)
if isinstance(example2, (int, float)):
print(example2)
对于字符串型变量,输出字符串长度和字符串内容。
example = 'Hello World'
if isinstance(example, str):
print('string({}) "{}"'.format(len(example), example))
对于列表型变量,输出列表长度和列表元素的类型和值。
example = [1,2,'three', 'four']
if isinstance(example, list):
print('array({})'.format(len(example)))
for index, value in enumerate(example):
print('[{}]: {}'.format(index, value))
对于字典型变量,输出字典键和值的类型和值。
example = {'name':'Mike', 'age':20}
if isinstance(example, dict):
print('array({})'.format(len(example)))
for key, value in example.items():
print('[{}]: {}'.format(key, value))
示例说明
示例1
输入变量:
example = [1,2,3]
输出结果:
array(3)
[0]: 1
[1]: 2
[2]: 3
解释:该变量为列表类型变量,输出列表的长度和每个元素的类型和值。
示例2
输入变量:
example = {'name':'Mike', 'age':20}
输出结果:
array(2)
[name]: Mike
[age]: 20
解释:该变量为字典类型变量,输出字典的长度和每个键值对的类型和值。
沃梦达教程
本文标题为:python中实现php的var_dump函数功能
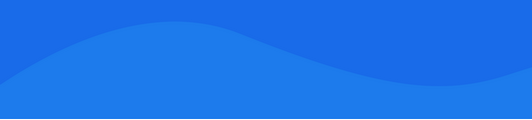
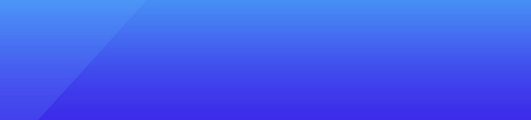
基础教程推荐
猜你喜欢
- php小技巧之过滤ascii控制字符 2024-01-31
- Laravel基础_关于view共享数据的示例讲解 2023-03-02
- php缓存的类型总结及用法 2023-06-25
- Laravel框架实现文件上传的方法分析 2023-02-21
- 解决在Laravel 中处理OPTIONS请求的问题 2023-02-22
- Thinkphp5框架简单实现钩子(Hook)行为的方法示例 2023-02-06
- PHP入门学习的几个不错的实例代码 2023-12-20
- PHP命名空间namespace定义及导入use用法详解 2022-10-09
- php常用日期时间函数实例小结 2023-01-25
- PHP获取文件属性的最简单方法 2022-09-02