下面来详细讲解一下“uniapp 页面间传参的几种方法实例总结”。
下面来详细讲解一下“uniapp 页面间传参的几种方法实例总结”。
uniapp 页面间传参的几种方法实例总结
一、通过URL进行传参
通过URL进行传参,是最常见也最简单的方法。通过修改URL中的参数,实现页面之间数据的传递。
一般来说,我们使用vue-router进行URL的跳转,可以通过$route对象来获取URL中的参数,如下所示:
// 跳转到目标页面并传递参数
this.$router.push({
path: '/target',
query: { id: 123, name: 'John' }
})
// 目标页面中获取参数
this.$route.query.id // 输出:123
this.$route.query.name // 输出:John
这种方式的优点是使用简单、易于理解,缺点是不能传递复杂对象,只能传递简单的值类型。
二、使用vuex进行传参
vuex是vue.js的状态管理器,它可以用来管理全局状态,实现不同组件之间的数据共享。
- 首先,在
store
文件夹中新建一个index.js
文件,定义state
、mutations
、actions
等状态管理对象。
// store/index.js
export default new Vuex.Store({
state: {
username: '',
age: 0
},
mutations: {
setUserName(state, name) {
state.username = name
},
setAge(state, age) {
state.age = age
}
},
actions: {
updateUserName({ commit }, name) {
commit('setUserName', name)
},
updateAge({ commit }, age) {
commit('setAge', age)
}
}
})
- 在需要传参的组件中,调用
$store
对象,通过this.$store.commit
或this.$store.dispatch
方法来更新、获取全局状态。
// components/UserName.vue
<template>
<div>
<span>用户名:</span>{{ username }}
<button @click="updateName">修改</button>
</div>
</template>
<script>
export default {
computed: {
username() {
return this.$store.state.username
}
},
methods: {
updateName() {
this.$store.dispatch('updateUserName', 'Tom')
}
}
}
</script>
这种方式的优点是可以传递复杂对象,实现数据共享,缺点是需要安装vuex插件,使用稍微复杂。
三、使用全局变量进行传参
在uniapp中,可以使用uni.$emit
方法和全局变量(比如uni.$app
)来实现页面间的通信。
- 定义全局变量,并修改其属性值:
// App.vue
<template>
<view>
<uni-nav-bar title="首页"></uni-nav-bar>
<router-view></router-view>
</view>
</template>
<script>
export default {
data() {
return {
username: ''
}
},
methods: {
setName(name) {
this.username = name
}
},
mounted() {
uni.$app = this
}
}
</script>
- 在目标页面中,调用全局变量的方法来修改属性值,实现数据传递。
// components/UserName.vue
<template>
<div>
<span>用户名:</span>{{ username }}
<button @click="updateName">修改</button>
</div>
</template>
<script>
export default {
computed: {
username() {
return this.$app.username
}
},
methods: {
updateName() {
this.$app.setName('Tom')
}
}
}
</script>
这种方式的优点是使用简单、易于理解,缺点是会造成全局变量污染,不利于代码的维护和复用。
以上是关于uniapp 页面间传参的几种方法实例总结,希望可以帮助到读者。
沃梦达教程
本文标题为:uniapp页面间传参的几种方法实例总结
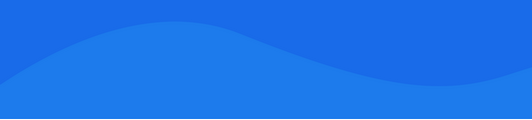
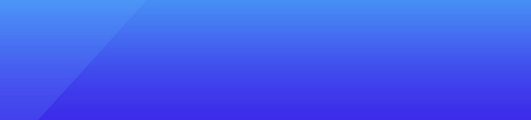
基础教程推荐
猜你喜欢
- ajax提交到java后台之后处理数据的实现 2023-02-01
- html,css基础(2)~元素盒模型,浮动布局,弹性布局 2023-10-28
- Ajax跨域访问Cookie丢失问题的解决方法 2023-01-26
- JS跨域总结 2024-01-05
- AJAX实现指定部分页面刷新效果 2023-02-23
- Ajax异步请求技术实例讲解 2023-02-14
- IE9 IE8 ajax跨域问题的快速解决方法 2023-01-20
- 纯CSS3绘制打火机动画火焰效果 2023-12-22
- php – 如何将HTML表单中的数字添加到数据库中已有的数字? 2023-10-26
- 基于Spring Boot利用 ajax实现上传图片功能 2023-02-23