在图像处理中,矩阵是重要的数据结构。Javascript 作为一门强大的编程语言,可以非常方便地完成矩阵的各种操作。在本篇文章中,我们将讲解为矩阵添加一些常用方法的过程,以便于以后的图像处理中使用。
Javascript 图像处理 - 为矩阵添加常用方法
前言
在图像处理中,矩阵是重要的数据结构。Javascript 作为一门强大的编程语言,可以非常方便地完成矩阵的各种操作。在本篇文章中,我们将讲解为矩阵添加一些常用方法的过程,以便于以后的图像处理中使用。
实现常用矩阵方法
为了方便起见,我们在这里定义一个矩阵的类:
class Matrix {
constructor(rows, cols, data) {
this.rows = rows;
this.cols = cols;
this.data = data;
}
get(row, col) {
return this.data[row][col];
}
set(row, col, val) {
this.data[row][col] = val;
}
getValueList() {
let res = [];
for(let i = 0; i < this.rows; i++) {
for(let j = 0; j < this.cols; j++) {
res.push(this.data[i][j]);
}
}
return res;
}
}
接下来我们为矩阵添加常用的方法:
转置
矩阵的转置是指将矩阵的行和列互换。可以使用Javascript的Array原型的 map
和 reduce
方法实现:
Matrix.prototype.transpose = function() {
return new Matrix(this.cols, this.rows, this.data[0].map((val, colIndex) =>
this.data.map((row) => row[colIndex])
));
}
求和
矩阵的求和是指将所有的元素相加。可以使用Javascript的Array原型的 reduce
方法实现:
Matrix.prototype.sum = function() {
return this.getValueList().reduce((a, b) => a + b);
}
示例
下面我们使用刚才实现的矩阵类和方法,演示一下矩阵的转置和求和的用法。
转置
let data = [[1, 2, 3], [4, 5, 6], [7, 8, 9]];
let m1 = new Matrix(3, 3, data);
let m2 = m1.transpose();
console.log(m1.data); // [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
console.log(m2.data); // [[1, 4, 7], [2, 5, 8], [3, 6, 9]]
求和
let data = [[1, 2, 3], [4, 5, 6], [7, 8, 9]];
let m = new Matrix(3, 3, data);
console.log(m.sum()); // 45
结论
在本篇文章中,我们演示了为矩阵添加常用方法的过程,并提供了转置和求和的示例。除此之外,还可以根据需要添加其他矩阵方法,以方便以后的图像处理使用。
沃梦达教程
本文标题为:Javascript图像处理—为矩阵添加常用方法
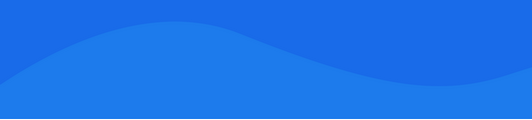
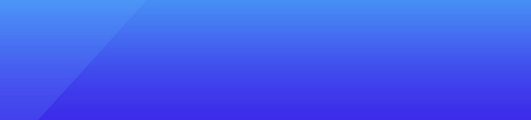
基础教程推荐
猜你喜欢
- Ajax异步上传文件实例代码分享 2023-01-20
- 重写 ajax 实现 session 超时跳转到登录页面实例代码 2023-02-01
- JavaScript统计数组中相同的数量的方法总结 2023-08-08
- 使用JavaScript获取电池状态的方法 2024-01-06
- 详解IE6中的position:fixed问题与随滚动条滚动的效果 2022-11-13
- 分享我学习js的过程 作者aircy javascript学习教程 2024-01-05
- javascript异步处理工作机制详解 2024-01-07
- 「免费开源」基于Vue和Quasar的前端SPA项目crudapi后台管理系统实战之业务数据增删改查(七) 2023-10-08
- 微信小程序自定义用户登录弹窗 2024-01-07
- Select2在使用ajax获取远程数据时显示默认数据的方法 2023-02-23