制作一个完整的表格需要用到AngularJS中的一些重要概念和指令。以下是详细的攻略:
制作一个完整的表格需要用到AngularJS中的一些重要概念和指令。以下是详细的攻略:
1. 设置AngularJS应用
在HTML文件中,使用ng-app
指令来定义一个AngularJS应用。例如:
<html ng-app="myApp">
其中,myApp
是定义的应用名称。
2. 定义AngularJS模块
使用angular.module()
函数来定义AngularJS模块。例如:
var app = angular.module('myApp', []);
其中,myApp
是定义的应用名称,[]
里面可以放置要加载的其他模块。
3. 定义数据模型
可以使用AngularJS中的$http
服务向服务器请求数据,从而得到数据模型。例如:
app.controller('myCtrl', function($scope, $http) {
$http.get("url-to-data-source")
.then(function(response) {
$scope.myData = response.data;
});
});
其中,myCtrl
是定义的控制器名称,$http
是AngularJS中的服务,可以通过调用其get()
方法向服务器发送GET请求。
4. 创建表格
通过使用ng-repeat
指令来创建表格。例如:
<table>
<thead>
<tr>
<th ng-repeat="header in headers">{{header}}</th>
</tr>
</thead>
<tbody>
<tr ng-repeat="data in myData">
<td>{{data.name}}</td>
<td>{{data.age}}</td>
<td>{{data.gender}}</td>
</tr>
</tbody>
</table>
其中,headers
是表头,myData
是控制器中定义的数据模型。
5. 添加样式
可以为表格添加样式,例如:
<table class="table table-striped">
其中,table
和table-striped
是Bootstrap框架中的CSS样式。
以下是一个完整示例:
var app = angular.module('myApp', []);
app.controller('myCtrl', function($scope, $http) {
$http.get("url-to-data-source")
.then(function(response) {
$scope.myData = response.data;
});
});
<html ng-app="myApp">
<head>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootswatch/4.5.2/slate/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular.min.js"></script>
<script src="app.js"></script>
</head>
<body>
<div ng-controller="myCtrl">
<table class="table table-striped">
<thead>
<tr>
<th ng-repeat="header in headers">{{header}}</th>
</tr>
</thead>
<tbody>
<tr ng-repeat="data in myData">
<td>{{data.name}}</td>
<td>{{data.age}}</td>
<td>{{data.gender}}</td>
</tr>
</tbody>
</table>
</div>
</body>
</html>
以上示例中,表格中的数据模型可以从url-to-data-source
获取,使用的是Bootstrap框架中的table
和table-striped
样式。
本文标题为:如何用angularjs制作一个完整的表格
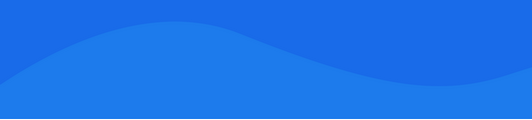
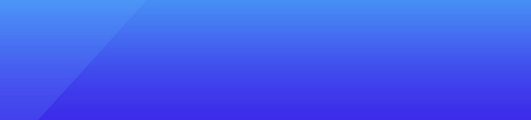
基础教程推荐
- vue3项目中使用tinymce的方法 2024-01-05
- Vue SPA 首屏优化方案 2024-03-09
- 关于 vue.js:在 weex 中初始化一个全局 mixin 2022-09-16
- 详解iframe跨域的几种常用方法(小结) 2024-01-07
- 微信小程序 教程之事件 2024-01-08
- ubuntu nginx修改根目录 访问html页面 2023-10-26
- JavaScript网页制作特殊效果用随机数 2024-04-09
- 利用JS将图标字体渲染为图片的方法详解 2024-04-09
- 利用AJAX实现无刷新数据分页 2022-12-28
- this[] 指的是什么内容 讨论 2023-11-30