How to mock static methods in c# using MOQ framework?(如何使用 MOQ 框架在 c# 中模拟静态方法?)
问题描述
我最近一直在做单元测试,我已经使用 MOQ 框架和 MS Test 成功地模拟了各种场景.我知道我们无法测试私有方法,但我想知道我们是否可以使用 MOQ 模拟静态方法.
I have been doing unit testing recently and I've successfully mocked various scenarios using MOQ framework and MS Test. I know we can't test private methods but I want to know if we can mock static methods using MOQ.
推荐答案
起订量(和其他DynamicProxy-based 模拟框架)无法模拟任何不是虚拟或抽象方法的东西.
Moq (and other DynamicProxy-based mocking frameworks) are unable to mock anything that is not a virtual or abstract method.
只能使用基于 Profiler API 的工具来伪造密封/静态类/方法,例如 Typemock(商业)或 Microsoft Moles(免费,在 Visual Studio 2012 Ultimate 中称为 Fakes/2013/2015).
Sealed/static classes/methods can only be faked with Profiler API based tools, like Typemock (commercial) or Microsoft Moles (free, known as Fakes in Visual Studio 2012 Ultimate /2013 /2015).
或者,您可以重构您的设计以抽象调用静态方法,并通过依赖注入将此抽象提供给您的类.那么您不仅会有更好的设计,而且还可以使用免费工具(例如 Moq)进行测试.
Alternatively, you could refactor your design to abstract calls to static methods, and provide this abstraction to your class via dependency injection. Then you'd not only have a better design, it will be testable with free tools, like Moq.
无需完全使用任何工具即可应用允许可测试性的通用模式.考虑以下方法:
A common pattern to allow testability can be applied without using any tools altogether. Consider the following method:
public class MyClass
{
public string[] GetMyData(string fileName)
{
string[] data = FileUtil.ReadDataFromFile(fileName);
return data;
}
}
您可以将其包装在 protected virtual
方法中,而不是尝试模拟 FileUtil.ReadDataFromFile
,如下所示:
Instead of trying to mock FileUtil.ReadDataFromFile
, you could wrap it in a protected virtual
method, like this:
public class MyClass
{
public string[] GetMyData(string fileName)
{
string[] data = GetDataFromFile(fileName);
return data;
}
protected virtual string[] GetDataFromFile(string fileName)
{
return FileUtil.ReadDataFromFile(fileName);
}
}
然后,在您的单元测试中,从 MyClass
派生并将其命名为 TestableMyClass
.然后你可以重写 GetDataFromFile
方法来返回你自己的测试数据.
Then, in your unit test, derive from MyClass
and call it TestableMyClass
. Then you can override the GetDataFromFile
method to return your own test data.
希望对您有所帮助.
这篇关于如何使用 MOQ 框架在 c# 中模拟静态方法?的文章就介绍到这了,希望我们推荐的答案对大家有所帮助,也希望大家多多支持编程学习网!
本文标题为:如何使用 MOQ 框架在 c# 中模拟静态方法?
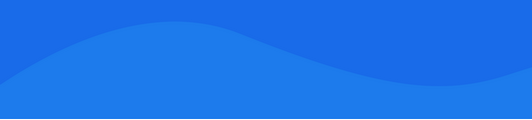
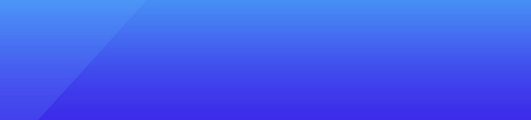
基础教程推荐
- SSE 浮点算术是否可重现? 2022-01-01
- 为什么Flurl.Http DownloadFileAsync/Http客户端GetAsync需要 2022-09-30
- 如何激活MC67中的红灯 2022-01-01
- 有没有办法忽略 2GB 文件上传的 maxRequestLength 限制? 2022-01-01
- rabbitmq 的 REST API 2022-01-01
- c# Math.Sqrt 实现 2022-01-01
- 如何在 IDE 中获取 Xamarin Studio C# 输出? 2022-01-01
- MS Visual Studio .NET 的替代品 2022-01-01
- 将 Office 安装到 Windows 容器 (servercore:ltsc2019) 失败,错误代码为 17002 2022-01-01
- 将 XML 转换为通用列表 2022-01-01