制作一个发光立方体特效需要使用HTML和CSS来实现。下面是制作发光立方体特效完整攻略:
制作一个发光立方体特效需要使用HTML和CSS来实现。下面是制作发光立方体特效完整攻略:
1. HTML
首先我们需要定义一个 HTML 结构来制作立方体特效。这里使用一个 div 元素来包含 6 个面,每个面使用一个 div 元素来实现,代码如下:
<div class="cube">
<div class="cube-face cube-face-front"></div>
<div class="cube-face cube-face-back"></div>
<div class="cube-face cube-face-left"></div>
<div class="cube-face cube-face-right"></div>
<div class="cube-face cube-face-top"></div>
<div class="cube-face cube-face-bottom"></div>
</div>
2. CSS
接下来我们使用 CSS 来控制这个立方体的样式和动态效果。代码如下:
.cube {
position: relative;
width: 200px;
height: 200px;
transform-style: preserve-3d;
animation: spin 5s infinite;
}
.cube-face {
position: absolute;
width: 200px;
height: 200px;
background: #FFF;
border: 5px solid #333;
}
.cube-face-front {
transform: translateZ(100px);
}
.cube-face-back {
transform: translateZ(-100px);
}
.cube-face-left {
transform: rotateY(-90deg) translateZ(100px);
}
.cube-face-right {
transform: rotateY(90deg) translateZ(100px);
}
.cube-face-top {
transform: rotateX(-90deg) translateZ(100px);
}
.cube-face-bottom {
transform: rotateX(90deg) translateZ(100px);
}
@keyframes spin {
0% { transform: rotateY(0deg); }
100% { transform: rotateY(360deg); }
}
运行后,可以看到出现了一个立方体,但是还没有实现发光的特效。
3. 给立方体增加发光效果
要为立方体增加发光效果,我们可以使用 box-shadow
属性。首先,我们需要为每个面都添加一个背景发光层和边框发光层。
.cube-face {
position: absolute;
width: 200px;
height: 200px;
/* 添加背景发光层和边框发光层 */
box-shadow: inset 0 0 40px rgba(255,255,255,0.5), 0 0 50px rgba(255,255,255,0.5);
border: 5px solid #333;
}
这里我们使用 box-shadow
属性为每个面添加背景发光层和边框发光层。然后我们再为整个立方体添加一个外部发光层。
.cube {
position: relative;
width: 200px;
height: 200px;
transform-style: preserve-3d;
animation: spin 5s infinite;
/* 添加外部发光层 */
box-shadow: 0 0 50px rgba(255,255,255,0.5);
}
这样立方体就拥有了发光的特效了。可以给立方体添加其他样式来美化,例如给立方体添加背景颜色,可以增加一个图片作为背景,等等。
示例说明
示例 1
下面是一个基于以上代码的示例。可以通过修改代码来尝试不同的效果:
<div class="cube">
<div class="cube-face cube-face-front"></div>
<div class="cube-face cube-face-back"></div>
<div class="cube-face cube-face-left"></div>
<div class="cube-face cube-face-right"></div>
<div class="cube-face cube-face-top"></div>
<div class="cube-face cube-face-bottom"></div>
</div>
<style>
.cube {
position: relative;
width: 200px;
height: 200px;
transform-style: preserve-3d;
animation: spin 5s infinite;
box-shadow: 0 0 50px rgba(255,255,255,0.5);
background-color: #f6f6f6;
}
.cube-face {
position: absolute;
width: 200px;
height: 200px;
box-shadow: inset 0 0 40px rgba(255,255,255,0.5), 0 0 50px rgba(255,255,255,0.5);
border: 5px solid #333;
}
.cube-face-front {
transform: translateZ(100px);
}
.cube-face-back {
transform: translateZ(-100px);
}
.cube-face-left {
transform: rotateY(-90deg) translateZ(100px);
}
.cube-face-right {
transform: rotateY(90deg) translateZ(100px);
}
.cube-face-top {
transform: rotateX(-90deg) translateZ(100px);
}
.cube-face-bottom {
transform: rotateX(90deg) translateZ(100px);
}
@keyframes spin {
0% { transform: rotateY(0deg); }
100% { transform: rotateY(360deg); }
}
</style>
示例 2
下面是一个基于以上代码的另一个示例。这个示例为立方体添加了一个背景图片,并调整了立方体的颜色和发光效果。
<div class="cube">
<div class="cube-face cube-face-front"></div>
<div class="cube-face cube-face-back"></div>
<div class="cube-face cube-face-left"></div>
<div class="cube-face cube-face-right"></div>
<div class="cube-face cube-face-top"></div>
<div class="cube-face cube-face-bottom"></div>
</div>
<style>
.cube {
position: relative;
width: 200px;
height: 200px;
transform-style: preserve-3d;
animation: spin 5s infinite;
box-shadow: 0 0 50px rgba(255,255,255,0.7);
background-image: url('https://cdn.pixabay.com/photo/2015/06/08/15/02/pencils-802734_1280.jpg');
}
.cube-face {
position: absolute;
width: 200px;
height: 200px;
box-shadow: inset 0 0 40px rgba(255,255,255,0.5), 0 0 50px rgba(255,255,255,0.7);
border: 5px solid #555;
}
.cube-face-front {
transform: translateZ(100px);
background-color: #ffcc33;
}
.cube-face-back {
transform: translateZ(-100px);
background-color: #ffb347;
}
.cube-face-left {
transform: rotateY(-90deg) translateZ(100px);
background-color: #ff7f50;
}
.cube-face-right {
transform: rotateY(90deg) translateZ(100px);
background-color: #ff6347;
}
.cube-face-top {
transform: rotateX(-90deg) translateZ(100px);
background-color: #ff7f24;
}
.cube-face-bottom {
transform: rotateX(90deg) translateZ(100px);
background-color: #ff4616;
}
@keyframes spin {
0% { transform: rotateY(0deg); }
100% { transform: rotateY(360deg); }
}
</style>
以上就是制作发光立方体特效的完整攻略和两条示例说明。
本文标题为:使用html+css制作一个发光立方体特效
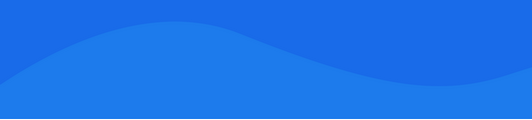
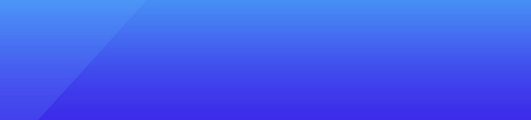
基础教程推荐
- 在IE中为abbr标签加样式 2022-10-16
- vue面试题 2023-10-08
- 使用flutter创建可移动的stack小部件功能 2023-08-08
- jquery实现弹出窗口效果的实例代码 2023-12-20
- 微信小程序 Page()函数详解 2024-01-04
- JS弹出新窗口被拦截的解决方法 2024-01-07
- JS循环中正确使用async、await的姿势分享 2023-08-12
- 小心:CSS代码书写顺序不同,导致显示效果不一样 2022-11-04
- 大学生网页设计作业的20款优秀HTML5制作工具 2023-10-28
- linux下html文件在浏览器中的显示乱码 2023-10-29