要将字符串中由空格隔开的每个单词首字母大写,可以使用字符串操作方法和正则表达式。
要将字符串中由空格隔开的每个单词首字母大写,可以使用字符串操作方法和正则表达式。
步骤如下:
- 首先要将字符串进行拆分,将每个单词分离。可以使用split()方法,该方法可以按照指定字符或正则表达式对字符串进行分割,返回一个由分割出来的子字符串组成的数组。
例如:
let str = "hello world";
let arr = str.split(" ");
console.log(arr); // ["hello", "world"]
- 然后遍历这个数组,对每个单词的首字母进行大写处理。可以使用for循环或forEach()方法进行遍历,结合正则表达式,将每个单词的首字母提取出来,然后进行大写处理。
例如:
let str = "hello world";
let arr = str.split(" ");
arr.forEach(function(word, index) {
arr[index] = word.replace(/^\w/, function(match) {
return match.toUpperCase();
});
});
console.log(arr); // ["Hello", "World"]
在这个例子中,使用了正则表达式的replace()方法,将每个单词的首字母提取出来并转换为大写。
- 最后再将这些单词重新组合成一个新的字符串。可以使用join()方法将数组转为字符串,并指定连接符。
例如:
let str = "hello world";
let arr = str.split(" ");
arr.forEach(function(word, index) {
arr[index] = word.replace(/^\w/, function(match) {
return match.toUpperCase();
});
});
let newStr = arr.join(" ");
console.log(newStr); // "Hello World"
这个新字符串就是将原来字符串中的每个单词的首字母都大写了的字符串。
示例说明:
假设原字符串为 "hello world",使用上述攻略进行处理后,得到的新字符串为 "Hello World"。
再假设原字符串为 "i love coding",使用上述攻略进行处理后,得到的新字符串为 "I Love Coding"。
沃梦达教程
本文标题为:将字符串中由空格隔开的每个单词首字母大写
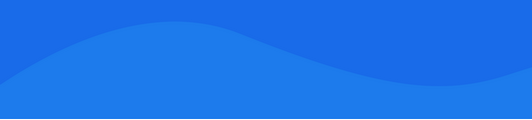
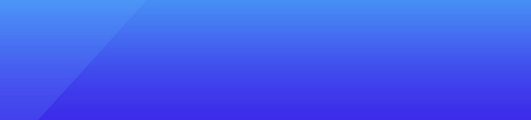
基础教程推荐
猜你喜欢
- div可以输入内容不用input作为输入框屏蔽自动的input样式 2024-01-21
- 用css alpha 滤镜 实现input file 样式美化代码 2024-03-13
- js常用排序实现代码 2023-12-01
- 详细谈谈JS中的内存与变量存储 2023-12-03
- CSS的expression使用简介 2022-10-16
- 关于JavaScript的Array数组方法详解 2023-07-09
- jQuery Validator验证Ajax提交表单的方法和Ajax传参的方法 2023-02-14
- HTML——学成网制作 2023-10-28
- CSS3实现动态翻牌效果 仿百度贴吧3D翻牌一次动画特效 2022-11-13
- ASP.NET MVC 3实现访问统计系统 2024-01-04