How to encrypt a string in .NET?(如何在 .NET 中加密字符串?)
问题描述
我必须加密/解密 Xml 文件中的一些敏感信息?是的,我可以通过编写自己的自定义算法来做到这一点.我想知道 .NET 中是否已经有内置方法可以做到这一点,以及我总是需要注意哪些方面..
I have to encrypt/decrypt some sensitive information in a Xml file? Yes I can do that by writing my own custom algorithms. I am wondering if there is already a built in way in .NET to do that and also what points I always need to take care..
推荐答案
下面是几个使用 .NET 框架加密和解密字符串的函数:
Here's a couple of functions that use the .NET framework to encrypt and decrypt a string:
public string EncryptString(string plainText)
{
// Instantiate a new RijndaelManaged object to perform string symmetric encryption
RijndaelManaged rijndaelCipher = new RijndaelManaged();
// Set key and IV
rijndaelCipher.Key = Convert.FromBase64String("ABC");
rijndaelCipher.IV = Convert.FromBase64String("123");
// Instantiate a new MemoryStream object to contain the encrypted bytes
MemoryStream memoryStream = new MemoryStream();
// Instantiate a new encryptor from our RijndaelManaged object
ICryptoTransform rijndaelEncryptor = rijndaelCipher.CreateEncryptor();
// Instantiate a new CryptoStream object to process the data and write it to the
// memory stream
CryptoStream cryptoStream = new CryptoStream(memoryStream, rijndaelEncryptor, CryptoStreamMode.Write);
// Convert the plainText string into a byte array
byte[] plainBytes = Encoding.ASCII.GetBytes(plainText);
// Encrypt the input plaintext string
cryptoStream.Write(plainBytes, 0, plainBytes.Length);
// Complete the encryption process
cryptoStream.FlushFinalBlock();
// Convert the encrypted data from a MemoryStream to a byte array
byte[] cipherBytes = memoryStream.ToArray();
// Close both the MemoryStream and the CryptoStream
memoryStream.Close();
cryptoStream.Close();
// Convert the encrypted byte array to a base64 encoded string
string cipherText = Convert.ToBase64String(cipherBytes, 0, cipherBytes.Length);
// Return the encrypted data as a string
return cipherText;
}
public string DecryptString(string cipherText)
{
// Instantiate a new RijndaelManaged object to perform string symmetric encryption
RijndaelManaged rijndaelCipher = new RijndaelManaged();
// Set key and IV
rijndaelCipher.Key = Convert.FromBase64String("ABC");
rijndaelCipher.IV = Convert.FromBase64String("123");
// Instantiate a new MemoryStream object to contain the encrypted bytes
MemoryStream memoryStream = new MemoryStream();
// Instantiate a new encryptor from our RijndaelManaged object
ICryptoTransform rijndaelDecryptor = rijndaelCipher.CreateDecryptor();
// Instantiate a new CryptoStream object to process the data and write it to the
// memory stream
CryptoStream cryptoStream = new CryptoStream(memoryStream, rijndaelDecryptor, CryptoStreamMode.Write);
// Will contain decrypted plaintext
string plainText = String.Empty;
try
{
// Convert the ciphertext string into a byte array
byte[] cipherBytes = Convert.FromBase64String(cipherText);
// Decrypt the input ciphertext string
cryptoStream.Write(cipherBytes, 0, cipherBytes.Length);
// Complete the decryption process
cryptoStream.FlushFinalBlock();
// Convert the decrypted data from a MemoryStream to a byte array
byte[] plainBytes = memoryStream.ToArray();
// Convert the encrypted byte array to a base64 encoded string
plainText = Encoding.ASCII.GetString(plainBytes, 0, plainBytes.Length);
}
finally
{
// Close both the MemoryStream and the CryptoStream
memoryStream.Close();
cryptoStream.Close();
}
// Return the encrypted data as a string
return plainText;
}
当然,我不建议像这样对密钥和初始化向量进行硬编码 :)
Of course I don't advise hardcoding the key and initialisation vector like this :)
这篇关于如何在 .NET 中加密字符串?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何在 .NET 中加密字符串?
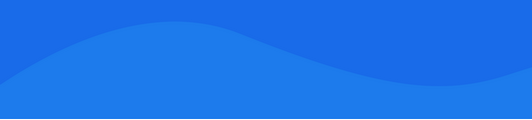
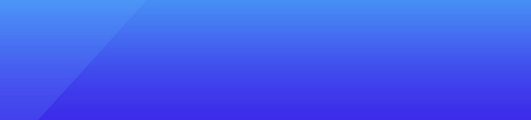
基础教程推荐
- 将 Office 安装到 Windows 容器 (servercore:ltsc2019) 失败,错误代码为 17002 2022-01-01
- c# Math.Sqrt 实现 2022-01-01
- rabbitmq 的 REST API 2022-01-01
- 如何在 IDE 中获取 Xamarin Studio C# 输出? 2022-01-01
- SSE 浮点算术是否可重现? 2022-01-01
- 将 XML 转换为通用列表 2022-01-01
- MS Visual Studio .NET 的替代品 2022-01-01
- 如何激活MC67中的红灯 2022-01-01
- 为什么Flurl.Http DownloadFileAsync/Http客户端GetAsync需要 2022-09-30
- 有没有办法忽略 2GB 文件上传的 maxRequestLength 限制? 2022-01-01