CSS 嵌套DIV布局是指在 HTML 页面中嵌套多个DIV块,使用CSS的position属性对这些块进行定位和布局,实现多个块按一定的规则排列的效果。下面是实现CSS嵌套DIV布局的完整攻略:
CSS 嵌套DIV布局是指在 HTML 页面中嵌套多个DIV块,使用CSS的position属性对这些块进行定位和布局,实现多个块按一定的规则排列的效果。下面是实现CSS嵌套DIV布局的完整攻略:
步骤一:HTML 结构准备
在HTML页面中嵌套多个DIV块,使用id或class属性封装起来,方便使用CSS对它们进行布局。
下面是一个HTML结构示例:
<body>
<div id="container">
<div class="box">1</div>
<div class="box">2</div>
<div class="box">3</div>
<div class="box">4</div>
<div class="box">5</div>
</div>
</body>
步骤二:CSS 样式设置
设置容器DIV #container 和每个块DIV .box 的样式,绑定每个块的位置和尺寸。具体样式如下:
#container {
position: relative;
}
.box {
position: absolute;
width: 100px;
height: 100px;
}
步骤三:块的位置设置
使用CSS的top,right,bottom,left属性,设置容器DIV #container 内每个块的位置,使用百分比或像素单位。具体位置如下:
.box:nth-child(1) {
top: 0;
left: 0;
background: red;
}
.box:nth-child(2) {
top: 0;
right: 0;
background: yellow;
}
.box:nth-child(3) {
bottom: 0;
left: 0;
background: blue;
}
.box:nth-child(4) {
bottom: 0;
right: 0;
background: green;
}
.box:nth-child(5) {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
background: purple;
}
上面的样式设置中,第 5 个块是相对于 #container 容器垂直居中和水平居中的,使用了 transform 属性。
步骤四:完整的代码示例
<style>
#container {
position: relative;
}
.box {
position: absolute;
width: 100px;
height: 100px;
}
.box:nth-child(1) {
top: 0;
left: 0;
background: red;
}
.box:nth-child(2) {
top: 0;
right: 0;
background: yellow;
}
.box:nth-child(3) {
bottom: 0;
left: 0;
background: blue;
}
.box:nth-child(4) {
bottom: 0;
right: 0;
background: green;
}
.box:nth-child(5) {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
background: purple;
}
</style>
<body>
<div id="container">
<div class="box">1</div>
<div class="box">2</div>
<div class="box">3</div>
<div class="box">4</div>
<div class="box">5</div>
</div>
</body>
示例1:实现三列等高布局
在同一行内显示三列等高的区块,每个区块高度根据内容自适应,不足一行可自动换行。可以使用以下代码实现:
<style>
#container {
display: flex;
flex-wrap: wrap;
}
.col {
width: 33.33%;
padding: 10px;
box-sizing: border-box;
}
.box {
position: relative;
height: 100%;
}
.box-inner {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%,-50%);
width: 80%;
padding: 10px;
box-sizing: border-box;
}
.box:nth-child(1) .box-inner {
background: red;
}
.box:nth-child(2) .box-inner {
background: yellow;
}
.box:nth-child(3) .box-inner {
background: blue;
}
</style>
<body>
<div id="container">
<div class="col">
<div class="box">
<div class="box-inner">box1</div>
</div>
</div>
<div class="col">
<div class="box">
<div class="box-inner">box2</div>
</div>
</div>
<div class="col">
<div class="box">
<div class="box-inner">box3</div>
</div>
</div>
</div>
</body>
示例2:实现背景全屏,内容局中
在背景中添加一个包含内容的区块,除了显示在前端的内容,其余部分都被覆盖。利用绝对定位与覆盖,实现背景全屏,内容局中的效果。代码如下:
<style>
html, body {
height: 100%;
}
body {
margin: 0;
background: url(bg.jpg) no-repeat center center fixed;
background-size: cover;
}
#container {
position: relative;
height: 100%;
}
.content {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%,-50%);
padding: 30px;
background: rgba(255, 255, 255, .8);
border-radius: 10px;
}
</style>
<body>
<div id="container">
<div class="content">
<h1>Hello</h1>
<p>这里是一段文本内容</p>
<button>点击按钮</button>
</div>
</div>
</body>
以上是 CSS 嵌套DIV布局的完整攻略,希望对你有所帮助。
本文标题为:CSS 嵌套DIV布局(position属性)
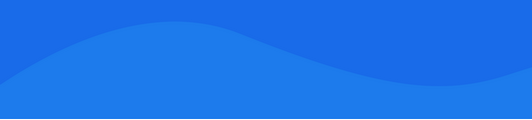
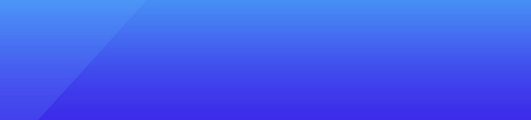
基础教程推荐
- 详解浏览器的缓存机制 2022-11-13
- Vue2.0 $set()的正确使用方式 2023-10-08
- 解决 Django 渲染模板 与 Vue {{ }} 冲突 2023-10-08
- sql、linq、lambda三种表达方式转换 转自https://www.cnblogs.com/drzhong/p/4393231.html 2023-10-26
- 一文掌握在Vue3中书写TSX的使用方法 2023-07-09
- Ajax传输中文乱码问题的解决办法 2023-01-20
- 16、laravel8 + inertia + vue3 2023-10-08
- VUE新增属性-数据更新页面不更新 2023-10-08
- 手把手教你用Javascript实现观察者模式 2023-08-12
- 小白前端入门笔记(七),HTML5中的main标签 2023-10-28