JS使用cookie实现DIV提示框只显示一次的方法可以分为以下几个步骤:
JS使用cookie实现DIV提示框只显示一次的方法可以分为以下几个步骤:
- 判断cookie是否存在
- 如果cookie不存在,则显示DIV提示框,并设置cookie
- 如果cookie存在,则不显示DIV提示框
具体步骤如下:
- 判断cookie是否存在:
function getCookie(name) {
var arr = document.cookie.match(new RegExp("(^| )" + name + "=([^;]*)(;|$)"));
if (arr != null) {
return unescape(arr[2]);
}
return null;
}
var isShow = getCookie("isShow");
if (isShow != null && isShow == "false") {
// do not show div
} else {
// show div
}
- 如果cookie不存在,则显示DIV提示框,并设置cookie:
function setCookie(name, value, day) {
var expires = "";
if (day) {
var date = new Date();
date.setTime(date.getTime() + day * 24 * 60 * 60 * 1000);
expires = "; expires=" + date.toUTCString();
}
document.cookie = name + "=" + escape(value) + expires + "; path=/";
}
var isShow = getCookie("isShow");
if (isShow == null) {
// show div
setCookie("isShow", "false", 30); // 30 days
}
- 如果cookie存在,则不显示DIV提示框:
var isShow = getCookie("isShow");
if (isShow != null && isShow == "false") {
// do not show div
} else {
// show div
}
以下是两个示例:
- 示例一:
<div id="tip" style="display:none;">This is a div tip.</div>
<script>
function getCookie(name) {
var arr = document.cookie.match(new RegExp("(^| )" + name + "=([^;]*)(;|$)"));
if (arr != null) {
return unescape(arr[2]);
}
return null;
}
function setCookie(name, value, day) {
var expires = "";
if (day) {
var date = new Date();
date.setTime(date.getTime() + day * 24 * 60 * 60 * 1000);
expires = "; expires=" + date.toUTCString();
}
document.cookie = name + "=" + escape(value) + expires + "; path=/";
}
var isShow = getCookie("isShow");
if (isShow == null) {
// show div
setCookie("isShow", "false", 30); // 30 days
document.getElementById("tip").style.display = "block";
}
</script>
- 示例二:
<div id="tip" style="display:none;">This is a div tip.</div>
<script>
function getCookie(name) {
var arr = document.cookie.match(new RegExp("(^| )" + name + "=([^;]*)(;|$)"));
if (arr != null) {
return unescape(arr[2]);
}
return null;
}
var isShow = getCookie("isShow");
if (isShow != null && isShow == "false") {
// do not show div
} else {
// show div
document.getElementById("tip").style.display = "block";
}
document.getElementById("close-btn").onclick = function() {
document.getElementById("tip").style.display = "none";
setCookie("isShow", "false", 30); // 30 days
};
</script>
在示例二中,还增加了一个点击关闭按钮后设置cookie的功能,确保用户可以关闭DIV提示框之后不再显示。
沃梦达教程
本文标题为:JS使用cookie实现DIV提示框只显示一次的方法
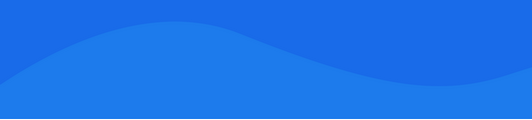
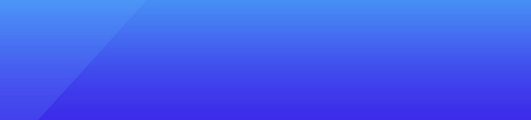
基础教程推荐
猜你喜欢
- Photoshop CC给前端开发者怎样的体验?新特性介绍 2024-01-23
- docker 进程 转载:https://www.cnblogs.com/ilinuxer/p/6188303.html 2023-10-25
- jquery photoFrame 图片边框美化显示插件 2024-01-21
- AngularJS中使用HTML5手机摄像头拍照 2024-01-05
- flex(弹性布局)教程之常用布局 2022-11-20
- jQuery实现单击和鼠标感应事件 2024-01-20
- 网页选项卡TAB设计原则和应用案例教程 2024-03-10
- 用JS做的简单的可折叠的两级树形菜单 2024-01-06
- 解决uniapp下载视频,使用uni.downloadFile下载大文件会出现下载到一半就停止问题 2023-08-29
- CSS3贝塞尔曲线示例:创建链接悬停动画效果 2023-12-22