当在webpack中引入jquery.mCustomScrollbar插件时,需要进行一些特殊处理。以下是详细的步骤:
当在webpack中引入jquery.mCustomScrollbar插件时,需要进行一些特殊处理。以下是详细的步骤:
1. 安装jQuery和jQuery.mCustomScrollbar插件
首先,在项目中安装需要使用到的jQuery库和jQuery.mCustomScrollbar插件。可以通过npm安装,执行以下命令:
npm install jquery@mcustomscrollbar/jquery.mcustomscrollbar --save
注意到这个插件使用了jQuery,所以在下载之前要先安装jQuery。
2. 在webpack的入口文件中引入依赖库
在项目的入口文件index.js
中,引入依赖库。可以将jQuery和插件的实例保存到全局变量中。以下是一个示例:
import $ from 'jquery';
import 'jquery.mcustomscrollbar';
window.$ = $;
window.jQuery = $;
const options = {
axis:"y",
theme:"dark"
};
$( document ).ready(function() {
$('#my-div-scrollbar').mCustomScrollbar(options);
});
此时我们已经将jQuery和插件的实例保存到全局变量中,使插件可以在其他文件中通过全局变量使用。
3. 在webpack的配置文件中,对jquery和jquery.mCustomScrollbar进行处理。
在webpack的配置文件中,需要使用webpack.ProvidePlugin
来自动加载jQuery和插件。
const webpack = require('webpack');
module.exports = {
plugins: [
new webpack.ProvidePlugin({
$: 'jquery',
jQuery: 'jquery'
})
]
}
这样就可以确保在任意文件中,都可以使用jQuery和插件了。如果我们需要修改插件的配置,可以通过传递一个对象给mCustomScrollbar
函数进行配置。
在下面,我们来看两个具体的使用示例:
示例一:添加滚动条
以下代码展示如何在一个容器块中添加定制自己风格的滚动条。
<div id="my-div-scrollbar" style="height: 300px; width: 200px;">
<p>这是要添加滚动条的文本内容</p>
</div>
import $ from 'jquery';
import 'jquery.mcustomscrollbar';
window.$ = $;
window.jQuery = $;
const options = {
axis:"y",
theme:"dark"
};
$( document ).ready(function() {
$('#my-div-scrollbar').mCustomScrollbar(options);
});
示例二:嵌套使用
有时候,我们需要在mCustomScrollbar
里嵌套使用jQueryUI或者其他插件。以下代码展示如何实现这点。
<div id="my-div-scrollbar">
<ul>
<li>
<div class="ui-widget">
<h3>jQuery UI Accordion - Default functionality</h3>
<div>
<p>
当前部分中的内容, 无需滚动条,因为容器已经设置成了高度自适应。
</p>
</div>
</div>
</li>
<li>
<div class="ui-widget">
<h3>jQuery UI Accordion - Custom animation</h3>
<div>
<p>
当前部分中的内容也是需要滚动条的。
</p>
</div>
</div>
</li>
<li>
<div class="ui-widget">
<h3>jQuery UI Accordion - Fill space</h3>
<div>
<p>
当前部分也需要滚动条。
</p>
</div>
</div>
</li>
</ul>
</div>
引入jQueryUI,并对mCustomScrollbar
进行初始化:
import $ from 'jquery';
import 'jquery.mcustomscrollbar';
import 'jquery-ui-bundle';
window.$ = $;
window.jQuery = $;
$(function() {
$( "#accordion" ).accordion();
});
$('.ui-widget').mCustomScrollbar({
theme:"dark-3",
axis: "y"
});
至此,我们已经完成了使用jquery.mCustomScrollbar插件的全部配置过程。
本文标题为:webpack里使用jquery.mCustomScrollbar插件的方法
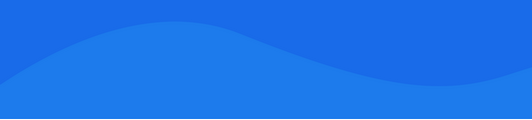
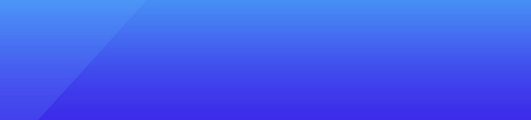
基础教程推荐
- Ajax异步检查用户名是否存在 2023-02-14
- 一款纯css3实现的非常实用的鼠标悬停特效演示 2024-01-23
- uniapp打包app提示通讯录权限问题,如何取消通讯录权限 2022-10-29
- 格式png24透明底 多种解决png24格式图片在ie6中透明问题 2024-04-02
- 浅谈javascript中onbeforeunload与onunload事件 2024-01-04
- IE6,IE7和firefox对DIV的支持区别 2023-12-21
- 基于CSS3的CSS 多栏(Multi-column)实现瀑布流源码分享 2024-04-02
- AngularJs Using $location详解及示例代码 2024-02-11
- LocalStorage记住用户和密码功能 2022-09-16
- CSS三栏布局探讨——中间固定宽度两边自适应宽度 2024-03-09