与iframe进行跨域交互是前端开发中常见的场景,但是由于同源策略的限制,直接使用JavaScript操作跨域iframe是不被允许的。那么,如何解决这一问题呢?
与iframe进行跨域交互是前端开发中常见的场景,但是由于同源策略的限制,直接使用JavaScript操作跨域iframe是不被允许的。那么,如何解决这一问题呢?
以下是利用postMessage进行与iframe跨域交互的解决方案(推荐):
步骤一:在iframe的源码中添加监听器
<html>
<head>
<script>
window.addEventListener("message", event => {
if (event.origin !== "https://parent.com") return; // 只接受父级窗口来自"https://parent.com"的信息
console.log(`received message: ${event.data}`);
// 接受到消息后,可以执行相应操作
});
</script>
</head>
<body>
<!-- 此处省略iframe内容 -->
</body>
</html>
上述代码中,我们使用window对象的addEventListener方法,添加一条消息监听器。当父级窗口向iframe发送消息时,该监听器会自动被触发,从而实现跨域交互。
步骤二:在父级窗口中向iframe发送信息
<!DOCTYPE html>
<html>
<head>
<script>
const iframe = document.querySelector("#myIframe");
iframe.contentWindow.postMessage("hello, iframe!", "https://iframe.com"); // 向iframe发送消息
</script>
</head>
<body>
<iframe src="https://iframe.com" id="myIframe"></iframe>
</body>
</html>
上述代码中,我们首先获取到iframe元素,然后使用iframe.contentWindow.postMessage方法向其发送一条消息,其中第一个参数是要发送的消息内容,第二个参数是目标窗口的源地址。
需要注意的是,由于postMessage方法具有一定的危险性,因此我们在监听器中添加了判断语句,限制了只接受来自指定源的消息。
示例二:
<html>
<head>
<script>
window.addEventListener("message", function (event) {
if (event.source != window.parent) return; // 只接受父级窗口信息
console.log(event.data);
}, false);
</script>
</head>
<body>
<!-- 此处省略 iframe 内容 -->
</body>
</html>
<html>
<body>
<script>
const iframe = document.createElement('iframe');
iframe.src = 'http://localhost:8080/';
iframe.style.display = 'none';
document.body.appendChild(iframe);
iframe.onload = () => {
iframe.contentWindow.postMessage('hello, iframe! from parent', 'http://localhost:8080/');
};
</script>
</body>
</html>
这里,我们在父级窗口中动态创建了一个隐藏的iframe,并在iframe加载成功后,向其发送了一条消息,携带了要传递的数据。
需要注意的是,如果当前窗口的url为https,而iframe的url为http,则需要使用http发送postMessage消息,并找到一个可以通过http访问的端口。
以上是两个使用postMessage进行与iframe跨域交互的示例。总的来说,这种解决方案比较方便、简单,并且较为安全,是目前前端开发中常用的解决跨域问题的方法之一。
本文标题为:与iframe进行跨域交互的解决方案(推荐)
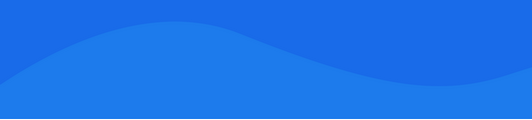
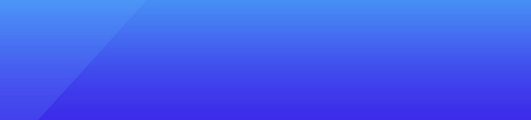
基础教程推荐
- Android WebView与JS交互全面详解(小结) 2024-02-05
- ajax详解_动力节点Java学院整理 2023-02-14
- javascript对下拉列表框(select)的操作实例讲解 2023-12-01
- js实现用户离开页面前提示是否离开此页面的方法(包括浏览器按钮事件) 2024-01-08
- JS(vue iview)分页解决方案 2023-10-08
- Ajax实现动态显示并操作表信息的方法 2023-02-23
- JavaScript实现带音效的烟花特效 2023-08-12
- 使用Ajax模仿百度搜索框的自动提示功能实例 2023-02-23
- layui实现表格内下拉框 2023-11-30
- Vue:三种情况下的生命周期执行顺序 2023-10-08