在Vue.js中,Vue Router是一个常用的路由管理器。Vue Router可以实现单页应用(SPA)的路由功能。在Vue Router中,有两种路由模式:hash模式和history模式。在本文中,我们将详细介绍这两种模式的区别和使用方法。
Vue Router路由hash模式与history模式详细介绍
在Vue.js中,Vue Router是一个常用的路由管理器。Vue Router可以实现单页应用(SPA)的路由功能。在Vue Router中,有两种路由模式:hash模式和history模式。在本文中,我们将详细介绍这两种模式的区别和使用方法。
hash模式
hash模式是Vue Router的默认模式,在URL中使用“#”作为路由路径的锚点标记,不会触发页面的刷新,也不会向服务器发送请求。通过监听URL的变化,从而实现页面的路由切换。在Vue Router中,我们可以通过配置路由的mode参数来设置使用hash模式。
配置方法
const router = new VueRouter({
mode: 'hash',
routes: [...]
})
示例
假设我们有两个路由,一个是首页(/),一个是关于我们(/about)。
// main.js
import Vue from 'vue'
import App from './App.vue'
import VueRouter from 'vue-router'
import Home from './components/Home.vue'
import About from './components/About.vue'
Vue.config.productionTip = false
Vue.use(VueRouter)
const routes = [
{
path: '/',
component: Home
},
{
path: '/about',
component: About
}
]
const router = new VueRouter({
mode: 'hash',
routes
})
new Vue({
render: h => h(App),
router
}).$mount('#app')
现在我们在App.vue中添加路由跳转的链接:
<template>
<div>
<router-link to="/">Home</router-link>
<router-link to="/about">About</router-link>
<router-view></router-view>
</div>
</template>
在浏览器中输入localhost:8080/#/,可以看到页面显示为Home。点击About的链接,页面会跳转到localhost:8080/#/about,显示为About。
history模式
history模式使用浏览器的history API来实现路由功能,将页面路由信息保存在浏览器的history栈中,可以使用前进、后退按钮切换路由,也可以通过URL直接访问页面。
配置方法
const router = new VueRouter({
mode: 'history',
routes: [...]
})
需要注意的是,使用history模式需要服务器端支持,否则会出现404错误。如果要在开发环境中使用history模式,可以使用vue-cli提供的history模式的dev server。启动dev server的方法如下:
npm run dev -- --history
示例
假设我们有两个路由,一个是首页(/),一个是关于我们(/about)。
// main.js
import Vue from 'vue'
import App from './App.vue'
import VueRouter from 'vue-router'
import Home from './components/Home.vue'
import About from './components/About.vue'
Vue.config.productionTip = false
Vue.use(VueRouter)
const routes = [
{
path: '/',
component: Home
},
{
path: '/about',
component: About
}
]
const router = new VueRouter({
mode: 'history',
routes
})
new Vue({
render: h => h(App),
router
}).$mount('#app')
现在我们在App.vue中添加路由跳转的链接:
<template>
<div>
<router-link to="/">Home</router-link>
<router-link to="/about">About</router-link>
<router-view></router-view>
</div>
</template>
在浏览器中输入localhost:8080,可以看到页面显示为Home。点击About的链接,页面会跳转到localhost:8080/about,显示为About。
以上是Vue Router路由hash模式与history模式的详细介绍。通过本文的学习,相信你已经掌握了这两种路由模式的使用方法。
本文标题为:Vue Router路由hash模式与history模式详细介绍
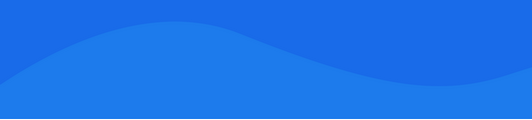
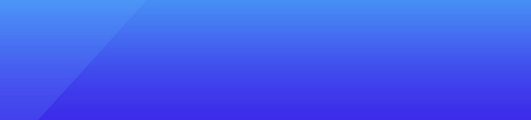
基础教程推荐
- ExtJS 3.x DateField menuListeners 显示/隐藏 2022-09-15
- Vue优化篇-2.防抖节流 2023-10-08
- Ajax请求超时与网络异常处理图文详解 2023-02-23
- 原生ajax瀑布流demo分享(必看篇) 2023-02-01
- c# – 使用HTML5捕获签名并将其作为映像保存到数据库 2023-10-26
- ES6新特性六:promise对象实例详解 2024-02-07
- 原生JS实现LOADING效果 2023-12-01
- javascript实现点击单选按钮链接转向对应网址的方法 2024-02-06
- Ajax跨域访问Cookie丢失问题的解决方法 2023-01-26
- TS如何从目录中提取所有指定扩展名的文件 2023-07-09