让我来为您详细讲解“jQuery实现div浮动层跟随页面滚动效果”的完整攻略:
让我来为您详细讲解“jQuery实现div浮动层跟随页面滚动效果”的完整攻略:
1. 引入jQuery库
在页面中引入jQuery库,可以使用CDN或者下载后引入本地:
<script src="https://cdn.bootcss.com/jquery/3.4.1/jquery.min.js"></script>
2. HTML结构设置
在HTML中,定义一个需要浮动的div
,并设置其初始状态为“不浮动”。可以使用CSS的position
属性将div
设置为绝对定位,然后初始设置为bottom:-50px;left:50%;margin-left:-100px;
,即位于底部中间的位置,z-index
属性为1,用于设置显示层级。
<style>
.floating {
position: absolute;
bottom: -50px;
left: 50%;
margin-left: -100px;
z-index: 1;
}
</style>
<div class="floating">
<p>浮动层的内容</p>
</div>
3. jQuery实现滚动效果
使用jQuery中的$(window).scroll()
方法来实现滚动效果:
$(window).scroll(function() {
var top = $(window).scrollTop();
$('.floating').stop().animate({
'top': top + 100
}, 500);
});
解释一下上述代码:
$(window).scroll()
监听了窗口的滚动事件,当滚动页面时就会触发该函数;$(window).scrollTop()
获取当前页面滚动的距离(即上端到顶部的距离),并赋值给变量top
;- 使用
.stop()
方法停止当前正在进行的动画,以避免滚动过快导致浮动层的位移和位置计算出现逻辑错误; .animate()
方法设置浮动层的动画效果,将top
属性设为当前滚动距离加上100px,即设置为固定距离滚动页面;500
为动画的执行时间,单位是毫秒。
4. 完整代码
下面是完整的代码:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Floating layer scrolling effect</title>
<style>
.container {
height: 2000px;
}
.floating {
position: absolute;
bottom: -50px;
left: 50%;
margin-left: -100px;
z-index: 1;
}
</style>
<script src="https://cdn.bootcss.com/jquery/3.4.1/jquery.min.js"></script>
<script>
$(function() {
$(window).scroll(function() {
var top = $(window).scrollTop();
$('.floating').stop().animate({
'top': top + 100
}, 500);
});
});
</script>
</head>
<body>
<div class="container">
<h1>浮动层跟随页面滚动效果演示</h1>
<p>这是一个演示的页面,里面有很多内容...</p>
<div class="floating">
<p>这是漂浮层的内容</p>
</div>
</div>
</body>
</html>
5. 示例说明
示例1:“浮动层”跟随滚动条
首先,需要模拟一个可以滚动的页面。
<div class="scrollable" style="height: 500px; overflow: auto;">
<h2>可以滚动的内容</h2>
<p>这里是内容的开始...</p>
<p>这里是内容的中间...</p>
<p>这里是内容的结尾...</p>
</div>
接下来,我们需要定义符合要求的div
,以获取“浮动的效果”。
<div class="floating-container" style="position: sticky; top: 50px;">
<h3>我是一个浮动层</h3>
<p>我一旦「起飞」,就会在您的页面中随意移动。</p>
</div>
初始化sccs样式:
.floating-container {
background-color: #f8f8f8;
border-radius: 16px;
padding: 64px;
left: 50%;
margin-left: -256px;
}
然后,可以使用JavaScript/jQuery,根据上面的方法“实现滚动效果”。
$(document).ready(function() {
var containerTop = $('.floating-container').position().top;
var scrollOffset = $('.scrollable').offset().top;
$(window).scroll(function() {
if ($('.scrollable').scrollTop() > (containerTop - scrollOffset)) {
$('.floating-container').addClass('floating');
} else {
$('.floating-container').removeClass('floating');
}
});
});
“滚动效果”就这样实现了,整段代码:
<div class="scrollable" style="height: 500px; overflow: auto;">
<h2>可以滚动的内容</h2>
<p>这里是内容的开始...</p>
<p>这里是内容的中间...</p>
<p>这里是内容的结尾...</p>
</div>
<div class="floating-container" style="position: sticky; top: 50px;">
<h3>我是一个浮动层</h3>
<p>我一旦「起飞」,就会在您的页面中随意移动。</p>
</div>
<style>
.floating-container {
background-color: #f8f8f8;
border-radius: 16px;
padding: 64px;
left: 50%;
margin-left: -256px;
}
.floating {
position: fixed;
top: 50px;
left: 50%;
margin-left: -256px;
transform: translateY(-50%);
}
</style>
<script>
$(document).ready(function() {
var containerTop = $('.floating-container').position().top;
var scrollOffset = $('.scrollable').offset().top;
$(window).scroll(function() {
if ($('.scrollable').scrollTop() > (containerTop - scrollOffset)) {
$('.floating-container').addClass('floating');
} else {
$('.floating-container').removeClass('floating');
}
});
});
</script>
示例2:滚动到页面底部时消失
改写一点点代码,实现滚动到页面底部时隐藏“浮动层”。
$(document).ready(function() {
var containerTop = $('.floating-container').position().top;
var scrollOffset = $('.scrollable').offset().top;
$(window).scroll(function() {
if ($('.scrollable').scrollTop() > (containerTop - scrollOffset)) {
$('.floating-container').addClass('floating');
if ($('.scrollable')[0].scrollHeight - $('.scrollable').scrollTop() - $('.scrollable').height() < 50) {
$('.floating-container').addClass('fadeaway');
} else {
$('.floating-container').removeClass('fadeaway');
}
} else {
$('.floating-container').removeClass('floating');
$('.floating-container').removeClass('fadeaway');
}
});
});
当滚动到页面底部时,判断scrollHeight
、scrollTop
和height
是否符合要求(例如,差距小于50px),只有符合时才会隐藏浮动层。
<div class="scrollable" style="height: 500px; overflow: auto;">
<h2>可以滚动的内容</h2>
<p>这里是内容的开始...</p>
<p>这里是内容的中间...</p>
<p>这里是内容的结尾...</p>
</div>
<div class="floating-container" style="position: sticky; top: 50px;">
<h3>我是一个浮动层</h3>
<p>我一旦「起飞」,就会在您的页面中随意移动。</p>
</div>
<style>
.floating-container {
background-color: #f8f8f8;
border-radius: 16px;
padding: 64px;
left: 50%;
margin-left: -256px;
transition: all .3s ease-out;
opacity: 1;
/*display: block;*/
}
.floating {
position: fixed;
top: 50px;
left: 50%;
margin-left: -256px;
transform: translateY(-50%);
}
.fadeaway {
opacity: 0;
/*display: none;*/
}
</style>
<script>
$(document).ready(function() {
var containerTop = $('.floating-container').position().top;
var scrollOffset = $('.scrollable').offset().top;
$(window).scroll(function() {
if ($('.scrollable').scrollTop() > (containerTop - scrollOffset)) {
$('.floating-container').addClass('floating');
if ($('.scrollable')[0].scrollHeight - $('.scrollable').scrollTop() - $('.scrollable').height() < 50) {
$('.floating-container').addClass('fadeaway');
} else {
$('.floating-container').removeClass('fadeaway');
}
} else {
$('.floating-container').removeClass('floating');
$('.floating-container').removeClass('fadeaway');
}
});
});
</script>
本文标题为:jQuery实现div浮动层跟随页面滚动效果
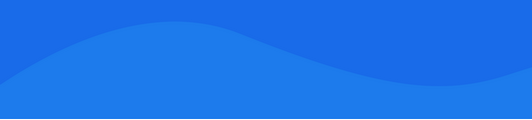
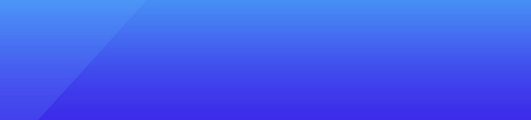
基础教程推荐
- 使用CSS的pointer-events属性实现鼠标穿透效果的神奇技巧 2024-01-23
- css3手动实现pc端横向滚动 2024-01-20
- 一文详解如何根据后端返回的url下载json文件 2024-01-04
- HTML/HTML5 基础知识 | 面试题专用 2023-10-28
- JavaScript实现移动端轮播效果 2024-01-05
- Ajax实现登录案例 2023-02-23
- 用js读写cookie的简单方法(推荐) 2024-02-05
- chrome浏览器不支持onmouseleave事件的解决技巧 2024-01-07
- 从gb到utf-8 2022-11-04
- 极酷的三层分离的标准滑动门导航菜单 2024-01-24