要实现为input与textarea自定义hover、focus效果的方法,可以用JavaScript来实现。下面是具体的步骤。
要实现为input与textarea自定义hover、focus效果的方法,可以用JavaScript来实现。下面是具体的步骤。
步骤1. 获取input或textarea元素
首先需要获取input或textarea元素,可以使用document.querySelector()方法来获取元素。比如:
const input = document.querySelector('input');
const textarea = document.querySelector('textarea');
步骤2. 添加事件监听器
接下来需要添加事件监听器,监听input或textarea的鼠标移入、移出和获取焦点、失焦事件。可以使用addEventListener()方法来添加事件监听器,比如:
input.addEventListener('mouseenter', function() {
// 鼠标移入时的操作
});
input.addEventListener('mouseleave', function() {
// 鼠标移出时的操作
});
input.addEventListener('focus', function() {
// 获取焦点时的操作
});
input.addEventListener('blur', function() {
// 失去焦点时的操作
});
步骤3. 修改元素的样式
在事件监听器中,可以修改元素的样式,从而实现自定义的hover、focus效果。比如:
input.addEventListener('mouseenter', function() {
input.style.border = '2px solid blue';
});
input.addEventListener('mouseleave', function() {
input.style.border = 'none';
});
input.addEventListener('focus', function() {
input.style.background = 'lightyellow';
});
input.addEventListener('blur', function() {
input.style.background = 'white';
});
这样就可以为input元素实现自定义的hover、focus效果了。
示例1
下面是一个简单的示例,为一个input元素添加自定义的hover、focus效果:
<!DOCTYPE html>
<html>
<head>
<title>Custom input hover/focus effect with JavaScript</title>
<style>
input {
border-radius: 5px;
padding: 5px;
font-size: 18px;
}
</style>
</head>
<body>
<input type="text" placeholder="Enter your name">
<script>
const input = document.querySelector('input');
input.addEventListener('mouseenter', function() {
input.style.border = '2px solid blue';
});
input.addEventListener('mouseleave', function() {
input.style.border = 'none';
});
input.addEventListener('focus', function() {
input.style.background = 'lightyellow';
});
input.addEventListener('blur', function() {
input.style.background = 'white';
});
</script>
</body>
</html>
示例2
下面是另一个示例,为一个textarea元素添加自定义的hover、focus效果:
<!DOCTYPE html>
<html>
<head>
<title>Custom textarea hover/focus effect with JavaScript</title>
<style>
textarea {
border-radius: 5px;
padding: 10px;
font-size: 18px;
resize: none;
}
</style>
</head>
<body>
<textarea placeholder="Enter your message"></textarea>
<script>
const textarea = document.querySelector('textarea');
textarea.addEventListener('mouseenter', function() {
textarea.style.border = '2px solid blue';
});
textarea.addEventListener('mouseleave', function() {
textarea.style.border = 'none';
});
textarea.addEventListener('focus', function() {
textarea.style.background = 'lightyellow';
});
textarea.addEventListener('blur', function() {
textarea.style.background = 'white';
});
</script>
</body>
</html>
这样就可以为textarea元素实现自定义的hover、focus效果了。
沃梦达教程
本文标题为:JavaScript实现为input与textarea自定义hover,focus效果的方法
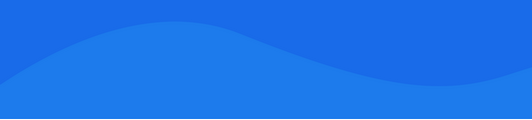
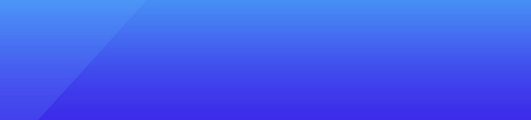
基础教程推荐
猜你喜欢
- 如何使用linux命令行发送HTML电子邮件 2023-10-25
- JavaScript用JSONP跨域请求数据实例详解 2024-02-08
- date.parse在IE和FF中的区别 2024-01-08
- vue 请求拦截request将token添加到请求头headers 2023-10-08
- CSS 完美兼容IE6/IE7/FF的通用hack方法 2023-12-20
- Select 选择器显示内容为icon图标选项(Ant Design of Vue) 2023-10-08
- VUE3.0-手写实现组合API 2023-10-08
- HTML+CSS+JS实现完美兼容各大浏览器的TABLE固定列 2023-12-21
- IE6下css设置容器高度的BUG不能小于某个值 2023-12-20
- vue插件和组件的区别 2023-10-08