Asynchronous iterator Tasklt;IEnumerablelt;Tgt;gt;(异步迭代器任务lt;IEnumerablelt;Tgt;gt;)
问题描述
我正在尝试实现一个返回迭代器的异步函数.思路如下:
I’m trying to implement an asynchronous function that returns an iterator. The idea is the following:
private async Task<IEnumerable<char>> TestAsync(string testString)
{
foreach (char c in testString.ToCharArray())
{
// do other work
yield return c;
}
}
但是,由于 Task
不是迭代器接口类型,因此有错误消息指出该函数不能是迭代器块.有解决办法吗?
However, there is an error message that the function cannot be an iterator block because Task<IEnumerable<char>>
is not an iterator interface type. Is there a solution?
推荐答案
听起来你可能真正想要的是 IObservable<T>
,有点像基于推送的异步 IEnumerable<T>代码>.查看 Reactive Extensions,又名 Rx(在 MIT 许可的代码)(无从属关系)了解大量方法与
IObservable<T>
一起工作,使其像 LINQ-to-Objects 等一样工作.
It sounds like what you may really be looking for is something like IObservable<T>
, which is sort of like a push-based asynchronous IEnumerable<T>
. See Reactive Extensions, a.k.a. Rx (code licensed under MIT) (no affiliation) for a huge host of methods that work with IObservable<T>
to make it work like LINQ-to-Objects and more.
IEnumerable<T>
的问题在于,没有什么可以真正使枚举本身异步.如果您不想添加对 Rx 的依赖(这确实是 IObservable<T>
大放异彩的原因),这个替代方案可能适合您:
The problem with IEnumerable<T>
is that there's nothing that really makes the enumeration itself asynchronous. If you don't want to add a dependency on Rx (which is really what makes IObservable<T>
shine), this alternative might work for you:
public async Task<IEnumerable<char>> TestAsync(string testString)
{
return GetChars(testString);
}
private static IEnumerable<char> GetChars(string testString)
{
foreach (char c in testString.ToCharArray())
{
// do other work
yield return c;
}
}
虽然我想指出的是,在不知道异步实际上做什么的情况下,可能有更好的方法来实现您的目标.您发布的所有代码实际上都不会异步执行任何操作,而且我真的不知道 //do other work
中的任何内容是否是异步的(在这种情况下,这不是您底层的解决方案问题虽然它会让你的代码编译).
though I'd like to point out that without knowing what's actually being done asynchronously, there may be a much better way to accomplish your goals. None of the code you posted will actually do anything asynchronously, and I don't really know if anything in // do other work
is asynchronous (in which case, this isn't a solution to your underlying problem though it will make your code compile).
这篇关于异步迭代器任务<IEnumerable<T>>的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:异步迭代器任务<IEnumerable<T>&
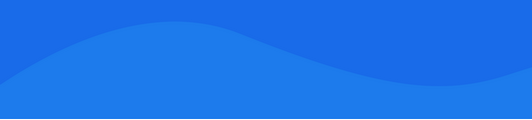
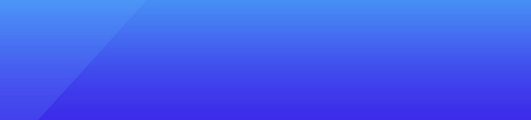
基础教程推荐
- 有没有办法忽略 2GB 文件上传的 maxRequestLength 限制? 2022-01-01
- 如何在 IDE 中获取 Xamarin Studio C# 输出? 2022-01-01
- 如何激活MC67中的红灯 2022-01-01
- MS Visual Studio .NET 的替代品 2022-01-01
- c# Math.Sqrt 实现 2022-01-01
- rabbitmq 的 REST API 2022-01-01
- 将 XML 转换为通用列表 2022-01-01
- 为什么Flurl.Http DownloadFileAsync/Http客户端GetAsync需要 2022-09-30
- 将 Office 安装到 Windows 容器 (servercore:ltsc2019) 失败,错误代码为 17002 2022-01-01
- SSE 浮点算术是否可重现? 2022-01-01