下面是针对“Vue实现内容可滚动的弹窗效果”的完整攻略。
下面是针对“Vue实现内容可滚动的弹窗效果”的完整攻略。
1. 弹窗样式
首先,我们需要用HTML、CSS实现出弹窗的样式,示例代码如下:
<div class="modal">
<div class="modal__body">
<div class="modal__header">
<h2 class="modal__title">弹窗标题</h2>
<button class="modal__close-btn">×</button>
</div>
<div class="modal__content">
<p>弹窗内容</p>
</div>
</div>
</div>
.modal {
position: fixed;
z-index: 9999;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: rgba(0, 0, 0, 0.5);
display: flex;
justify-content: center;
align-items: center;
}
.modal__body {
position: relative;
width: 80%;
max-height: 80%;
border-radius: 10px;
background-color: #fff;
overflow-y: scroll;
-webkit-overflow-scrolling: touch;
}
.modal__header {
padding: 20px;
border-bottom: 1px solid #eee;
}
.modal__title {
margin: 0;
font-size: 20px;
font-weight: bold;
}
.modal__close-btn {
position: absolute;
top: 10px;
right: 10px;
padding: 5px;
font-size: 24px;
border: none;
background-color: transparent;
cursor: pointer;
}
.modal__content {
padding: 20px;
}
在这段代码中,我们定义了一个 modal
类,用于覆盖整个屏幕,并设置了半透明的黑色背景。然后在其中定义了一个 modal__body
类,用来包含弹窗内容并在其中设置了最大高度为80%,当内容超过该高度时会出现纵向滚动条。
2. 弹窗组件
接下来,我们需要将弹窗作为一个Vue组件来实现。示例代码如下:
<template>
<div class="modal" v-show="visible">
<div class="modal__body">
<div class="modal__header">
<h2 class="modal__title">{{ title }}</h2>
<button class="modal__close-btn" @click="close">×</button>
</div>
<div class="modal__content">
<slot></slot>
</div>
</div>
</div>
</template>
<script>
export default {
name: 'Modal',
props: {
title: {
type: String,
default: '弹窗标题'
}
},
data() {
return {
visible: false
}
},
methods: {
show() {
this.visible = true
document.body.style.overflow = 'hidden'
},
close() {
this.visible = false
document.body.style.overflow = 'auto'
}
},
mounted() {
document.addEventListener('keydown', (e) => {
if (e.keyCode === 27 && this.visible) {
this.visible = false
document.body.style.overflow = 'auto'
}
})
},
destroyed() {
document.body.style.overflow = 'auto'
}
}
</script>
<style>
/* 同上 */
</style>
在这个组件中,我们使用了Vue的插槽功能来动态渲染弹窗内容,在 props
中定义了一个 title
属性,并在组件的 data
属性中定义了一个 visible
属性来控制弹窗的显示和隐藏。在 mounted
钩子函数中监听 keydown
事件,用于在按下ESC键时关闭弹窗。
3. 引入外部组件
当然,我们也可以通过引入第三方组件来实现内容可滚动的弹窗。以 vue-js-modal
为例,我们可以这样使用它:
<template>
<div>
<button @click="showModal">弹出窗口</button>
<modal name="my-modal" height="80%">
<h2>弹窗标题</h2>
<div>弹窗内容</div>
</modal>
</div>
</template>
<script>
import VModal from 'vue-js-modal'
export default {
name: 'MyComponent',
methods: {
showModal() {
this.$modal.show('my-modal')
}
},
components: {
Modal: VModal
}
}
</script>
在这个代码片段中,我们使用了 vue-js-modal
提供的 Modal
组件,并通过 height
属性设置了弹窗的最大高度为80%。我们可以像使用其他组件一样使用它,并在需要时调用 $modal.show
方法弹出窗口。
以上就是关于Vue实现内容可滚动的弹窗效果的完整攻略,希望能对您有所帮助!
本文标题为:vue实现内容可滚动的弹窗效果
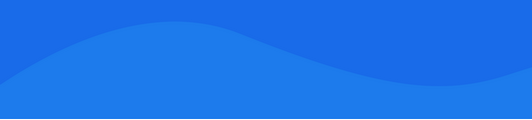
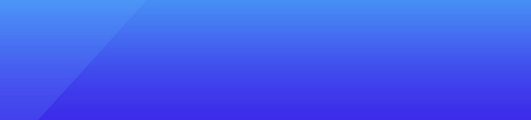
基础教程推荐
- css锚点定位被顶部固定导航栏遮住的解决方案 2023-12-22
- Nodejs 连接 mysql时报Error: Cannot enqueue Query after fatal error错误的处理办法 2023-07-09
- 关于JS Lodop打印插件打印Bootstrap样式错乱问题的解决方案 2024-01-04
- Layui在table中使用select下拉框 2022-10-20
- vue实现鼠标经过显示悬浮框效果 2024-01-21
- 浅谈vue项目打包优化策略 2024-03-09
- vue任意关系组件通信与跨组件监听状态vue-communication 2024-01-05
- 第6天:XHTML代码规范 2022-11-04
- 在CHtmlView中指定IE版本 2023-10-28
- 瀑布流布局的两种实现方式:传统多列浮动和绝对定位布局 2024-01-24