今天做项目的时候需要通过element-ui
做一个导入excel
并解析成json
数据,下面给大家介绍一下基本代码!
<template>
<div class="app-container">
<upload-excel-component
:on-success="handleSuccess"
:before-upload="beforeUpload"
/>
<el-table
:data="tableData"
border
highlight-current-row
style="width: 100%;margin-top:20px;"
>
<el-table-column
v-for="item of tableHeader"
:key="item"
:prop="item"
:label="item"
/>
</el-table>
</div>
</template>
<script>
import uploadExcelComponent from "@/components/UploadExcel.vue";
export default {
name: "UploadExcel",
components: { uploadExcelComponent },
data() {
return {
tableData: [],
tableHeader: []
};
},
methods: {
beforeUpload(file) {
const isLt1M = file.size / 1024 / 1024 < 1;
if (isLt1M) {
return true;
}
this.$message({
message: "Please do not upload files larger than 1m in size.",
type: "warning"
});
return false;
},
//成功后获取数据
handleSuccess({ results, header }) {
console.log("results=====", results);
this.tableData = results;
this.tableHeader = header;
}
}
};
</script>
把导入解析封装到UploadExcel里面
<template>
<div>
<input
ref="excel-upload-input"
class="excel-upload-input"
type="file"
accept=".xlsx, .xls"
@change="handleClick"
/>
<div
class="drop"
@drop="handleDrop"
@dragover="handleDragover"
@dragenter="handleDragover"
>
Drop excel file here or
<el-button
:loading="loading"
style="margin-left:16px;"
size="mini"
type="primary"
@click="handleUpload"
>
Browse
</el-button>
</div>
</div>
</template>
<script>
import XLSX from "xlsx";
export default {
props: {
beforeUpload: Function, // eslint-disable-line
onSuccess: Function // eslint-disable-line
},
data() {
return {
loading: false,
excelData: {
header: null,
results: null
}
};
},
methods: {
generateData({ header, results }) {
this.excelData.header = header;
this.excelData.results = results;
this.onSuccess && this.onSuccess(this.excelData);
},
handleDrop(e) {
e.stopPropagation();
e.preventDefault();
if (this.loading) return;
const files = e.dataTransfer.files;
if (files.length !== 1) {
this.$message.error("Only support uploading one file!");
return;
}
const rawFile = files[0]; // only use files[0]
if (!this.isExcel(rawFile)) {
this.$message.error(
"Only supports upload .xlsx, .xls, .csv suffix files"
);
return false;
}
this.upload(rawFile);
e.stopPropagation();
e.preventDefault();
},
handleDragover(e) {
e.stopPropagation();
e.preventDefault();
e.dataTransfer.dropEffect = "copy";
},
handleUpload() {
this.$refs["excel-upload-input"].click();
},
handleClick(e) {
const files = e.target.files;
const rawFile = files[0]; // only use files[0]
if (!rawFile) return;
this.upload(rawFile);
},
upload(rawFile) {
this.$refs["excel-upload-input"].value = null; // fix can't select the same excel
if (!this.beforeUpload) {
this.readerData(rawFile);
return;
}
const before = this.beforeUpload(rawFile);
if (before) {
this.readerData(rawFile);
}
},
readerData(rawFile) {
this.loading = true;
return new Promise((resolve, reject) => {
const reader = new FileReader();
reader.onload = e => {
const data = e.target.result;
const workbook = XLSX.read(data, { type: "array" });
const firstSheetName = workbook.SheetNames[0];
const worksheet = workbook.Sheets[firstSheetName];
const header = this.getHeaderRow(worksheet);
const results = XLSX.utils.sheet_to_json(worksheet);
this.generateData({ header, results });
this.loading = false;
resolve();
};
reader.readAsArrayBuffer(rawFile);
});
},
getHeaderRow(sheet) {
const headers = [];
const range = XLSX.utils.decode_range(sheet["!ref"]);
let C;
const R = range.s.r;
/* start in the first row */
for (C = range.s.c; C <= range.e.c; ++C) {
/* walk every column in the range */
const cell = sheet[XLSX.utils.encode_cell({ c: C, r: R })];
/* find the cell in the first row */
let hdr = "UNKNOWN " + C; // <-- replace with your desired default
if (cell && cell.t) hdr = XLSX.utils.format_cell(cell);
headers.push(hdr);
}
return headers;
},
isExcel(file) {
return /\.(xlsx|xls|csv)$/.test(file.name);
}
}
};
</script>
<style scoped>
.excel-upload-input {
display: none;
z-index: -9999;
}
.drop {
border: 2px dashed #bbb;
width: 600px;
height: 160px;
line-height: 160px;
margin: 0 auto;
font-size: 24px;
border-radius: 5px;
text-align: center;
color: #bbb;
position: relative;
}
</style>
这样应该可以了!
以上是编程学习网小编为您介绍的“vuejs导入excel(把excel表格解析成JSON数据)”的全面内容,想了解更多关于 vuejs 内容,请继续关注编程基础学习网。
沃梦达教程
本文标题为:vuejs导入excel(把excel表格解析成JSON数据)
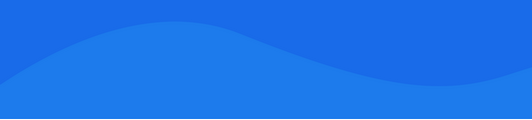
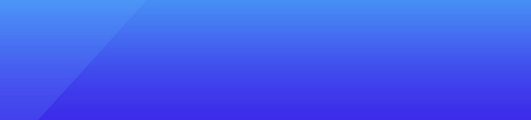
基础教程推荐
猜你喜欢
- Ajax异步刷新功能及简单案例 2023-02-24
- HTML 表单到 ExtJS 表单 2022-09-15
- Bootstrap组件系列之福利篇几款好用的组件(推荐) 2024-01-22
- 前端(HTML)+后端(Django)+数据库(MySQL):用户注册及登录演示 2023-10-26
- Ajax+Servlet实现无刷新下拉联动效果 2023-02-14
- Vue实现Tab标签路由效果并用Animate.css做转场动画效果的代码第2/3页 2024-03-31
- For循环中分号隔开的3部分的执行顺序探讨 2024-01-07
- 简述Angular 5 快速入门 2024-04-15
- 详解微信小程序开发聊天室—实时聊天,支持图片预览 2024-04-23
- 关于 vue.js:在 weex 中初始化一个全局 mixin 2022-09-16